module web.WebClient
class ()
module web.KanbanRenderer
class KanbanRenderer()
method addQuickCreate()
Displays the quick create record in the active column
method quickCreateToggleFold()
Toggle fold/unfold the Column quick create widget
method removeWidget(widget)
Removes a widget (record if ungrouped, column if grouped) from the view.
- widget (
Widget
) – the instance of the widget to removemethod updateColumn(localID)
Overrides to restore the left property and the scrollTop on the updated column, and to enable the swipe handlers
- localID
method updateRecord(recordState)
Updates a given record with its new state.
- recordState (
Object
)module payment_stripe.stripe
namespace
module web.notification
namespace
module web.GanttRenderer
class (parent, state, params)
class Params()
attribute stacked boolean
module web.FilterMenu
class (parent, filters, fields)
parent
filters
fields
module web.AbstractController
class AbstractController(parent, model, renderer, params)
parent (Widget
)
model (AbstractModel
)
renderer (AbstractRenderer
)
params (AbstractControllerParams
)
method start() → Deferred
Simply renders and updates the url.
method on_attach_callback()
Called each time the controller is attached into the DOM.
method on_detach_callback()
Called each time the controller is detached from the DOM.
method discardChanges([recordID]) → Deferred
Discards the changes made on the record associated to the given ID, or all changes made by the current controller if no recordID is given. For example, when the user open the ‘home’ screen, the view manager will call this method on the active view to make sure it is ok to open the home screen (and lose all current state).
Note that it returns a deferred, because the view could choose to ask the user if he agrees to discard.
- recordID (
string
) – if not given, we consider all the changes made by the controllermethod getContext() → Object
Returns any special keys that may be useful when reloading the view to get the same effect. This is necessary for saving the current view in the favorites. For example, a graph view might want to add a key to save the current graph type.
Object
method getTitle() → string
Returns a title that may be displayed in the breadcrumb area. For example, the name of the record.
Note: this seems wrong right now, it should not be implemented, we have no guarantee that there is a display_name variable in a controller.
string
method is_action_enabled(action) → boolean
The use of this method is discouraged. It is still snakecased, because it currently is used in many templates, but we will move to a simpler mechanism as soon as we can.
- action (
string
) – type of action, such as ‘create’, ‘read’, …boolean
method reload([params]) → Deferred
Short helper method to reload the view
- params (
Object
) – This object will simply be given to the updatemethod renderButtons($node)
Most likely called by the view manager, this method is responsible for adding buttons in the control panel (buttons such as save/discard/…)
Note that there is no guarantee that this method will be called. The controller is supposed to work even without a view manager, for example in the frontend (flectra frontend = public website)
- $node (
jQuery
)method renderPager($node)
For views that require a pager, this method will be called to allow the controller to instantiate and render a pager. Note that in theory, the controller can actually render whatever he wants in the pager zone. If your view does not want a pager, just let this method empty.
- $node (
Query
)method renderSidebar($node)
Same as renderPager, but for the ‘sidebar’ zone (the zone with the menu dropdown in the control panel next to the buttons)
- $node (
Query
)method setScrollTop(scrollTop)
Not sure about this one, it probably needs to be reworked, maybe merged in get/set local state methods.
- scrollTop (
number
)method update(params[, options]) → Deferred
This is the main entry point for the controller. Changes from the search view arrive in this method, and internal changes can sometimes also call this method. It is basically the way everything notifies the controller that something has changed.
The update method is responsible for fetching necessary data, then updating the renderer and wait for the rendering to complete.
params (Object
) – will be given to the model and to the renderer
options (UpdateOptions
)
class UpdateOptions()
attribute reload boolean
if true, the model will reload data
class AbstractControllerParams()
attribute modelName string
attribute handle any
a handle that will be given to the model (some id)
attribute initialState any
the initialState
module web.widget_registry
object instance of Registry
module web.ListController
class ListController()
method getActiveDomain() → Deferred<array[]>
Calculate the active domain of the list view. This should be done only if the header checkbox has been checked. This is done by evaluating the search results, and then adding the dataset domain (i.e. action domain).
method getSelectedIds() → number[]
Returns the list of currently selected res_ids (with the check boxes on the left)
Array
<number
>method getSelectedRecords() → Object[]
Returns the list of currently selected records (with the check boxes on the left)
Array
<Object
>method renderButtons()
Extends the renderButtons function of ListView by adding an event listener on the import button.
method renderSidebar($node)
Render the sidebar (the ‘action’ menu in the control panel, right of the main buttons)
- $node (
jQuery
)method update(params, options)
Overrides to update the list of selected records
params
options
module web.collections
class Tree(data)
- data (
any
) – the data associated to the root nodeAllows to build a tree representation of a data.
method getData() → *
Returns the root’s associated data.
any
method addChild(tree)
Adds a child tree.
- tree (
Tree
)namespace
class Tree(data)
- data (
any
) – the data associated to the root nodeAllows to build a tree representation of a data.
method getData() → *
Returns the root’s associated data.
any
method addChild(tree)
Adds a child tree.
- tree (
Tree
)module web.datepicker
namespace
module mail.Chatter
class Chatter(parent, record, mailFields, options)
module web.kanban_quick_create
namespace
module web.BrowserDetection
class BrowserDetection()
module web.view_dialogs
class SelectCreateDialog()
Search dialog (displays a list of records and permits to create a new one by switching to a form view)
namespace
class FormViewDialog(parent[, options])
parent (Widget
)
options (FormViewDialogOptions
)
Create and edit dialog (displays a form view record and leave once saved)
method open() → FormViewDialog
Open the form view dialog. It is necessarily asynchronous, but this method returns immediately.
class FormViewDialogOptions()
attribute parentID string
- the id of the parent record. It is
- useful for situations such as a one2many opened in a form view dialog. In that case, we want to be able to properly evaluate domains with the ‘parent’ key.
attribute res_id integer
the id of the record to open
attribute form_view_options Object
- dict of options to pass to
- the Form View @todo: make it work
attribute fields_view Object
optional form fields_view
attribute readonly boolean
- only applicable when not in
- creation mode
attribute on_saved function
- callback executed after saving a
- record. It will be called with the record data, and a boolean which indicates if something was changed
attribute model BasicModel
- if given, it will be used instead of
- a new form view model
attribute recordID string
- if given, the model has to be given as
- well, and in that case, it will be used without loading anything.
attribute shouldSaveLocally boolean
- if true, the view dialog
- will save locally instead of actually saving (useful for one2manys)
class SelectCreateDialog()
Search dialog (displays a list of records and permits to create a new one by switching to a form view)
class ViewDialog(parent[, options])
parent (Widget
)
options (ViewDialogOptions
)
Class with everything which is common between FormViewDialog and SelectCreateDialog.
class ViewDialogOptions()
attribute dialogClass string
attribute res_model string
the model of the record(s) to open
attribute domain any[]
attribute context Object
class FormViewDialog(parent[, options])
parent (Widget
)
options (FormViewDialogOptions
)
Create and edit dialog (displays a form view record and leave once saved)
method open() → FormViewDialog
Open the form view dialog. It is necessarily asynchronous, but this method returns immediately.
class FormViewDialogOptions()
attribute parentID string
- the id of the parent record. It is
- useful for situations such as a one2many opened in a form view dialog. In that case, we want to be able to properly evaluate domains with the ‘parent’ key.
attribute res_id integer
the id of the record to open
attribute form_view_options Object
- dict of options to pass to
- the Form View @todo: make it work
attribute fields_view Object
optional form fields_view
attribute readonly boolean
- only applicable when not in
- creation mode
attribute on_saved function
- callback executed after saving a
- record. It will be called with the record data, and a boolean which indicates if something was changed
attribute model BasicModel
- if given, it will be used instead of
- a new form view model
attribute recordID string
- if given, the model has to be given as
- well, and in that case, it will be used without loading anything.
attribute shouldSaveLocally boolean
- if true, the view dialog
- will save locally instead of actually saving (useful for one2manys)
module web.time
function str_to_date(str) → Date
Converts a string to a Date javascript object using OpenERP’s date string format (exemple: ‘2011-12-01’).
As a date is not subject to time zones, we assume it should be represented as a Date javascript object at 00:00:00 in the time zone of the browser.
- str (
String
) – A string representing a date.Date
function moment_to_strftime_format(value)
Convert moment.js format to python strftime
- value (
String
) – original formatfunction getLangDatetimeFormat()
Get date time format of the user’s language
function str_to_datetime(str) → Date
Converts a string to a Date javascript object using OpenERP’s datetime string format (exemple: ‘2011-12-01 15:12:35.832’).
The time zone is assumed to be UTC (standard for OpenERP 6.1) and will be converted to the browser’s time zone.
- str (
String
) – A string representing a datetime.Date
namespace
function date_to_utc(k, v) → Object
Replacer function for JSON.stringify, serializes Date objects to UTC datetime in the OpenERP Server format.
However, if a serialized value has a toJSON method that method is called before the replacer is invoked. Date#toJSON exists, and thus the value passed to the replacer is a string, the original Date has to be fetched on the parent object (which is provided as the replacer’s context).
k (String
)
v (Object
)
Object
function str_to_datetime(str) → Date
Converts a string to a Date javascript object using OpenERP’s datetime string format (exemple: ‘2011-12-01 15:12:35.832’).
The time zone is assumed to be UTC (standard for OpenERP 6.1) and will be converted to the browser’s time zone.
- str (
String
) – A string representing a datetime.Date
function str_to_date(str) → Date
Converts a string to a Date javascript object using OpenERP’s date string format (exemple: ‘2011-12-01’).
As a date is not subject to time zones, we assume it should be represented as a Date javascript object at 00:00:00 in the time zone of the browser.
- str (
String
) – A string representing a date.Date
function str_to_time(str) → Date
Converts a string to a Date javascript object using OpenERP’s time string format (exemple: ‘15:12:35’).
The OpenERP times are supposed to always be naive times. We assume it is represented using a javascript Date with a date 1 of January 1970 and a time corresponding to the meant time in the browser’s time zone.
- str (
String
) – A string representing a time.Date
function datetime_to_str(obj) → String
Converts a Date javascript object to a string using OpenERP’s datetime string format (exemple: ‘2011-12-01 15:12:35’).
The time zone of the Date object is assumed to be the one of the browser and it will be converted to UTC (standard for OpenERP 6.1).
- obj (
Date
)String
function date_to_str(obj) → String
Converts a Date javascript object to a string using OpenERP’s date string format (exemple: ‘2011-12-01’).
As a date is not subject to time zones, we assume it should be represented as a Date javascript object at 00:00:00 in the time zone of the browser.
- obj (
Date
)String
function time_to_str(obj) → String
Converts a Date javascript object to a string using OpenERP’s time string format (exemple: ‘15:12:35’).
The OpenERP times are supposed to always be naive times. We assume it is represented using a javascript Date with a date 1 of January 1970 and a time corresponding to the meant time in the browser’s time zone.
- obj (
Date
)String
function strftime_to_moment_format(value)
Convert Python strftime to escaped moment.js format.
- value (
String
) – original formatfunction moment_to_strftime_format(value)
Convert moment.js format to python strftime
- value (
String
) – original formatfunction getLangDateFormat()
Get date format of the user’s language
function getLangTimeFormat()
Get time format of the user’s language
function getLangDatetimeFormat()
Get date time format of the user’s language
function getLangDateFormat()
Get date format of the user’s language
function date_to_str(obj) → String
Converts a Date javascript object to a string using OpenERP’s date string format (exemple: ‘2011-12-01’).
As a date is not subject to time zones, we assume it should be represented as a Date javascript object at 00:00:00 in the time zone of the browser.
- obj (
Date
)String
function getLangTimeFormat()
Get time format of the user’s language
function datetime_to_str(obj) → String
Converts a Date javascript object to a string using OpenERP’s datetime string format (exemple: ‘2011-12-01 15:12:35’).
The time zone of the Date object is assumed to be the one of the browser and it will be converted to UTC (standard for OpenERP 6.1).
- obj (
Date
)String
function strftime_to_moment_format(value)
Convert Python strftime to escaped moment.js format.
- value (
String
) – original formatfunction str_to_time(str) → Date
Converts a string to a Date javascript object using OpenERP’s time string format (exemple: ‘15:12:35’).
The OpenERP times are supposed to always be naive times. We assume it is represented using a javascript Date with a date 1 of January 1970 and a time corresponding to the meant time in the browser’s time zone.
- str (
String
) – A string representing a time.Date
function time_to_str(obj) → String
Converts a Date javascript object to a string using OpenERP’s time string format (exemple: ‘15:12:35’).
The OpenERP times are supposed to always be naive times. We assume it is represented using a javascript Date with a date 1 of January 1970 and a time corresponding to the meant time in the browser’s time zone.
- obj (
Date
)String
function date_to_utc(k, v) → Object
Replacer function for JSON.stringify, serializes Date objects to UTC datetime in the OpenERP Server format.
However, if a serialized value has a toJSON method that method is called before the replacer is invoked. Date#toJSON exists, and thus the value passed to the replacer is a string, the original Date has to be fetched on the parent object (which is provided as the replacer’s context).
k (String
)
v (Object
)
Object
module web.Menu
class Menu()
method reflow(behavior)
Reflow the menu items and dock overflowing items into a “More” menu item. Automatically called when ‘menu_bound’ event is triggered and on window resizing.
- behavior (
string
) – If set to ‘all_outside’, all the items are displayed.
If not set, only the overflowing items are hidden.method open_menu(id)
Opens a given menu by id, as if a user had browsed to that menu by hand except does not trigger any event on the way
- id (
Number
) – database id of the terminal menu to selectmethod open_action(id[, menuID])
Call open_menu on a menu_item that matches the action_id
If menuID
is a match on this action, open this menu_item.
Otherwise open the first menu_item that matches the action_id.
id (Number
) – the action_id to match
menuID (Number
) – a menu ID that may match with provided action
method menu_click(id)
Process a click on a menu item
- id (
Number
) – the menu_idmethod on_change_top_menu([menu_id])
Change the current top menu
- menu_id (
int
) – the top menu idmodule web.AbstractView
class AbstractView(viewInfo, params)
viewInfo (AbstractViewViewInfo
)
params (AbstractViewParams
)
method getController(parent) → Deferred
Main method of the view class. Create a controller, and make sure that data and libraries are loaded.
There is a unusual thing going in this method with parents: we create renderer/model with parent as parent, then we have to reassign them at the end to make sure that we have the proper relationships. This is necessary to solve the problem that the controller need the model and the renderer to be instantiated, but the model need a parent to be able to load itself, and the renderer needs the data in its constructor.
method getModel(parent) → Object
Returns the view model or create an instance of it if none
- parent (
Widget
) – the parent of the model, if it has to be createdObject
method getRenderer(parent, state) → Object
Returns the a new view renderer instance
parent (Widget
) – the parent of the model, if it has to be created
state (Object
) – the information related to the rendered view
Object
method setController(Controller)
this is useful to customize the actual class to use before calling createView.
- Controller (
Controller
)class AbstractViewParams()
attribute modelName string
The actual model name
attribute context Object
attribute count number
attribute domain string[]
attribute groupBy string[]
attribute currentId number
attribute ids number[]
attribute action.help string
class AbstractViewViewInfo()
attribute arch Object
attribute fields Object
attribute fieldsInfo Object
module website.mobile
namespace
module website_sale.utils
namespace
module web.KanbanModel
class KanbanModel()
method addRecordToGroup(groupID, resId) → Deferred<string>
Adds a record to a group in the localData, and fetch the record.
groupID (string
) – localID of the group
resId (integer
) – id of the record
Deferred
<string
>method createGroup(name, parentID) → Deferred<string>
Creates a new group from a name (performs a name_create).
name (string
)
parentID (string
) – localID of the parent of the group
Deferred
<string
>method get() → Object
Add the key tooltipData
(kanban specific) when performing a geŧ
.
Object
method getColumn(id) → Object
Same as @see get but getting the parent element whose ID is given.
- id (
string
)Object
method loadColumnRecords(groupID) → Deferred
Opens a given group and loads its <limit> first records
- groupID (
string
)method loadMore(groupID) → Deferred<string>
Load more records in a group.
- groupID (
string
) – localID of the groupDeferred
<string
>method moveRecord(recordID, groupID, parentID) → Deferred<string[]>
Moves a record from a group to another.
recordID (string
) – localID of the record
groupID (string
) – localID of the new group of the record
parentID (string
) – localID of the parent
module website_sale.website_sale
namespace
module mail.chat_client_action
class ChatAction()
class PartnerInviteDialog(parent, title, channel_id)
parent
title
channel_id
Widget : Invite People to Channel Dialog
Popup containing a ‘many2many_tags’ custom input to select multiple partners. Searches user according to the input, and triggers event when selection is validated.
module web.ChangePassword
- web.Dialog
- web.Widget
- web.core
- web.web_client
class ChangePassword()
module web_settings_dashboard
namespace
module web_editor.IframeRoot
namespace
module pad.pad
class FieldPad()
method commitChanges()
If we had to generate an url, we wait for the generation to be completed, so the current record will be associated with the correct pad url.
module web.search_filters
namespace
module web_diagram.DiagramModel
class DiagramModel()
DiagramModel
class DiagramModel()
DiagramModel
module website_crm.tour
namespace
module portal.signature_form
namespace
module web.GanttController
class ()
module mail.Followers
class Followers(parent, name, record, options)
parent
name
record
options
module website_event.website_event
class EventRegistrationForm()
module web.Dialog
class Dialog(parent[, options])
parent (Widget
)
options (DialogOptions
)
A useful class to handle dialogs. Attributes:
$footer
- A jQuery element targeting a dom part where buttons can be added. It always exists during the lifecycle of the dialog.
method willStart()
Wait for XML dependencies and instantiate the modal structure (except modal-body).
method safeConfirm(owner, message[, options]) → Dialog
Static method to open double confirmation dialog.
owner (Widget
)
message (string
)
options (SafeConfirmOptions
) – @see Dialog.init @see Dialog.confirm
class SafeConfirmOptions()
@see Dialog.init @see Dialog.confirm
attribute securityLevel string
bootstrap color
attribute securityMessage string
am sure about this”]
class DialogOptions()
attribute title string
attribute subtitle string
attribute size string
‘large’, ‘medium’ or ‘small’
attribute dialogClass string
class to add to the modal-body
attribute $content jQuery
- Element which will be the $el, replace the .modal-body and get the
- modal-body class
attribute buttons Object[]
- List of button descriptions. Note: if no buttons, a “ok” primary
- button is added to allow closing the dialog
attribute buttons[].text string
attribute buttons[].classes string
- Default to ‘btn-primary’ if only one button, ‘btn-default’
- otherwise
attribute buttons[].close boolean
attribute buttons[].click function
attribute buttons[].disabled boolean
attribute technical boolean
- If set to false, the modal will have the standard frontend style
- (use this for non-editor frontend features)
class Dialog(parent[, options])
parent (Widget
)
options (DialogOptions
)
A useful class to handle dialogs. Attributes:
$footer
- A jQuery element targeting a dom part where buttons can be added. It always exists during the lifecycle of the dialog.
method willStart()
Wait for XML dependencies and instantiate the modal structure (except modal-body).
method safeConfirm(owner, message[, options]) → Dialog
Static method to open double confirmation dialog.
owner (Widget
)
message (string
)
options (SafeConfirmOptions
) – @see Dialog.init @see Dialog.confirm
class SafeConfirmOptions()
@see Dialog.init @see Dialog.confirm
attribute securityLevel string
bootstrap color
attribute securityMessage string
am sure about this”]
class DialogOptions()
attribute title string
attribute subtitle string
attribute size string
‘large’, ‘medium’ or ‘small’
attribute dialogClass string
class to add to the modal-body
attribute $content jQuery
- Element which will be the $el, replace the .modal-body and get the
- modal-body class
attribute buttons Object[]
- List of button descriptions. Note: if no buttons, a “ok” primary
- button is added to allow closing the dialog
attribute buttons[].text string
attribute buttons[].classes string
- Default to ‘btn-primary’ if only one button, ‘btn-default’
- otherwise
attribute buttons[].close boolean
attribute buttons[].click function
attribute buttons[].disabled boolean
attribute technical boolean
- If set to false, the modal will have the standard frontend style
- (use this for non-editor frontend features)
module web.planner.common
namespace
module web.SearchView
class SearchView(parent, dataset, fvg[, options])
parent
dataset
fvg
options (SearchViewOptions
)
method do_search([_query][, options])
Performs the search view collection of widget data.
If the collection went well (all fields are valid), then triggers
instance.web.SearchView.on_search()
.
If at least one field failed its validation, triggers
instance.web.SearchView.on_invalid()
instead.
_query
options (Object
)
method build_search_data() → Object
Extract search data from the view’s facets.
Result is an object with 3 (own) properties:
- domains
- Array of domains
- contexts
- Array of contexts
- groupbys
- Array of domains, in groupby order rather than view order
Object
method setup_global_completion()
Sets up search view’s view-wide auto-completion widget
method complete_global_search(req, resp)
Provide auto-completion result for req.term (an array to resp
)
req (CompleteGlobalSearchReq
) – request to complete
resp (Function
) – response callback
class CompleteGlobalSearchReq()
request to complete
attribute term String
searched term to complete
function select_completion(e, ui)
Action to perform in case of selection: create a facet (model) and add it to the search collection
e (Object
) – selection event, preventDefault to avoid setting value on object
ui (SelectCompletionUi
) – selection information
class SelectCompletionUi()
selection information
attribute item Object
selected completion item
function renderChangedFacets(model, options)
Call the renderFacets method with the correct arguments. This is due to the fact that change events are called with two arguments (model, options) while add, reset and remove events are called with (collection, model, options) as arguments
model
options
class SearchViewOptions()
attribute hidden Boolean
hide the search view
attribute disable_custom_filters Boolean
do not load custom filters from ir.filters
module web.KanbanRecord
class KanbanRecord(parent, state, options)
parent
state
options
method update(state)
Re-renders the record with a new state
- state (
Object
)module point_of_sale.devices
namespace
module mail.Activity
class Activity()
function _readActivities(self, ids) → Deferred<Array>
Fetches activities and postprocesses them.
This standalone function performs an RPC, but to do so, it needs an instance of a widget that implements the _rpc() function.
function setDelayLabel(activities) → Array
Set the ‘label_delay’ entry in activity data according to the deadline date
- activities (
Array
) – list of activity ObjectArray
module web.relational_fields
namespace
class FieldMany2ManyBinaryMultiFiles()
Widget to upload or delete one or more files at the same time.
class FieldSelection()
The FieldSelection widget is a simple select tag with a dropdown menu to allow the selection of a range of values. It is designed to work with fields of type ‘selection’ and ‘many2one’.
class FieldReference()
The FieldReference is a combination of a select (for the model) and
a FieldMany2one for its value.
Its intern representation is similar to the many2one (a datapoint with a
name_get
as data).
Note that there is some logic to support char field because of one use in our
codebase, but this use should be removed along with this note.
class FieldReference()
The FieldReference is a combination of a select (for the model) and
a FieldMany2one for its value.
Its intern representation is similar to the many2one (a datapoint with a
name_get
as data).
Note that there is some logic to support char field because of one use in our
codebase, but this use should be removed along with this note.
class FieldMany2ManyBinaryMultiFiles()
Widget to upload or delete one or more files at the same time.
class FieldSelection()
The FieldSelection widget is a simple select tag with a dropdown menu to allow the selection of a range of values. It is designed to work with fields of type ‘selection’ and ‘many2one’.
module web.FieldManagerMixin
namespace FieldManagerMixin
module web.search_inputs
function facet_from(field, pair)
Utility function for m2o & selection fields taking a selection/name_get pair (value, name) and converting it to a Facet descriptor
field (instance.web.search.Field
) – holder field
pair (Array
) – pair value to convert
namespace
class CharField()
Implementation of the char
OpenERP field type:
- Default operator is
ilike
rather than=
- The Javascript and the HTML values are identical (strings)
module web.data
function serialize_sort(criterion) → String
Serializes the sort criterion array of a dataset into a form which can be consumed by OpenERP’s RPC APIs.
- criterion (
Array
) – array of fields, from first to last criteria, prefixed with ‘-‘ for reverse sortingString
function deserialize_sort(criterion)
Reverse of the serialize_sort function: convert an array of SQL-like sort descriptors into a list of fields prefixed with ‘-‘ if necessary.
- criterion
namespace data
module website_slides.upload
namespace
module web_editor.transcoder
namespace
function fontToImg($editable)
Converts font icons to images.
- $editable (
jQuery
) – the element in which the font icons have to be
converted to imagesfunction imgToFont($editable)
Converts images which were the result of a font icon convertion to a font icon again.
- $editable (
jQuery
) – the element in which the images will be converted
back to font iconsfunction classToStyle($editable)
Converts css style to inline style (leave the classes on elements but forces the style they give as inline style).
- $editable (
jQuery
)function styleToClass($editable)
Removes the inline style which is not necessary (because, for example, a class on an element will induce the same style).
- $editable (
jQuery
)function attachmentThumbnailToLinkImg($editable)
Converts css display for attachment link to real image. Without this post process, the display depends on the css and the picture does not appear when we use the html without css (to send by email for e.g.)
- $editable (
jQuery
)function linkImgToAttachmentThumbnail($editable)
Revert attachmentThumbnailToLinkImg changes
- $editable (
jQuery
)function fontToImg($editable)
Converts font icons to images.
- $editable (
jQuery
) – the element in which the font icons have to be
converted to imagesfunction linkImgToAttachmentThumbnail($editable)
Revert attachmentThumbnailToLinkImg changes
- $editable (
jQuery
)function styleToClass($editable)
Removes the inline style which is not necessary (because, for example, a class on an element will induce the same style).
- $editable (
jQuery
)function classToStyle($editable)
Converts css style to inline style (leave the classes on elements but forces the style they give as inline style).
- $editable (
jQuery
)function imgToFont($editable)
Converts images which were the result of a font icon convertion to a font icon again.
- $editable (
jQuery
) – the element in which the images will be converted
back to font iconsfunction getMatchedCSSRules(a) → Object
Returns the css rules which applies on an element, tweaked so that they are browser/mail client ok.
- a (
DOMElement
)Object
function attachmentThumbnailToLinkImg($editable)
Converts css display for attachment link to real image. Without this post process, the display depends on the css and the picture does not appear when we use the html without css (to send by email for e.g.)
- $editable (
jQuery
)module web.config
namespace config
attribute debug Boolean
debug is a boolean flag. It is only considered true if the flag is set in the url
function _getSizeClass() → integer
Return the current size class
integer
function _updateSizeProps()
Update the size dependant properties in the config object. This method should be called every time the size class changes.
module web.Session
class Session(parent, origin, options)
parent – The parent of the newly created object. or `null` if the server to contact is the origin server.
origin
options (Dict
) – A dictionary that can contain the following options:
“override_session”: Default to false. If true, the current session object will
not try to re-use a previously created session id stored in a cookie.
“session_id”: Default to null. If specified, the specified session_id will be used
by this session object. Specifying this option automatically implies that the option
“override_session” is set to true.
method session_bind(origin)
Setup a session
- origin
method session_init()
Init a session, reloads from cookie, if it exists
method session_authenticate()
The session is validated by restoration of a previous session
method load_modules()
Load additional web addons of that instance and init them
method session_reload() → $.Deferred
(re)loads the content of a session: db name, username, user id, session context and status of the support contract
jQuery.Deferred
method rpc(url, params, options) → jQuery.Deferred
Executes an RPC call, registering the provided callbacks.
Registers a default error callback if none is provided, and handles setting the correct session id and session context in the parameter objects
url (String
) – RPC endpoint
params (Object
) – call parameters
options (Object
) – additional options for rpc call
jQuery.Deferred
method getTZOffset(date) → integer
Returns the time zone difference (in minutes) from the current locale (host system settings) to UTC, for a given date. The offset is positive if the local timezone is behind UTC, and negative if it is ahead.
- date (
string
or moment
) – a valid string date or moment instanceinteger
module web.rainbow_man
class RainbowMan([options])
- options (
RainbowManOptions
) – key-value options to decide rainbowman behavior / appearanceclass RainbowManOptions()
key-value options to decide rainbowman behavior / appearance
attribute message string
Message to be displayed on rainbowman card
attribute fadeout string
- Delay for rainbowman to disappear - [options.fadeout=’fast’] will make rainbowman
- dissapear quickly, [options.fadeout=’medium’] and [options.fadeout=’slow’] will wait little longer before disappearing (can be used when [options.message] is longer), [options.fadeout=’no’] will keep rainbowman on screen until user clicks anywhere outside rainbowman
attribute img_url string
URL of the image to be displayed
attribute click_close Boolean
If true, destroys rainbowman on click outside
module website_links.charts
namespace exports
module web_editor.BodyManager
class BodyManager()
- ServiceProvider
Element which is designed to be unique and that will be the top-most element in the widget hierarchy. So, all other widgets will be indirectly linked to this Class instance. Its main role will be to retrieve RPC demands from its children and handle them.
class BodyManager()
- ServiceProvider
Element which is designed to be unique and that will be the top-most element in the widget hierarchy. So, all other widgets will be indirectly linked to this Class instance. Its main role will be to retrieve RPC demands from its children and handle them.
module web.field_registry
object instance of Registry
module barcodes.BarcodeEvents
namespace
object BarcodeEvents instance of BarcodeEvents
Singleton that emits barcode_scanned events on core.bus
attribute ReservedBarcodePrefixes Array
List of barcode prefixes that are reserved for internal purposes
module web.concurrency
namespace
function asyncWhen() → Deferred
The jquery implementation for $.when has a (most of the time) useful property: it is synchronous, if the deferred is resolved immediately.
This means that when we execute $.when(def), then all registered callbacks will be executed before the next line is executed. This is useful quite often, but in some rare cases, we might want to force an async behavior. This is the purpose of this function, which simply adds a setTimeout before resolving the deferred.
function delay([wait]) → Deferred
Returns a deferred resolved after ‘wait’ milliseconds
- wait=0 (
int
) – the delay in msclass DropMisordered([failMisordered])
- failMisordered=false (
boolean
) – whether mis-ordered responses
should be failed or just ignoredThe DropMisordered abstraction is useful for situations where you have a sequence of operations that you want to do, but if one of them completes after a subsequent operation, then its result is obsolete and should be ignored.
Note that is is kind of similar to the DropPrevious abstraction, but subtly different. The DropMisordered operations will all resolves if they complete in the correct order.
method add(deferred) → Deferred
Adds a deferred (usually an async request) to the sequencer
class DropPrevious()
The DropPrevious abstraction is useful when you have a sequence of operations that you want to execute, but you only care of the result of the last operation.
For example, let us say that we have a _fetch method on a widget which fetches data. We want to rerender the widget after. We could do this:
this._fetch().then(function (result) {
self.state = result;
self.render();
});
Now, we have at least two problems:
- if this code is called twice and the second _fetch completes before the first, the end state will be the result of the first _fetch, which is not what we expect
- in any cases, the user interface will rerender twice, which is bad.
Now, if we have a DropPrevious:
this.dropPrevious = new DropPrevious();
Then we can wrap the _fetch in a DropPrevious and have the expected result:
this.dropPrevious
.add(this._fetch())
.then(function (result) {
self.state = result;
self.render();
});
method add(deferred) → Promise
Registers a new deferred and rejects the previous one
- deferred (
Deferred
) – the new deferredPromise
class Mutex()
A (Flectra) mutex is a primitive for serializing computations. This is useful to avoid a situation where two computations modify some shared state and cause some corrupted state.
Imagine that we have a function to fetch some data _load(), which returns a deferred which resolves to something useful. Now, we have some code looking like this:
return this._load().then(function (result) {
this.state = result;
});
If this code is run twice, but the second execution ends before the first, then the final state will be the result of the first call to _load. However, if we have a mutex:
this.mutex = new Mutex();
and if we wrap the calls to _load in a mutex:
return this.mutex.exec(function() {
return this._load().then(function (result) {
this.state = result;
});
});
Then, it is guaranteed that the final state will be the result of the second execution.
A Mutex has to be a class, and not a function, because we have to keep track of some internal state.
method exec(action) → Deferred
Add a computation to the queue, it will be executed as soon as the previous computations are completed.
- action (
function
) – a function which may return a deferredfunction rejectAfter([target_def][, reference_def]) → Deferred
Rejects a deferred as soon as a reference deferred is either resolved or rejected
module web.UserMenu
class UserMenu()
module website_hr_recruitment.tour
namespace
module web_editor.root_widget
class RootWidget()
Specialized Widget which automatically instantiates child widgets to attach to internal DOM elements once it is started. The widgets to instantiate are known thanks to a linked registry which contains info about the widget classes and jQuery selectors to use to find the elements to attach them to.
namespace
class RootWidget()
Specialized Widget which automatically instantiates child widgets to attach to internal DOM elements once it is started. The widgets to instantiate are known thanks to a linked registry which contains info about the widget classes and jQuery selectors to use to find the elements to attach them to.
module website.theme
class ThemeCustomizeDialog()
module web.CalendarView
class CalendarView(viewInfo, params)
viewInfo
params
module mail.ChatWindow
class (parent, channel_id, title, is_folded, unread_msgs, options)
parent
channel_id
title
is_folded
unread_msgs
options
module web.FavoriteMenu
class (parent, query, target_model, action_id, filters)
parent
query
target_model
action_id
filters
function start()
We manually add the ‘add to dashboard’ feature in the searchview.
function key_for(filter) → String
Generates a mapping key (in the filters and $filter mappings) for the
filter descriptor object provided (as returned by get_filters
).
The mapping key is guaranteed to be unique for a given (user_id, name) pair.
class KeyForFilter()
attribute name String
attribute user_id Number|Pair<Number, String>
function facet_for(filter) → Object
Generates a Facet()
descriptor from a
filter descriptor
- filter (
FacetForFilter
)Object
class FacetForFilter()
attribute name String
attribute context Object
attribute domain Array
function add_filter([filter])
Adds a filter description to the filters dict
- filter (
Object
) – the filter descriptionfunction append_filter([filter])
Creates a $filter JQuery node, adds it to the $filters dict and appends it to the filter menu
- filter (
Object
) – the filter descriptionmodule web.GanttView
class GanttView(viewInfo)
- viewInfo
module web.Widget
class Widget(parent)
- PropertiesMixin
- ServicesMixin
- parent (
openerp.Widget
) – Binds the current instance to the given Widget instance.
When that widget is destroyed by calling destroy(), the current instance will be
destroyed too. Can be null.Base class for all visual components. Provides a lot of functions helpful for the management of a part of the DOM.
Widget handles:
- Rendering with QWeb.
- Life-cycle management and parenting (when a parent is destroyed, all its children are destroyed too).
- Insertion in DOM.
Guide to create implementations of the Widget class
Here is a sample child class:
var MyWidget = openerp.base.Widget.extend({
// the name of the QWeb template to use for rendering
template: "MyQWebTemplate",
init: function(parent) {
this._super(parent);
// stuff that you want to init before the rendering
},
start: function() {
// stuff you want to make after the rendering, `this.$el` holds a correct value
this.$(".my_button").click(/* an example of event binding * /);
// if you have some asynchronous operations, it's a good idea to return
// a promise in start()
var promise = this._rpc(...);
return promise;
}
});
Now this class can simply be used with the following syntax:
var my_widget = new MyWidget(this);
my_widget.appendTo($(".some-div"));
With these two lines, the MyWidget instance was initialized, rendered,
inserted into the DOM inside the .some-div
div and its events were
bound.
And of course, when you don’t need that widget anymore, just do:
my_widget.destroy();
That will kill the widget in a clean way and erase its content from the dom.
attribute template String
The name of the QWeb template that will be used for rendering. Must be redefined in subclasses or the default render() method can not be used.
attribute xmlDependencies string[]
List of paths to xml files that need to be loaded before the widget can be rendered. This will not induce loading anything that has already been loaded.
method willStart() → Deferred
Method called between @see init and @see start. Performs asynchronous calls required by the rendering and the start method.
This method should return a Deferred which is resolved when start can be executed.
method destroy()
Destroys the current widget, also destroys all its children before destroying itself.
method appendTo(target)
Renders the current widget and appends it to the given jQuery object or Widget.
- target – A jQuery object or a Widget instance.
method prependTo(target)
Renders the current widget and prepends it to the given jQuery object or Widget.
- target – A jQuery object or a Widget instance.
method insertAfter(target)
Renders the current widget and inserts it after to the given jQuery object or Widget.
- target – A jQuery object or a Widget instance.
method insertBefore(target)
Renders the current widget and inserts it before to the given jQuery object or Widget.
- target – A jQuery object or a Widget instance.
method attachTo(target)
Attach the current widget to a dom element
- target – A jQuery object or a Widget instance.
method replace(target)
Renders the current widget and replaces the given jQuery object.
- target – A jQuery object or a Widget instance.
method start() → jQuery.Deferred or any
Method called after rendering. Mostly used to bind actions, perform asynchronous calls, etc…
By convention, this method should return an object that can be passed to $.when() to inform the caller when this widget has been initialized.
jQuery.Deferred
or any
method renderElement()
Renders the element. The default implementation renders the widget using QWeb,
this.template
must be defined. The context given to QWeb contains the “widget”
key that references this
.
method replaceElement($el) → Widget
Re-sets the widget’s root element and replaces the old root element (if any) by the new one in the DOM.
method setElement(element) → Widget
Re-sets the widget’s root element (el/$el/$el).
Includes:
- re-delegating events
- re-binding sub-elements
- if the widget already had a root element, replacing the pre-existing element in the DOM
- element (
HTMLElement
or jQuery
) – new root element for the widgetmethod make(tagName[, attributes][, content]) → Element
Utility function to build small DOM elements.
tagName (String
) – name of the DOM element to create
attributes (Object
) – map of DOM attributes to set on the element
content (String
) – HTML content to set on the element
Element
method $(selector) → jQuery
Shortcut for this.$el.find(selector)
- selector (
String
) – CSS selector, rooted in $eljQuery
method do_show()
Displays the widget
method do_hide()
Hides the widget
method do_toggle([display])
Displays or hides the widget
- display (
Boolean
) – use true to show the widget or false to hide itclass Widget(parent)
- PropertiesMixin
- ServicesMixin
- parent (
openerp.Widget
) – Binds the current instance to the given Widget instance.
When that widget is destroyed by calling destroy(), the current instance will be
destroyed too. Can be null.Base class for all visual components. Provides a lot of functions helpful for the management of a part of the DOM.
Widget handles:
- Rendering with QWeb.
- Life-cycle management and parenting (when a parent is destroyed, all its children are destroyed too).
- Insertion in DOM.
Guide to create implementations of the Widget class
Here is a sample child class:
var MyWidget = openerp.base.Widget.extend({
// the name of the QWeb template to use for rendering
template: "MyQWebTemplate",
init: function(parent) {
this._super(parent);
// stuff that you want to init before the rendering
},
start: function() {
// stuff you want to make after the rendering, `this.$el` holds a correct value
this.$(".my_button").click(/* an example of event binding * /);
// if you have some asynchronous operations, it's a good idea to return
// a promise in start()
var promise = this._rpc(...);
return promise;
}
});
Now this class can simply be used with the following syntax:
var my_widget = new MyWidget(this);
my_widget.appendTo($(".some-div"));
With these two lines, the MyWidget instance was initialized, rendered,
inserted into the DOM inside the .some-div
div and its events were
bound.
And of course, when you don’t need that widget anymore, just do:
my_widget.destroy();
That will kill the widget in a clean way and erase its content from the dom.
attribute template String
The name of the QWeb template that will be used for rendering. Must be redefined in subclasses or the default render() method can not be used.
attribute xmlDependencies string[]
List of paths to xml files that need to be loaded before the widget can be rendered. This will not induce loading anything that has already been loaded.
method willStart() → Deferred
Method called between @see init and @see start. Performs asynchronous calls required by the rendering and the start method.
This method should return a Deferred which is resolved when start can be executed.
method destroy()
Destroys the current widget, also destroys all its children before destroying itself.
method appendTo(target)
Renders the current widget and appends it to the given jQuery object or Widget.
- target – A jQuery object or a Widget instance.
method prependTo(target)
Renders the current widget and prepends it to the given jQuery object or Widget.
- target – A jQuery object or a Widget instance.
method insertAfter(target)
Renders the current widget and inserts it after to the given jQuery object or Widget.
- target – A jQuery object or a Widget instance.
method insertBefore(target)
Renders the current widget and inserts it before to the given jQuery object or Widget.
- target – A jQuery object or a Widget instance.
method attachTo(target)
Attach the current widget to a dom element
- target – A jQuery object or a Widget instance.
method replace(target)
Renders the current widget and replaces the given jQuery object.
- target – A jQuery object or a Widget instance.
method start() → jQuery.Deferred or any
Method called after rendering. Mostly used to bind actions, perform asynchronous calls, etc…
By convention, this method should return an object that can be passed to $.when() to inform the caller when this widget has been initialized.
jQuery.Deferred
or any
method renderElement()
Renders the element. The default implementation renders the widget using QWeb,
this.template
must be defined. The context given to QWeb contains the “widget”
key that references this
.
method replaceElement($el) → Widget
Re-sets the widget’s root element and replaces the old root element (if any) by the new one in the DOM.
method setElement(element) → Widget
Re-sets the widget’s root element (el/$el/$el).
Includes:
- re-delegating events
- re-binding sub-elements
- if the widget already had a root element, replacing the pre-existing element in the DOM
- element (
HTMLElement
or jQuery
) – new root element for the widgetmethod make(tagName[, attributes][, content]) → Element
Utility function to build small DOM elements.
tagName (String
) – name of the DOM element to create
attributes (Object
) – map of DOM attributes to set on the element
content (String
) – HTML content to set on the element
Element
method $(selector) → jQuery
Shortcut for this.$el.find(selector)
- selector (
String
) – CSS selector, rooted in $eljQuery
method do_show()
Displays the widget
method do_hide()
Hides the widget
method do_toggle([display])
Displays or hides the widget
- display (
Boolean
) – use true to show the widget or false to hide itmodule web.Pager
class Pager([parent, ][size, ][current_min, ][limit, ]options)
parent (Widget
) – the parent widget
size (int
) – the total number of elements
current_min (int
) – the first element of the current_page
limit (int
) – the number of elements per page
options (PagerOptions
)
method start() → jQuery.Deferred
Renders the pager
jQuery.Deferred
method disable()
Disables the pager’s arrows and the edition
method enable()
Enables the pager’s arrows and the edition
method next()
Executes the next action on the pager
method previous()
Executes the previous action on the pager
method updateState([state][, options])
Sets the state of the pager and renders it
state (Object
) – the values to update (size, current_min and limit)
options (UpdateStateOptions
)
class UpdateStateOptions()
attribute notifyChange boolean
- set to true to make the pager
- notify the environment that its state changed
class PagerOptions()
attribute can_edit boolean
editable feature of the pager
attribute single_page_hidden boolean
- (not) to display the pager
- if only one page
attribute validate function
- callback returning a Deferred to
- validate changes
module web.ListView
class ListView()
module web.view_registry
object instance of Registry
module sms.sms_widget
class SmsWidget()
SmsWidget is a widget to display a textarea (the body) and a text representing the number of SMS and the number of characters. This text is computed every time the user changes the body.
class SmsWidget()
SmsWidget is a widget to display a textarea (the body) and a text representing the number of SMS and the number of characters. This text is computed every time the user changes the body.
module survey.survey
namespace
module web.mixins
class Events()
Backbone’s events. Do not ever use it directly, use EventDispatcherMixin instead.
This class just handle the dispatching of events, it is not meant to be extended, nor used directly. All integration with parenting and automatic unregistration of events is done in EventDispatcherMixin.
Copyright notice for the following Class:
(c) 2010-2012 Jeremy Ashkenas, DocumentCloud Inc. Backbone may be freely distributed under the MIT license. For all details and documentation: http://backbonejs.org
mixin EventDispatcherMixin
Mixin containing an event system. Events are also registered by specifying the target object (the object which will receive the event when it is raised). Both the event-emitting object and the target object store or reference to each other. This is used to correctly remove all reference to the event handler when any of the object is destroyed (when the destroy() method from ParentedMixin is called). Removing those references is necessary to avoid memory leak and phantom events (events which are raised and sent to a previously destroyed object).
namespace
mixin ParentedMixin
Mixin to structure objects’ life-cycles folowing a parent-children relationship. Each object can a have a parent and multiple children. When an object is destroyed, all its children are destroyed too releasing any resource they could have reserved before.
function setParent(parent)
Set the parent of the current object. When calling this method, the parent will also be informed and will return the current object when its getChildren() method is called. If the current object did already have a parent, it is unregistered before, which means the previous parent will not return the current object anymore when its getChildren() method is called.
- parent
function getParent()
Return the current parent of the object (or null).
function getChildren()
Return a list of the children of the current object.
function isDestroyed()
Returns true if destroy() was called on the current object.
function alive(promise[, reject]) → $.Deferred
Utility method to only execute asynchronous actions if the current object has not been destroyed.
promise (jQuery.Deferred
) – The promise representing the asynchronous
action.
reject=false (bool
) – If true, the returned promise will be
rejected with no arguments if the current
object is destroyed. If false, the
returned promise will never be resolved
or rejected.
jQuery.Deferred
function destroy()
Inform the object it should destroy itself, releasing any resource it could have reserved.
function findAncestor(predicate)
Find the closest ancestor matching predicate
- predicate
mixin EventDispatcherMixin
Mixin containing an event system. Events are also registered by specifying the target object (the object which will receive the event when it is raised). Both the event-emitting object and the target object store or reference to each other. This is used to correctly remove all reference to the event handler when any of the object is destroyed (when the destroy() method from ParentedMixin is called). Removing those references is necessary to avoid memory leak and phantom events (events which are raised and sent to a previously destroyed object).
mixin ParentedMixin
Mixin to structure objects’ life-cycles folowing a parent-children relationship. Each object can a have a parent and multiple children. When an object is destroyed, all its children are destroyed too releasing any resource they could have reserved before.
function setParent(parent)
Set the parent of the current object. When calling this method, the parent will also be informed and will return the current object when its getChildren() method is called. If the current object did already have a parent, it is unregistered before, which means the previous parent will not return the current object anymore when its getChildren() method is called.
- parent
function getParent()
Return the current parent of the object (or null).
function getChildren()
Return a list of the children of the current object.
function isDestroyed()
Returns true if destroy() was called on the current object.
function alive(promise[, reject]) → $.Deferred
Utility method to only execute asynchronous actions if the current object has not been destroyed.
promise (jQuery.Deferred
) – The promise representing the asynchronous
action.
reject=false (bool
) – If true, the returned promise will be
rejected with no arguments if the current
object is destroyed. If false, the
returned promise will never be resolved
or rejected.
jQuery.Deferred
function destroy()
Inform the object it should destroy itself, releasing any resource it could have reserved.
function findAncestor(predicate)
Find the closest ancestor matching predicate
- predicate
module web.DataManager
class ()
function invalidate()
Invalidates the whole cache Suggestion: could be refined to invalidate some part of the cache
function load_action([action_id][, additional_context]) → Deferred
Loads an action from its id or xmlid.
action_id (int
or string
) – the action id or xmlid
additional_context (Object
) – used to load the action
function load_views(params[, options]) → Deferred
Loads various information concerning views: fields_view for each view, the fields of the corresponding model, and optionally the filters.
params (LoadViewsParams
)
options (Object
) – dictionary of various options:
- options.load_filters: whether or not to load the filters,
- options.action_id: the action_id (required to load filters),
- options.toolbar: whether or not a toolbar will be displayed,
class LoadViewsParams()
attribute model String
attribute context Object
attribute views_descr Array
array of [view_id, view_type]
function load_filters([dataset][, action_id]) → Deferred
Loads the filters of a given model and optional action id.
dataset (Object
) – the dataset for which the filters are loaded
action_id (int
) – the id of the action (optional)
function create_filter([filter]) → Deferred
Calls ‘create_or_replace’ on ‘ir_filters’.
- filter (
Object
) – the filter descriptionfunction delete_filter([filter]) → Deferred
Calls ‘unlink’ on ‘ir_filters’.
- filter (
Object
) – the description of the filter to removefunction processViews(fieldsViews, fields)
Processes fields and fields_views. For each field, writes its name inside the field description to make it self-contained. For each fields_view, completes its fields with the missing ones.
fieldsViews (Object
) – object of fields_views (keys are view types)
fields (Object
) – all the fields of the model
module web.GroupByMenu
class (parent, groups, fields)
parent
groups
fields
module account.dashboard_setup_bar
namespace
module web.UserProfile
class UserProfile()
module point_of_sale.gui
namespace
module web.AbstractModel
class AbstractModel(parent)
method get() → *
This method should return the complete state necessary for the renderer to display the currently viewed data.
any
method load(params) → Deferred
The load method is called once in a model, when we load the data for the first time. The method returns (a deferred that resolves to) some kind of token/handle. The handle can then be used with the get method to access a representation of the data.
class LoadParams()
attribute modelName string
the name of the model
function reload(params) → Deferred
When something changes, the data may need to be refetched. This is the job for this method: reloading (only if necessary) all the data and making sure that they are ready to be redisplayed.
- params (
Object
)module website_forum.website_forum
namespace
module point_of_sale.popups
class SelectionPopupWidget()
A popup that allows the user to select one item from a list.
Example:
show_popup('selection',{
title: "Popup Title",
list: [
{ label: 'foobar', item: 45 },
{ label: 'bar foo', item: 'stuff' },
],
confirm: function(item) {
// get the item selected by the user.
},
cancel: function(){
// user chose nothing
}
});
class PopupWidget(parent, args)
parent
args
module web.DomainSelector
class DomainSelector()
The DomainSelector widget can be used to build prefix char domain. It is the DomainTree specialization to use to have a fully working widget.
Known limitations:
- Some operators like “child_of”, “parent_of”, “like”, “not like”, “=like”, “=ilike” will come only if you use them from demo data or debug input.
- Some kind of domain can not be build right now e.g (“country_id”, “in”, [1,2,3]) but you can insert from debug input.
method setDomain(domain) → Deferred
Changes the internal domain value and forces a reparsing and rerendering. If the internal domain value was already equal to the given one, this does nothing.
function instantiateNode(parent, model, domain, options) → DomainTree|DomainLeaf|null
Instantiates a DomainTree if the given domain contains several parts and a DomainLeaf if it only contains one part. Returns null otherwise.
parent (Object
)
model (string
) – the model name
domain (Array
or string
) – the prefix representation of the domain
options (Object
) – @see DomainNode.init.options
DomainTree
or DomainLeaf
or nullclass DomainNode(parent, model, domain[, options])
parent (Object
)
model (string
) – the model name
domain (Array
or string
) – the prefix representation of the domain
options (DomainNodeOptions
) – an object with possible values:
Abstraction for widgets which can represent and allow edition of a domain.
method isValid() → boolean
Should return if the node is representing a well-formed domain, whose field chains properly belong to the associated model.
boolean
method getDomain() → Array
Should return the prefix domain the widget is currently representing (an array).
Array
class DomainNodeOptions()
an object with possible values:
attribute readonly boolean
true if is readonly
attribute operators string[]
a list of available operators (null = all of supported ones)
attribute debugMode boolean
true if should be in debug
class DomainLeaf(parent, model, domain, options)
parent
model
domain
options
DomainNode which handles a domain which cannot be split in another subdomains, i.e. composed of a field chain, an operator and a value.
method willStart() → Deferred
Prepares the information the rendering of the widget will need by pre-instantiating its internal field selector widget.
class DomainTree(parent, model, domain)
parent
model
domain
DomainNode which can handle subdomains (a domain which is composed of multiple parts). It thus will be composed of other DomainTree instances and/or leaf parts of a domain (@see DomainLeaf).
class DomainSelector()
The DomainSelector widget can be used to build prefix char domain. It is the DomainTree specialization to use to have a fully working widget.
Known limitations:
- Some operators like “child_of”, “parent_of”, “like”, “not like”, “=like”, “=ilike” will come only if you use them from demo data or debug input.
- Some kind of domain can not be build right now e.g (“country_id”, “in”, [1,2,3]) but you can insert from debug input.
method setDomain(domain) → Deferred
Changes the internal domain value and forces a reparsing and rerendering. If the internal domain value was already equal to the given one, this does nothing.
module website.backend.dashboard
class Dashboard(parent, context)
method fetch_data(website_id)
Fetches dashboard data
- website_id
module web.StandaloneFieldManagerMixin
mixin StandaloneFieldManagerMixin
The StandaloneFieldManagerMixin is a mixin, designed to be used by a widget that instanciates its own field widgets.
mixin StandaloneFieldManagerMixin
The StandaloneFieldManagerMixin is a mixin, designed to be used by a widget that instanciates its own field widgets.
module web.viewUtils
namespace utils
FIXME: move this module to its own file in master
function isQuickCreateEnabled(list) → Boolean
States whether or not the quick create feature is available for the given datapoint, depending on its groupBy field.
- list (
Object
) – dataPoint of type listBoolean
namespace utils
FIXME: move this module to its own file in master
function isQuickCreateEnabled(list) → Boolean
States whether or not the quick create feature is available for the given datapoint, depending on its groupBy field.
- list (
Object
) – dataPoint of type listBoolean
module web.IFrameWidget
class IFrameWidget(parent, url)
Generic widget to create an iframe that listens for clicks
It should be extended by overwriting the methods:
init: function(parent) {
this._super(parent, <url_of_iframe>);
},
_onIFrameClicked: function(e){
filter the clicks you want to use and apply
an action on it
}
class IFrameWidget(parent, url)
Generic widget to create an iframe that listens for clicks
It should be extended by overwriting the methods:
init: function(parent) {
this._super(parent, <url_of_iframe>);
},
_onIFrameClicked: function(e){
filter the clicks you want to use and apply
an action on it
}
module web_diagram.DiagramView
class DiagramView(viewInfo, params)
viewInfo (Object
)
params (Object
)
Diagram View
method getController()
This override is quite tricky: the graph renderer uses Raphael.js to render itself, so it needs it to be loaded in the window before rendering However, the raphael.js library is built in such a way that if it detects that a module system is present, it will try to use it. So, in that case, it is not available on window.Raphael. This means that the diagram view is then broken.
As a workaround, we simply remove and restore the define function, if present, while we are loading Raphael.
class DiagramView(viewInfo, params)
viewInfo (Object
)
params (Object
)
Diagram View
method getController()
This override is quite tricky: the graph renderer uses Raphael.js to render itself, so it needs it to be loaded in the window before rendering However, the raphael.js library is built in such a way that if it detects that a module system is present, it will try to use it. So, in that case, it is not available on window.Raphael. This means that the diagram view is then broken.
As a workaround, we simply remove and restore the define function, if present, while we are loading Raphael.
module web.BasicRenderer
class BasicRenderer(parent, state, params)
parent
state
params
method canBeSaved(recordID) → string[]
This method has two responsabilities: find every invalid fields in the current view, and making sure that they are displayed as invalid, by toggling the o_form_invalid css class. It has to be done both on the widget, and on the label, if any.
- recordID (
string
)Array
<string
>method commitChanges(recordID) → Deferred
Calls ‘commitChanges’ on all field widgets, so that they can notify the environment with their current value (useful for widgets that can’t detect when their value changes or that have to validate their changes before notifying them).
- recordID (
string
)method confirmChange(state, id, fields, ev) → Deferred<AbstractField[]>
Updates the internal state of the renderer to the new state. By default, this also implements the recomputation of the modifiers and their application to the DOM and the reset of the field widgets if needed.
In case the given record is not found anymore, a whole re-rendering is completed (possible if a change in a record caused an onchange which erased the current record).
We could always rerender the view from scratch, but then it would not be as efficient, and we might lose some local state, such as the input focus cursor, or the scrolling position.
state (Object
)
id (string
)
fields (Array
<string
>)
ev (FlectraEvent
)
method focusField(id, fieldName, offset)
Activates the widget and move the cursor to the given offset
id (string
)
fieldName (string
)
offset (integer
)
module website.seo
namespace
module web.UserLogout
namespace
module web_editor.context
namespace
module report.client_action
class ReportAction(parent, action, options)
method on_message_received(ev)
Event handler of the message post. We only handle them if they’re from
web.base.url
host and protocol and if they’re part of AUTHORIZED_MESSAGES
.
- ev
module point_of_sale.chrome
namespace
module web.LocalStorageService
class LocalStorageService()
module l10n_in_gst.gstr1
namespace
module point_of_sale.DB
class PosDB(options)
- options
module web.ListRenderer
class ListRenderer(parent, state, params)
parent
state
params (ListRendererParams
)
method canBeSaved([recordID]) → string[]
If the given recordID is the list main one (or that no recordID is given), then the whole view can be saved if one of the two following conditions is true: - There is no line in edition (all lines are saved so they are all valid) - The line in edition can be saved
- recordID (
string
)Array
<string
>method confirmChange(state, id)
We need to override the confirmChange method from BasicRenderer to reevaluate the row decorations. Since they depends on the current value of the row, they might have changed between each edit.
state
id
method confirmUpdate(state, id, fields, ev) → Deferred<AbstractField[]>
This is a specialized version of confirmChange, meant to be called when the change may have affected more than one line (so, for example, an onchange which add/remove a few lines in a x2many. This does not occur in a normal list view)
The update is more difficult when other rows could have been changed. We need to potentially remove some lines, add some other lines, update some other lines and maybe reorder a few of them. This problem would neatly be solved by using a virtual dom, but we do not have this luxury yet. So, in the meantime, what we do is basically remove every current row except the ‘main’ one (the row which caused the update), then rerender every new row and add them before/after the main one.
state (Object
)
id (string
)
fields (Array
<string
>)
ev (FlectraEvent
)
method editRecord(recordID)
Edit a given record in the list
- recordID (
string
)method getEditableRecordID() → string|null
Returns the recordID associated to the line which is currently in edition or null if there is no line in edition.
string
or nullmethod removeLine(state, recordID)
Removes the line associated to the given recordID (the index of the row is found thanks to the old state), then updates the state.
state (Object
)
recordID (string
)
method setRowMode(recordID, mode) → Deferred
Updates the already rendered row associated to the given recordID so that it fits the given mode.
recordID (string
)
mode (string
)
method unselectRow() → Deferred
This method is called whenever we click/move outside of a row that was in edit mode. This is the moment we save all accumulated changes on that row, if needed (@see BasicController.saveRecord).
Note that we have to disable the focusable elements (inputs, …) to prevent subsequent editions. These edits would be lost, because the list view only saves records when unselecting a row.
class ListRendererParams()
attribute addCreateLine boolean
attribute addTrashIcon boolean
module web.CalendarController
class CalendarController(parent, model, renderer, params)
parent (Widget
)
model (AbstractModel
)
renderer (AbstractRenderer
)
params (Object
)
method renderButtons([$node])
Render the buttons according to the CalendarView.buttons template and add listeners on it. Set this.$buttons with the produced jQuery element
- $node (
jQueryElement
) – a jQuery node where the rendered buttons
should be inserted. $node may be undefined, in which case the Calendar
inserts them into this.options.$buttons or into a div of its templatemodule web.DomainSelectorDialog
class DomainSelectorDialog(parent, model, domain, options)
parent
model
domain
options
module hr_attendance.my_attendances
class MyAttendances()
module account.payment
namespace
module project_scrum.dashboard
namespace
module web.Loading
class Loading(parent)
- parent
module web.AppsLauncher
class Apps(parent)
- parent
module web.AbstractField
class AbstractField(parent, name, record[, options])
parent (Widget
)
name (string
) – The field name defined in the model
record (Object
) – A record object (result of the get method of
a basic model)
options (AbstractFieldOptions
)
namespace fieldDependencies
An object representing fields to be fetched by the model eventhough not present in the view This object contains “field name” as key and an object as value. That value object must contain the key “type” see FieldBinaryImage for an example.
attribute resetOnAnyFieldChange Boolean
If this flag is set to true, the field widget will be reset on every change which is made in the view (if the view supports it). This is currently a form view feature.
attribute specialData Boolean
If this flag is given a string, the related BasicModel will be used to initialize specialData the field might need. This data will be available through this.record.specialData[this.name].
attribute supportedFieldTypes Array<String>
to override to indicate which field types are supported by the widget
method start() → Deferred
When a field widget is appended to the DOM, its start method is called, and will automatically call render. Most widgets should not override this.
method willStart()
Loads the libraries listed in this.jsLibs and this.cssLibs
method activate([options]) → boolean
Activates the field widget. By default, activation means focusing and selecting (if possible) the associated focusable element. The selecting part can be disabled. In that case, note that the focused input/textarea will have the cursor at the very end.
- options (
ActivateOptions
)boolean
class ActivateOptions()
attribute noselect boolean
- if false and the input
- is of type text or textarea, the content will also be selected
attribute event Event
the event which fired this activation
function commitChanges() → Deferred|undefined
This function should be implemented by widgets that are not able to notify their environment when their value changes (maybe because their are not aware of the changes) or that may have a value in a temporary state (maybe because some action should be performed to validate it before notifying it). This is typically called before trying to save the widget’s value, so it should call _setValue() to notify the environment if the value changed but was not notified.
Deferred
or undefinedfunction getFocusableElement() → jQuery
Returns the main field’s DOM element (jQuery form) which can be focused by the browser.
jQuery
function isFocusable() → boolean
Returns true iff the widget has a visible element that can take the focus
boolean
function isSet() → boolean
this method is used to determine if the field value is set to a meaningful value. This is useful to determine if a field should be displayed as empty
boolean
function isValid() → boolean
A field widget is valid if it was checked as valid the last time its value was changed by the user. This is checked before saving a record, by the view.
Note: this is the responsability of the view to check that required fields have a set value.
boolean
function reset(record[, event]) → Deferred
this method is supposed to be called from the outside of field widgets. The typical use case is when an onchange has changed the widget value. It will reset the widget to the values that could have changed, then will rerender the widget.
record (any
)
event (FlectraEvent
) – an event that triggered the reset action. It
is optional, and may be used by a widget to share information from the
moment a field change event is triggered to the moment a reset
operation is applied.
class AbstractFieldOptions()
attribute mode string
should be ‘readonly’ or ‘edit’
module hr_attendance.kiosk_confirm
class KioskConfirm(parent, action)
parent
action
module web.CustomizeSwitcher
class Theme()
module web.SystrayMenu
class SystrayMenu(parent)
- parent
The SystrayMenu is the class that manage the list of icons in the top right of the menu bar.
class SystrayMenu(parent)
- parent
The SystrayMenu is the class that manage the list of icons in the top right of the menu bar.
module web.Class
class Class()
Improved John Resig’s inheritance, based on:
Simple JavaScript Inheritance By John Resig http://ejohn.org/ MIT Licensed.
Adds “include()”
Defines The Class object. That object can be used to define and inherit classes using the extend() method.
Example:
var Person = Class.extend({
init: function(isDancing){
this.dancing = isDancing;
},
dance: function(){
return this.dancing;
}
});
The init() method act as a constructor. This class can be instanced this way:
var person = new Person(true);
person.dance();
The Person class can also be extended again:
var Ninja = Person.extend({
init: function(){
this._super( false );
},
dance: function(){
// Call the inherited version of dance()
return this._super();
},
swingSword: function(){
return true;
}
});
When extending a class, each re-defined method can use this._super() to call the previous implementation of that method.
method extend(prop)
Subclass an existing class
- prop (
Object
) – class-level properties (class attributes and instance methods) to set on the new classclass Class()
Improved John Resig’s inheritance, based on:
Simple JavaScript Inheritance By John Resig http://ejohn.org/ MIT Licensed.
Adds “include()”
Defines The Class object. That object can be used to define and inherit classes using the extend() method.
Example:
var Person = Class.extend({
init: function(isDancing){
this.dancing = isDancing;
},
dance: function(){
return this.dancing;
}
});
The init() method act as a constructor. This class can be instanced this way:
var person = new Person(true);
person.dance();
The Person class can also be extended again:
var Ninja = Person.extend({
init: function(){
this._super( false );
},
dance: function(){
// Call the inherited version of dance()
return this._super();
},
swingSword: function(){
return true;
}
});
When extending a class, each re-defined method can use this._super() to call the previous implementation of that method.
method extend(prop)
Subclass an existing class
- prop (
Object
) – class-level properties (class attributes and instance methods) to set on the new classmodule mail.ExtendedChatWindow
class ()
module web_editor.IframeRoot.instance
namespace
module web.Bookmark
namespace
module web.AbstractRenderer
class AbstractRenderer(parent, state, params)
parent (Widget
)
state (any
)
params (AbstractRendererParams
)
method start() → Deferred
The rendering can be asynchronous (but it is not encouraged). The start method simply makes sure that we render the view.
method on_attach_callback()
Called each time the renderer is attached into the DOM.
method on_detach_callback()
Called each time the renderer is detached from the DOM.
method getLocalState() → any
Returns any relevant state that the renderer might want to keep.
The idea is that a renderer can be destroyed, then be replaced by another one instantiated with the state from the model and the localState from the renderer, and the end result should be the same.
The kind of state that we expect the renderer to have is mostly DOM state such as the scroll position, the currently active tab page, …
This method is called before each updateState, by the controller.
any
method setLocalState(localState)
This is the reverse operation from getLocalState. With this method, we expect the renderer to restore all DOM state, if it is relevant.
This method is called after each updateState, by the controller.
- localState (
any
) – the result of a call to getLocalStatemethod updateState(state, params) → Deferred
Updates the state of the view. It retriggers a full rerender, unless told otherwise (for optimization for example).
state (any
)
params (UpdateStateParams
)
class UpdateStateParams()
attribute noRender boolean
if true, the method only updates the state without rerendering
class AbstractRendererParams()
attribute noContentHelp string
module mail.ChatterComposer
class ChatterComposer(parent, model, suggested_partners, options)
parent
model
suggested_partners
options
method prevent_send(event)
Send the message on SHIFT+ENTER, but go to new line on ENTER
- event
method get_checked_suggested_partners() → Array
Get the list of selected suggested partners
Array
method check_suggested_partners(checked_suggested_partners) → Deferred
Check the additional partners (not necessary registered partners), and open a popup form view for the ones who informations is missing.
- checked_suggested_partners (
Array
) – list of ‘recipient’ partners to complete informations or validatemodule web.AutoComplete
class (parent, options)
parent
options
module website_links.code_editor
namespace
module pos_restaurant.floors
namespace
module mail.systray
namespace
class ActivityMenu()
Menu item appended in the systray part of the navbar, redirects to the next activities of all app
class ActivityMenu()
Menu item appended in the systray part of the navbar, redirects to the next activities of all app
class MessagingMenu()
Menu item appended in the systray part of the navbar
The menu item indicates the counter of needactions + unread messages in chat channels. When clicking on it, it toggles a dropdown containing a preview of each pinned channels (except static and mass mailing channels) with a quick link to open them in chat windows. It also contains a direct link to the Inbox in Discuss.
module website_sale_wishlist.wishlist
namespace
module payment.payment_form
class PaymentForm(parent, options)
parent
options
module web_editor.snippets.options
namespace registry
The registry object contains the list of available options.
class margin-y()
Handles the edition of margin-top and margin-bottom.
class resize()
Handles the edition of snippet’s height.
class colorpicker()
Handles the edition of snippet’s background color classes.
class pos_background()
Handles the edition of snippet’s background image.
method background(previewMode, value, $li)
Handles a background change.
previewMode
value
$li
method chooseImage(previewMode, value, $li)
Opens a media dialog to add a custom background image.
previewMode
value
$li
method bindBackgroundEvents()
Attaches events so that when a background-color is set, the background image is removed.
class background_position()
Handles the edition of snippet’s background image position.
method backgroundPosition(previewMode, value, $li)
Opens a Dialog to edit the snippet’s backgroung image position.
previewMode
value
$li
class many2one()
Allows to replace a text value with the name of a database record.
class pos_background()
Handles the edition of snippet’s background image.
method background(previewMode, value, $li)
Handles a background change.
previewMode
value
$li
method chooseImage(previewMode, value, $li)
Opens a media dialog to add a custom background image.
previewMode
value
$li
method bindBackgroundEvents()
Attaches events so that when a background-color is set, the background image is removed.
namespace
class SnippetOption(parent, $target, $overlay, data)
parent
$target
$overlay
data
Handles a set of options for one snippet. The registry returned by this module contains the names of the specialized SnippetOption which can be referenced thanks to the data-js key in the web_editor options template.
attribute preventChildPropagation Boolean
When editing a snippet, its options are shown alongside the ones of its parent snippets. The parent options are only shown if the following flag is set to false (default).
method start()
Called when the option is initialized (i.e. the parent edition overlay is shown for the first time).
method onFocus()
Called when the parent edition overlay is covering the associated snippet (the first time, this follows the call to the @see start method).
method onBuilt()
Called when the parent edition overlay is covering the associated snippet for the first time, when it is a new snippet dropped from the d&d snippet menu. Note: this is called after the start and onFocus methods.
method onBlur()
Called when the parent edition overlay is removed from the associated snippet (another snippet enters edition for example).
method onClone(options)
Called when the associated snippet is the result of the cloning of
another snippet (so this.$target
is a cloned element).
- options (
OnCloneOptions
)class OnCloneOptions()
attribute isCurrent boolean
- true if the associated snippet is a clone of the main element that
- was cloned (so not a clone of a child of this main element that was cloned)
function onMove()
Called when the associated snippet is moved to another DOM location.
function onRemove()
Called when the associated snippet is about to be removed from the DOM.
function cleanForSave()
Called when the template which contains the associated snippet is about to be saved.
function selectClass(previewMode, value, $li)
Default option method which allows to select one and only one class in
the option classes set and set it on the associated snippet. The common
case is having a subdropdown with each <li/> having a data-select-class
value allowing to choose the associated class.
previewMode (boolean
or string
) – truthy if the option is enabled for preview or if leaving it (in
that second case, the value is ‘reset’)
- false if the option should be activated for good
value (any
) – the class to activate ($li.data(‘selectClass’))
$li (jQuery
) – the related DOMElement option
function toggleClass(previewMode, value, $li)
Default option method which allows to select one or multiple classes in
the option classes set and set it on the associated snippet. The common
case is having a subdropdown with each <li/> having a data-toggle-class
value allowing to toggle the associated class.
previewMode
value
$li
function $()
Override the helper method to search inside the $target element instead of the dropdown <li/> element.
function notify(name, data)
Sometimes, options may need to notify other options, even in parent editors. This can be done thanks to the ‘option_update’ event, which will then be handled by this function.
name (string
) – an identifier for a type of update
data (any
)
function setTarget($target)
Sometimes, an option is binded on an element but should in fact apply on another one. For example, elements which contain slides: we want all the per-slide options to be in the main menu of the whole snippet. This function allows to set the option’s target.
- $target (
jQuery
) – the new target elementnamespace registry
The registry object contains the list of available options.
class margin-y()
Handles the edition of margin-top and margin-bottom.
class resize()
Handles the edition of snippet’s height.
class colorpicker()
Handles the edition of snippet’s background color classes.
class pos_background()
Handles the edition of snippet’s background image.
method background(previewMode, value, $li)
Handles a background change.
previewMode
value
$li
method chooseImage(previewMode, value, $li)
Opens a media dialog to add a custom background image.
previewMode
value
$li
method bindBackgroundEvents()
Attaches events so that when a background-color is set, the background image is removed.
class background_position()
Handles the edition of snippet’s background image position.
method backgroundPosition(previewMode, value, $li)
Opens a Dialog to edit the snippet’s backgroung image position.
previewMode
value
$li
class many2one()
Allows to replace a text value with the name of a database record.
class pos_background()
Handles the edition of snippet’s background image.
method background(previewMode, value, $li)
Handles a background change.
previewMode
value
$li
method chooseImage(previewMode, value, $li)
Opens a media dialog to add a custom background image.
previewMode
value
$li
method bindBackgroundEvents()
Attaches events so that when a background-color is set, the background image is removed.
class SnippetOption(parent, $target, $overlay, data)
parent
$target
$overlay
data
Handles a set of options for one snippet. The registry returned by this module contains the names of the specialized SnippetOption which can be referenced thanks to the data-js key in the web_editor options template.
attribute preventChildPropagation Boolean
When editing a snippet, its options are shown alongside the ones of its parent snippets. The parent options are only shown if the following flag is set to false (default).
method start()
Called when the option is initialized (i.e. the parent edition overlay is shown for the first time).
method onFocus()
Called when the parent edition overlay is covering the associated snippet (the first time, this follows the call to the @see start method).
method onBuilt()
Called when the parent edition overlay is covering the associated snippet for the first time, when it is a new snippet dropped from the d&d snippet menu. Note: this is called after the start and onFocus methods.
method onBlur()
Called when the parent edition overlay is removed from the associated snippet (another snippet enters edition for example).
method onClone(options)
Called when the associated snippet is the result of the cloning of
another snippet (so this.$target
is a cloned element).
- options (
OnCloneOptions
)class OnCloneOptions()
attribute isCurrent boolean
- true if the associated snippet is a clone of the main element that
- was cloned (so not a clone of a child of this main element that was cloned)
function onMove()
Called when the associated snippet is moved to another DOM location.
function onRemove()
Called when the associated snippet is about to be removed from the DOM.
function cleanForSave()
Called when the template which contains the associated snippet is about to be saved.
function selectClass(previewMode, value, $li)
Default option method which allows to select one and only one class in
the option classes set and set it on the associated snippet. The common
case is having a subdropdown with each <li/> having a data-select-class
value allowing to choose the associated class.
previewMode (boolean
or string
) – truthy if the option is enabled for preview or if leaving it (in
that second case, the value is ‘reset’)
- false if the option should be activated for good
value (any
) – the class to activate ($li.data(‘selectClass’))
$li (jQuery
) – the related DOMElement option
function toggleClass(previewMode, value, $li)
Default option method which allows to select one or multiple classes in
the option classes set and set it on the associated snippet. The common
case is having a subdropdown with each <li/> having a data-toggle-class
value allowing to toggle the associated class.
previewMode
value
$li
function $()
Override the helper method to search inside the $target element instead of the dropdown <li/> element.
function notify(name, data)
Sometimes, options may need to notify other options, even in parent editors. This can be done thanks to the ‘option_update’ event, which will then be handled by this function.
name (string
) – an identifier for a type of update
data (any
)
function setTarget($target)
Sometimes, an option is binded on an element but should in fact apply on another one. For example, elements which contain slides: we want all the per-slide options to be in the main menu of the whole snippet. This function allows to set the option’s target.
- $target (
jQuery
) – the new target elementmodule point_of_sale.keyboard
namespace
module web.PivotController
class PivotController(parent, model, renderer, params)
parent
model
renderer
params (PivotControllerParams
)
method getContext() → Object
Returns the current measures and groupbys, so we can restore the view when we save the current state in the search view, or when we add it to the dashboard.
Object
method renderButtons([$node])
Render the buttons according to the PivotView.buttons template and add listeners on it. Set this.$buttons with the produced jQuery element
- $node (
jQuery
) – a jQuery node where the rendered buttons should
be inserted. $node may be undefined, in which case the PivotView
does nothingclass PivotControllerParams()
attribute groupableFields Object
- a map from field name to field
- props
attribute enableLinking boolean
- configure the pivot view to allow
- opening a list view by clicking on a cell with some data.
module web.BasicView
class BasicView(viewInfo)
- viewInfo
module web.PivotModel
class PivotModel(params)
- params (
Object
)method closeHeader(headerID) → Deferred
Close a header. This method is actually synchronous, but returns a deferred.
- headerID (
any
)method expandHeader(header, field)
Expand (open up) a given header, be it a row or a column.
header (any
)
field (any
)
method exportData() → Object
Export the current pivot view in a simple JS object.
Object
method flip()
Swap the columns and the rows. It is a synchronous operation.
method sortRows(col_id, measure, descending)
Sort the rows, depending on the values of a given column. This is an in-memory sort.
col_id (any
)
measure (any
)
descending (any
)
method toggleMeasure(field) → Deferred
Toggle the active state for a given measure, then reload the data.
- field (
string
)module website.content.compatibility
namespace
module pos_reprint.pos_reprint
namespace
module survey.result
namespace
module mail.ThreadField
class ThreadField()
module web_diagram.DiagramRenderer
class DiagramRenderer()
Diagram Renderer
The diagram renderer responsability is to render a diagram view, that is, a set of (labelled) nodes and edges. To do that, it uses the Raphael.js library.
class DiagramRenderer()
Diagram Renderer
The diagram renderer responsability is to render a diagram view, that is, a set of (labelled) nodes and edges. To do that, it uses the Raphael.js library.
module barcodes.field
namespace
module web.CrashManager
namespace ExceptionHandler
An interface to implement to handle exceptions. Register implementation in instance.web.crash_manager_registry.
function display()
Called to inform to display the widget, if necessary. A typical way would be to implement this interface in a class extending instance.web.Dialog and simply display the dialog in this method.
class CrashManager()
class RedirectWarningHandler(parent, error)
Handle redirection warnings, which behave more or less like a regular warning, with an additional redirection button.
module mail.chat_manager
object chat_manager instance of ChatManager
module web.Apps
class Apps(parent, action)
parent
action
module web.Bus
class Bus(parent)
Event Bus used to bind events scoped in the current instance
module web_editor.snippet.editor
class SnippetsMenu(parent, $editable)
parent
$editable
Management of drag&drop menu and snippet related behaviors in the page.
class SnippetEditor(parent, target, templateOptions)
Management of the overlay and option list for a snippet.
method buildSnippet()
Notifies all the associated snippet options that the snippet has just been dropped in the page.
method cleanForSave()
Notifies all the associated snippet options that the template which contains the snippet is about to be saved.
method cover()
Makes the editor overlay cover the associated snippet.
method toggleFocus(focus)
Displays/Hides the editor overlay and notifies the associated snippet options. Note: when it is displayed, this is here that the parent snippet options are moved to the editor overlay.
- focus (
boolean
) – true to display, false to hidenamespace
class SnippetsMenu(parent, $editable)
parent
$editable
Management of drag&drop menu and snippet related behaviors in the page.
class SnippetEditor(parent, target, templateOptions)
Management of the overlay and option list for a snippet.
method buildSnippet()
Notifies all the associated snippet options that the snippet has just been dropped in the page.
method cleanForSave()
Notifies all the associated snippet options that the template which contains the snippet is about to be saved.
method cover()
Makes the editor overlay cover the associated snippet.
method toggleFocus(focus)
Displays/Hides the editor overlay and notifies the associated snippet options. Note: when it is displayed, this is here that the parent snippet options are moved to the editor overlay.
- focus (
boolean
) – true to display, false to hidemodule web.ControlPanelMixin
namespace ControlPanelMixin
Mixin allowing widgets to communicate with the ControlPanel. Widgets needing a ControlPanel should use this mixin and call update_control_panel(cp_status) where cp_status contains information for the ControlPanel to update itself.
Note that the API is slightly awkward. Hopefully we will improve this when we get the time to refactor the control panel.
For example, here is what a typical client action would need to do to add support for a control panel with some buttons:
var ControlPanelMixin = require('web.ControlPanelMixin');
var SomeClientAction = Widget.extend(ControlPanelMixin, {
...
start: function () {
this._renderButtons();
this._updateControlPanel();
...
},
do_show: function () {
...
this._updateControlPanel();
},
_renderButtons: function () {
this.$buttons = $(QWeb.render('SomeTemplate.Buttons'));
this.$buttons.on('click', ...);
},
_updateControlPanel: function () {
this.update_control_panel({
breadcrumbs: this.action_manager.get_breadcrumbs(),
cp_content: {
$buttons: this.$buttons,
},
});
function update_control_panel([cp_status][, options])
Triggers ‘update’ on the cp_bus to update the ControlPanel according to cp_status
cp_status (Object
) – see web.ControlPanel.update() for a description
options (Object
) – see web.ControlPanel.update() for a description
namespace ControlPanelMixin
Mixin allowing widgets to communicate with the ControlPanel. Widgets needing a ControlPanel should use this mixin and call update_control_panel(cp_status) where cp_status contains information for the ControlPanel to update itself.
Note that the API is slightly awkward. Hopefully we will improve this when we get the time to refactor the control panel.
For example, here is what a typical client action would need to do to add support for a control panel with some buttons:
var ControlPanelMixin = require('web.ControlPanelMixin');
var SomeClientAction = Widget.extend(ControlPanelMixin, {
...
start: function () {
this._renderButtons();
this._updateControlPanel();
...
},
do_show: function () {
...
this._updateControlPanel();
},
_renderButtons: function () {
this.$buttons = $(QWeb.render('SomeTemplate.Buttons'));
this.$buttons.on('click', ...);
},
_updateControlPanel: function () {
this.update_control_panel({
breadcrumbs: this.action_manager.get_breadcrumbs(),
cp_content: {
$buttons: this.$buttons,
},
});
function update_control_panel([cp_status][, options])
Triggers ‘update’ on the cp_bus to update the ControlPanel according to cp_status
cp_status (Object
) – see web.ControlPanel.update() for a description
options (Object
) – see web.ControlPanel.update() for a description
module web.dom_ready
namespace def
module hr_attendance.greeting_message
class GreetingMessage(parent, action)
parent
action
module web.OrgChart
class FieldOrgChart()
module barcodes.BarcodeParser
class BarcodeParser(attributes)
- attributes
module web.GraphController
class GraphController(parent, model, renderer, params)
parent (Widget
)
model (GraphModel
)
renderer (GraphRenderer
)
params (GraphControllerParams
)
method getContext() → Object
Returns the current mode, measure and groupbys, so we can restore the view when we save the current state in the search view, or when we add it to the dashboard.
Object
method renderButtons([$node])
Render the buttons according to the GraphView.buttons and add listeners on it. Set this.$buttons with the produced jQuery element
- $node (
jQuery
) – a jQuery node where the rendered buttons should
be inserted $node may be undefined, in which case the GraphView does
nothingclass GraphControllerParams()
attribute measures string[]
module point_of_sale.screens
namespace
module account.ReconciliationRenderer
class StatementRenderer(parent, model, state)
rendering of the bank statement action contains progress bar, title and auto reconciliation button
method start()
display iniial state and create the name statement field
method update(state)
update the statement rendering
- state (
UpdateState
) – statement dataclass UpdateState()
statement data
attribute valuenow integer
for the progress bar
attribute valuemax integer
for the progress bar
attribute title string
for the progress bar
attribute notifications [object]
namespace
class StatementRenderer(parent, model, state)
rendering of the bank statement action contains progress bar, title and auto reconciliation button
method start()
display iniial state and create the name statement field
method update(state)
update the statement rendering
- state (
UpdateState
) – statement dataclass UpdateState()
statement data
attribute valuenow integer
for the progress bar
attribute valuemax integer
for the progress bar
attribute title string
for the progress bar
attribute notifications [object]
class ManualRenderer()
rendering of the manual reconciliation action contains progress bar, title and auto reconciliation button
class LineRenderer(parent, model, state)
rendering of the bank statement line, contains line data, proposition and view for ‘match’ and ‘create’ mode
method update(state)
update the statement line rendering
- state (
object
) – statement lineclass ManualLineRenderer()
rendering of the manual reconciliation, contains line data, proposition and view for ‘match’ mode
method start()
move the partner field
class ManualRenderer()
rendering of the manual reconciliation action contains progress bar, title and auto reconciliation button
class ManualLineRenderer()
rendering of the manual reconciliation, contains line data, proposition and view for ‘match’ mode
method start()
move the partner field
class LineRenderer(parent, model, state)
rendering of the bank statement line, contains line data, proposition and view for ‘match’ and ‘create’ mode
method update(state)
update the statement line rendering
- state (
object
) – statement linemodule website.WebsiteRoot
namespace
module web_editor.translate
namespace
module web.BasicModel
class BasicModel()
method addDefaultRecord(listID[, options]) → Deferred<string>
Add a default record to a list object. This method actually makes a new record with the _makeDefaultRecord method, then adds it to the list object. The default record is added in the data directly. This is meant to be used by list or kanban controllers (i.e. not for x2manys in form views, as in this case, we store changes as commands).
listID (string
) – a valid handle for a list object
options (AddDefaultRecordOptions
)
Deferred
<string
>class AddDefaultRecordOptions()
attribute position string
- if the new record should be added
- on top or on bottom of the list
function applyDefaultValues(recordID, values[, options]) → Deferred
Add and process default values for a given record. Those values are parsed and stored in the ‘_changes’ key of the record. For relational fields, sub-dataPoints are created, and missing relational data is fetched. Also generate default values for fields with no given value. Typically, this function is called with the result of a ‘default_get’ RPC, to populate a newly created dataPoint. It may also be called when a one2many subrecord is open in a form view (dialog), to generate the default values for the fields displayed in the o2m form view, but not in the list or kanban (mainly to correctly create sub-dataPoints for relational fields).
recordID (string
) – local id for a record
values (Object
) – dict of default values for the given record
options (ApplyDefaultValuesOptions
)
class ApplyDefaultValuesOptions()
attribute viewType string
- current viewType. If not set, we will
- assume main viewType from the record
attribute fieldNames Array
- list of field names for which a
- default value must be generated (used to complete the values dict)
function applyRawChanges(recordID, viewType) → Deferred<string>
Onchange RPCs may return values for fields that are not in the current view. Those fields might even be unknown when the onchange returns (e.g. in x2manys, we only know the fields that are used in the inner view, but not those used in the potential form view opened in a dialog when a sub- record is clicked). When this happens, we can’t infer their type, so the given value can’t be processed. It is instead stored in the ‘_rawChanges’ key of the record, without any processing. Later on, if this record is displayed in another view (e.g. the user clicked on it in the x2many list, and the record opens in a dialog), those changes that were left behind must be applied. This function applies changes stored in ‘_rawChanges’ for a given viewType.
recordID (string
) – local resource id of a record
viewType (string
) – the current viewType
Deferred
<string
>function deleteRecords(recordIds, modelName) → Deferred
Delete a list of records, then, if the records have a parent, reload it.
recordIds (Array
<string
>) – list of local resources ids. They should all
be of type ‘record’, be of the same model and have the same parent.
modelName (string
) – mode name used to unlink the records
function discardChanges(id[, options])
Discard all changes in a local resource. Basically, it removes everything that was stored in a _changes key.
id (string
) – local resource id
options (DiscardChangesOptions
)
class DiscardChangesOptions()
attribute rollback boolean
- if true, the changes will
- be reset to the last _savePoint, otherwise, they are reset to null
function duplicateRecord(recordID) → Deferred<string>
Duplicate a record (by calling the ‘copy’ route)
- recordID (
string
) – id for a local resourceDeferred
<string
>function get(id, options) → Object
The get method first argument is the handle returned by the load method. It is optional (the handle can be undefined). In some case, it makes sense to use the handle as a key, for example the BasicModel holds the data for various records, each with its local ID.
synchronous method, it assumes that the resource has already been loaded.
id (string
) – local id for the resource
options (GetOptions
)
Object
class GetOptions()
attribute env boolean
- if true, will only return res_id
- (if record) or res_ids (if list)
attribute raw boolean
if true, will not follow relations
function getName(id) → string
Returns the current display_name for the record.
- id (
string
) – the localID for a valid record elementstring
function canBeAbandoned(id) → boolean
Returns true if a record can be abandoned.
Case for not abandoning the record:
- flagged as ‘no abandon’ (i.e. during a
default_get
, including anyonchange
from adefault_get
)
- registered in a list on addition
- 2.1. registered as non-new addition 2.2. registered as new additon on update
- record is not new
Otherwise, the record can be abandoned.
This is useful when discarding changes on this record, as it means that we must keep the record even if some fields are invalids (e.g. required field is empty).
- id (
string
) – id for a local resourceboolean
function isDirty(id) → boolean
Returns true if a record is dirty. A record is considered dirty if it has some unsaved changes, marked by the _isDirty property on the record or one of its subrecords.
- id (
string
) – the local resource idboolean
function isNew(id) → boolean
Check if a localData is new, meaning if it is in the process of being created and no actual record exists in db. Note: if the localData is not of the “record” type, then it is always considered as not new.
Note: A virtual id is a character string composed of an integer and has a dash and other information. E.g: in calendar, the recursive event have virtual id linked to a real id virtual event id “23-20170418020000” is linked to the event id 23
- id (
string
) – id for a local resourceboolean
function load(params) → Deferred<string>
Main entry point, the goal of this method is to fetch and process all data (following relations if necessary) for a given record/list.
class LoadParams()
attribute fieldsInfo Object
contains the fieldInfo of each field
attribute fields Object
contains the description of each field
attribute type string
‘record’ or ‘list’
attribute recordID string
an ID for an existing resource.
function makeRecord(model, fields[, fieldInfo]) → string
This helper method is designed to help developpers that want to use a field widget outside of a view. In that case, we want a way to create data without actually performing a fetch.
model (string
) – name of the model
fields (Array
<Object
>) – a description of field properties
fieldInfo (Object
) – various field info that we want to set
string
function notifyChanges(record_id, changes[, options]) → string[]
This is an extremely important method. All changes in any field go through this method. It will then apply them in the local state, check if onchanges needs to be applied, actually do them if necessary, then resolves with the list of changed fields.
record_id (string
)
changes (Object
) – a map field => new value
options (Object
) – will be transferred to the applyChange method
Array
<string
>function reload(id[, options]) → Deferred<string>
Reload all data for a given resource. At any time there is at most one reload operation active.
id (string
) – local id for a resource
options (ReloadOptions
)
Deferred
<string
>class ReloadOptions()
attribute keepChanges boolean
- if true, doesn’t discard the
- changes on the record before reloading it
function removeLine(elementID)
In some case, we may need to remove an element from a list, without going through the notifyChanges machinery. The motivation for this is when the user click on ‘Add an item’ in a field one2many with a required field, then clicks somewhere else. The new line need to be discarded, but we don’t want to trigger a real notifyChanges (no need for that, and also, we don’t want to rerender the UI).
- elementID (
string
) – some valid element id. It is necessary that the
corresponding element has a parent.function resequence(modelName, resIDs, parentID[, options]) → Deferred<string>
Resequences records.
modelName (string
) – the resIDs model
resIDs (Array
<integer
>) – the new sequence of ids
parentID (string
) – the localID of the parent
options (ResequenceOptions
)
Deferred
<string
>class ResequenceOptions()
attribute offset integer
attribute field string
the field name used as sequence
function save(recordID[, options]) → Deferred
Save a local resource, if needed. This is a complicated operation, - it needs to check all changes, - generate commands for x2many fields, - call the /create or /write method according to the record status - After that, it has to reload all data, in case something changed, server side.
recordID
options (SaveOptions
)
class SaveOptions()
attribute reload boolean
if true, data will be reloaded
attribute savePoint boolean
- if true, the record will only
- be ‘locally’ saved: its changes written in a _savePoint key that can be restored later by call discardChanges with option rollback to true
attribute viewType string
- current viewType. If not set, we will
- assume main viewType from the record
function addFieldsInfo(recordID, viewInfo)
Completes the fields and fieldsInfo of a dataPoint with the given ones. It is useful for the cases where a record element is shared between various views, such as a one2many with a tree and a form view.
recordID (string
) – a valid element ID
viewInfo (AddFieldsInfoViewInfo
)
class AddFieldsInfoViewInfo()
attribute fields Object
attribute fieldsInfo Object
function freezeOrder(listID)
For list resources, this freezes the current records order.
- listID (
string
) – a valid element ID of type listfunction setDirty(id)
Manually sets a resource as dirty. This is used to notify that a field has been modified, but with an invalid value. In that case, the value is not sent to the basic model, but the record should still be flagged as dirty so that it isn’t discarded without any warning.
- id (
string
) – a resource idfunction setSort(list_id, fieldName) → Deferred
For list resources, this changes the orderedBy key.
list_id (string
) – id for the list resource
fieldName (string
) – valid field name
function toggleActive(recordIDs, value, parentID) → Deferred<string>
Toggle the active value of given records (to archive/unarchive them)
recordIDs (Array
) – local ids of the records to (un)archive
value (boolean
) – false to archive, true to unarchive (value of the active field)
parentID (string
) – id of the parent resource to reload
Deferred
<string
>function toggleGroup(groupId) → Deferred<string>
Toggle (open/close) a group in a grouped list, then fetches relevant data
function unfreezeOrder(elementID)
For a list datapoint, unfreezes the current records order and sorts it. For a record datapoint, unfreezes the x2many list datapoints.
- elementID (
string
) – a valid element IDfunction updateMessageIDs(id, msgIDs)
Update the message ids on a datapoint.
Note that we directly update the res_ids on the datapoint as the message has already been posted ; this change can’t be handled ‘normally’ with x2m commands because the change won’t be saved as a normal field.
id (string
)
msgIDs (Array
<integer
>)
module web.AbstractWebClient
class AbstractWebClient(parent)
method set_title(title)
Sets the first part of the title of the window, dedicated to the current action.
- title
method set_title_part(part, title)
Sets an arbitrary part of the title of the window. Title parts are
identified by strings. Each time a title part is changed, all parts
are gathered, ordered by alphabetical order and displayed in the title
of the window separated by -
.
part
title
method do_action()
When do_action is performed on the WebClient, forward it to the main ActionManager This allows to widgets that are not inside the ActionManager to perform do_action
module web.KanbanController
class KanbanController()
method renderButtons()
Extends the renderButtons function of ListView by adding an event listener on the import button.
method update() → Deferred
Override update method to recompute createColumnEnabled.
module website.content.lazy_template_call
class LazyTemplateRenderer()
method start()
Lazy replaces the [data-oe-call]
elements by their corresponding
template content.
module mass_mailing.unsubscribe
namespace
module web.special_fields
class FieldTimezoneMismatch()
This widget is intended to display a warning near a label of a ‘timezone’ field indicating if the browser timezone is identical (or not) to the selected timezone. This widget depends on a field given with the param ‘tz_offset_field’, which contains the time difference between UTC time and local time, in minutes.
namespace
class FieldTimezoneMismatch()
This widget is intended to display a warning near a label of a ‘timezone’ field indicating if the browser timezone is identical (or not) to the selected timezone. This widget depends on a field given with the param ‘tz_offset_field’, which contains the time difference between UTC time and local time, in minutes.
module web_tour.TourManager
class (parent, consumed_tours)
function register(name, [options, ]steps)
Registers a tour described by the following arguments in order
name (string
) – tour’s name
options (RegisterOptions
) – options (optional), available options are:
steps (Array
<Object
>) – steps’ descriptions, each step being an object
containing a tip description
class RegisterOptions()
options (optional), available options are:
attribute test boolean
true if this is only for tests
attribute skip_enabled boolean
true to add a link in its tips to consume the whole tour
attribute url string
the url to load when manually running the tour
attribute rainbowMan boolean
whether or not the rainbowman must be shown at the end of the tour
attribute wait_for Deferred
indicates when the tour can be started
function update(tour_name)
Checks for tooltips to activate (only from the running tour or specified tour if there is one, from all active tours otherwise). Should be called each time the DOM changes.
- tour_name
namespace STEPS
Tour predefined steps
module web.GraphView
class GraphView(viewInfo)
- viewInfo
module web_editor.rte.summernote
class SummernoteManager(parent)
module web.PivotRenderer
class PivotRenderer(parent, state, params)
parent
state
params (PivotRendererParams
)
class PivotRendererParams()
attribute widgets Object
- mapping (fieldName -> widget) used to
- format the cells
module stock.ReportWidget
class ReportWidget(parent)
- parent
module crm.partner_assign
namespace
module website_sale_stock.website_sale
namespace
module base_import.import
namespace
function jsonp(form, attributes, callback)
Safari does not deal well at all with raw JSON data being
returned. As a result, we’re going to cheat by using a
pseudo-jsonp: instead of getting JSON data in the iframe, we’re
getting a script
tag which consists of a function call and
the returned data (the json dump).
The function is an auto-generated name bound to window
,
which calls back into the callback provided here.
form (Object
) – the form element (DOM or jQuery) to use in the call
attributes (Object
) – jquery.form attributes object
callback (Function
) – function to call with the returned data
module FlectraLicensing.DialogRegisterContract
class (parent)
- parent
module point_of_sale.models
namespace exports
module website_event.registration_form.instance
namespace
module website_blog.editor
namespace
module web.CalendarModel
class ()
function calendarEventToRecord(event)
Transform fullcalendar event object to OpenERP Data object
- event
module web.ControlPanel
class ControlPanel(parent[, template])
parent
template (String
) – the QWeb template to render the ControlPanel.
By default, the template ‘ControlPanel’ will be used
method start() → jQuery.Deferred
Renders the control panel and creates a dictionnary of its exposed elements
jQuery.Deferred
method update(status, options)
Updates the content and displays the ControlPanel
status (UpdateStatus
)
options (UpdateOptions
)
class UpdateOptions()
attribute clear Boolean
set to true to clear from control panel elements that are not in status.cp_content
class UpdateStatus()
attribute active_view Object
the current active view
attribute breadcrumbs Array
the breadcrumbs to display (see _render_breadcrumbs() for precise description)
attribute cp_content Object
dictionnary containing the new ControlPanel jQuery elements
attribute hidden Boolean
true if the ControlPanel should be hidden
attribute searchview openerp.web.SearchView
the searchview widget
attribute search_view_hidden Boolean
true if the searchview is hidden, false otherwise
module web.GraphRenderer
class (parent, state, params)
function on_attach_callback()
The graph view uses the nv(d3) lib to render the graph. This lib requires that the rendering is done directly into the DOM (so that it can correctly compute positions). However, the views are always rendered in fragments, and appended to the DOM once ready (to prevent them from flickering). We here use the on_attach_callback hook, called when the widget is attached to the DOM, to perform the rendering. This ensures that the rendering is always done in the DOM.
class Params()
attribute stacked boolean
module website.customizeMenu
class CustomizeMenu()
module web.AjaxService
class AjaxService()
module web.AbstractService
function ()
Create subclass for AbstractService
class AbstractService()
module web.CalendarRenderer
class (parent, state, params)
parent (Widget
)
state (Object
)
params (Object
)
function getAvatars(record, fieldName, imageField) → string[]
Note: this is not dead code, it is called by the calendar-box template
record (any
)
fieldName (any
)
imageField (any
)
Array
<string
>function getColor(key) → integer
Note: this is not dead code, it is called by two template
- key (
any
)integer
module web.DebugManager
class DebugManager()
DebugManager base + general features (applicable to any context)
method perform_callback(evt)
Calls the appropriate callback when clicking on a Debug option
- evt
method perform_js_tests()
Runs the JS (desktop) tests
method perform_js_mobile_tests()
Runs the JS mobile tests
class DebugManager()
DebugManager base + general features (applicable to any context)
method perform_callback(evt)
Calls the appropriate callback when clicking on a Debug option
- evt
method perform_js_tests()
Runs the JS (desktop) tests
method perform_js_mobile_tests()
Runs the JS mobile tests
module stock.stock_report_generic
class stock_report_generic(parent, action)
module bus.bus
namespace bus
class CrossTabBus()
CrossTabBus Widget
Manage the communication before browser tab to allow only one tab polling for the others (performance improvement) When a tab is opened, and the start_polling method is called, the tab is signaling through the localStorage to the others. When a tab is closed, it signals its removing. If he was the master tab (the polling one), he choose another one in the list of open tabs. This one start polling for the other. When a notification is recieved from the poll, it is signaling through the localStorage too.
localStorage used keys are:
- bus.channels : shared public channel list to listen during the poll
- bus.options : shared options
- bus.notification : the received notifications from the last poll
- bus.tab_list : list of opened tab ids
- bus.tab_master : generated id of the master tab
module website.content.snippets.animation
namespace registry
The registry object contains the list of available animations.
class _fixAppleCollapse()
This is a fix for apple device (<= IPhone 4, IPad 2) Standard bootstrap requires data-toggle=’collapse’ element to be <a/> tags. Unfortunatly one snippet uses a <div/> tag instead. The fix forces an empty click handler on these div, which allows standard bootstrap to work.
This should be removed in a future flectra snippets refactoring.
class Animation(parent, editableMode)
parent (Object
)
editableMode (boolean
) – true if the page is in edition mode
Provides a way for executing code once a website DOM element is loaded in the dom and handle the case where the website edit mode is triggered.
Also register AnimationEffect automatically (@see effects, _prepareEffects).
attribute selector Boolean
The selector attribute, if defined, allows to automatically create an
instance of this animation on page load for each DOM element which
matches this selector. The Animation.$target
element will then be that
particular DOM element. This should be the main way of instantiating
Animation
elements.
namespace edit_events
Acts as @see Widget.events except that the events are only binded if the Animation instance is instanciated in edit mode.
namespace read_events
Acts as @see Widget.events except that the events are only binded if the Animation instance is instanciated in readonly mode.
attribute maxFPS Number
The max FPS at which all the automatic animation effects will be running by default.
method start()
Initializes the animation. The method should not be called directly as called automatically on animation instantiation and on restart.
Also, prepares animation’s effects and start them if any.
method destroy()
Destroys the animation and basically restores the target to the state it was before the start method was called (unlike standard widget, the associated $el DOM is not removed).
Also stops animation effects and destroys them if any.
class AnimationEffect(parent, updateCallback[, startEvents][, $startTarget][, options])
parent (Object
)
updateCallback (function
) – the animation update callback
startEvents=scroll (string
) – space separated list of events which starts the animation loop
$startTarget=window (jQuery
or DOMElement
) – the element(s) on which the startEvents are listened
options (AnimationEffectOptions
)
In charge of handling one animation loop using the requestAnimationFrame
feature. This is used by the Animation
class below and should not be called
directly by an end developer.
This uses a simple API: it can be started, stopped, played and paused.
method start()
Initializes when the animation must be played and paused and initializes the animation first frame.
method stop()
Pauses the animation and destroys the attached events which trigger the animation to be played or paused.
method play(e)
Forces the requestAnimationFrame loop to start.
- e (
Event
) – the event which triggered the animation to playmethod pause()
Forces the requestAnimationFrame loop to stop.
class AnimationEffectOptions()
attribute getStateCallback function
- a function which returns a value which represents the state of the
- animation, i.e. for two same value, no refreshing of the animation is needed. Can be used for optimization. If the $startTarget is the window element, this defaults to returning the current scoll offset of the window or the size of the window for the scroll and resize events respectively.
attribute endEvents string
- space separated list of events which pause the animation loop. If
- not given, the animation is stopped after a while (if no startEvents is received again)
attribute $endTarget jQuery|DOMElement
the element(s) on which the endEvents are listened
namespace
class Animation(parent, editableMode)
parent (Object
)
editableMode (boolean
) – true if the page is in edition mode
Provides a way for executing code once a website DOM element is loaded in the dom and handle the case where the website edit mode is triggered.
Also register AnimationEffect automatically (@see effects, _prepareEffects).
attribute selector Boolean
The selector attribute, if defined, allows to automatically create an
instance of this animation on page load for each DOM element which
matches this selector. The Animation.$target
element will then be that
particular DOM element. This should be the main way of instantiating
Animation
elements.
namespace edit_events
Acts as @see Widget.events except that the events are only binded if the Animation instance is instanciated in edit mode.
namespace read_events
Acts as @see Widget.events except that the events are only binded if the Animation instance is instanciated in readonly mode.
attribute maxFPS Number
The max FPS at which all the automatic animation effects will be running by default.
method start()
Initializes the animation. The method should not be called directly as called automatically on animation instantiation and on restart.
Also, prepares animation’s effects and start them if any.
method destroy()
Destroys the animation and basically restores the target to the state it was before the start method was called (unlike standard widget, the associated $el DOM is not removed).
Also stops animation effects and destroys them if any.
namespace registry
The registry object contains the list of available animations.
class _fixAppleCollapse()
This is a fix for apple device (<= IPhone 4, IPad 2) Standard bootstrap requires data-toggle=’collapse’ element to be <a/> tags. Unfortunatly one snippet uses a <div/> tag instead. The fix forces an empty click handler on these div, which allows standard bootstrap to work.
This should be removed in a future flectra snippets refactoring.
module web_editor.ready
namespace
module website_sale_comparison.comparison
namespace
module web.DataExport
class DataExport(parent, record)
parent
record
module web.rpc
namespace
function query(params, options) → Deferred<any>
Perform a RPC. Please note that this is not the preferred way to do a rpc if you are in the context of a widget. In that case, you should use the this._rpc method.
params (Object
) – @see buildQuery for a description
options (Object
)
Deferred
<any
>module website_quote.website_quote
namespace
module web.core
attribute debug Boolean
Whether the client is currently in “debug” mode
namespace
attribute debug Boolean
Whether the client is currently in “debug” mode
class Class()
Improved John Resig’s inheritance, based on:
Simple JavaScript Inheritance By John Resig http://ejohn.org/ MIT Licensed.
Adds “include()”
Defines The Class object. That object can be used to define and inherit classes using the extend() method.
Example:
var Person = Class.extend({
init: function(isDancing){
this.dancing = isDancing;
},
dance: function(){
return this.dancing;
}
});
The init() method act as a constructor. This class can be instanced this way:
var person = new Person(true);
person.dance();
The Person class can also be extended again:
var Ninja = Person.extend({
init: function(){
this._super( false );
},
dance: function(){
// Call the inherited version of dance()
return this._super();
},
swingSword: function(){
return true;
}
});
When extending a class, each re-defined method can use this._super() to call the previous implementation of that method.
method extend(prop)
Subclass an existing class
- prop (
Object
) – class-level properties (class attributes and instance methods) to set on the new classfunction _t(source) → String
Eager translation function, performs translation immediately at call
site. Beware using this outside of method bodies (before the
translation database is loaded), you probably want _lt()
instead.
- source (
String
) – string to translateString
function _lt(s) → Object
Lazy translation function, only performs the translation when actually printed (e.g. inserted into a template)
Useful when defining translatable strings in code evaluated before the translation database is loaded, as class attributes or at the top-level of an OpenERP Web module
- s (
String
) – string to translateObject
module web.GanttModel
class (params)
- params (
Object
)module website.utils
namespace
function autocompleteWithPages(self, $input)
Allows the given input to propose existing website URLs.
self (ServicesMixin
or Widget
) – an element capable to trigger an RPC
$input (jQuery
)
function autocompleteWithPages(self, $input)
Allows the given input to propose existing website URLs.
self (ServicesMixin
or Widget
) – an element capable to trigger an RPC
$input (jQuery
)
module hr_attendance.kiosk_mode
class KioskMode()
module web_tour.tour
namespace
module web_editor.editor
namespace
module website.newMenu
class NewContentMenu()
module website_slides.slides
namespace
module account.ReconciliationClientAction
class ManualAction()
Widget used as action for ‘account.move.line’ and ‘res.partner’ for the manual reconciliation and mark data as reconciliate
class StatementAction(parent, params)
Widget used as action for ‘account.bank.statement’ reconciliation
method willStart()
instantiate the action renderer
method start()
append the renderer and instantiate the line renderers
method do_show()
update the control panel and breadcrumbs
class StatementActionParams()
attribute context Object
namespace
class StatementAction(parent, params)
Widget used as action for ‘account.bank.statement’ reconciliation
method willStart()
instantiate the action renderer
method start()
append the renderer and instantiate the line renderers
method do_show()
update the control panel and breadcrumbs
class StatementActionParams()
attribute context Object
class ManualAction()
Widget used as action for ‘account.move.line’ and ‘res.partner’ for the manual reconciliation and mark data as reconciliate
module web.Sidebar
class Sidebar(parent, options)
parent
options
method start()
Get the attachment linked to the record when the toolbar started
module web.data_manager
object data_manager instance of
module web.planner
namespace
module web.utils
namespace utils
function assert(bool)
Throws an error if the given condition is not true
- bool (
any
)function binaryToBinsize(value) → string
Check if the value is a bin_size or not. If not, compute an approximate size out of the base64 encoded string.
- value (
string
) – original formatstring
function confine([val][, min][, max]) → number
Confines a value inside an interval
val (number
) – the value to confine
min (number
) – the minimum of the interval
max (number
) – the maximum of the interval
number
function divmod(a, b, fn)
computes (Math.floor(a/b), a%b and passes that to the callback.
returns the callback’s result
a
b
fn
function generateID() → integer
Generate a unique numerical ID
integer
function get_cookie(c_name) → string
Read the cookie described by c_name
- c_name (
string
)string
function human_number(number[, decimals][, minDigits][, formatterCallback]) → string
Returns a human readable number (e.g. 34000 -> 34k).
number (number
)
decimals=0 (integer
) – maximum number of decimals to use in human readable representation
minDigits=1 (integer
) – the minimum number of digits to preserve when switching to another
level of thousands (e.g. with a value of ‘2’, 4321 will still be
represented as 4321 otherwise it will be down to one digit (4k))
formatterCallback (function
) – a callback to transform the final number before adding the
thousands symbol (default to adding thousands separators (useful
if minDigits > 1))
string
function human_size(size)
Returns a human readable size
- size (
Number
) – number of bytesfunction insert_thousand_seps(num) → String
Insert “thousands” separators in the provided number (which is actually a string)
- num (
String
)String
function intersperse(str, indices, separator) → String
Intersperses separator
in str
at the positions indicated by
indices
.
indices
is an array of relative offsets (from the previous insertion
position, starting from the end of the string) at which to insert
separator
.
There are two special values:
-1
- indicates the insertion should end now
0
- indicates that the previous section pattern should be repeated (until all
of
str
is consumed)
str (String
)
indices (Array
<Number
>)
separator (String
)
String
function lpad(str, size) → string
Left-pad provided arg 1 with zeroes until reaching size provided by second argument.
str (number
or string
) – value to pad
size (number
) – size to reach on the final padded value
string
function modf(x, fn)
Passes the fractional and integer parts of x to the callback, returns the callback’s result
x
fn
function round_decimals(value, decimals)
performs a half up rounding with a fixed amount of decimals, correcting for float loss of precision See the corresponding float_round() in server/tools/float_utils.py for more info
value (Number
) – the value to be rounded
decimals (Number
) – the number of decimals. eg: round_decimals(3.141592,2) -> 3.14
function round_precision(value, precision)
performs a half up rounding with arbitrary precision, correcting for float loss of precision See the corresponding float_round() in server/tools/float_utils.py for more info
value (number
) – the value to be rounded
precision (number
) – a precision parameter. eg: 0.01 rounds to two digits.
function set_cookie(name, value, ttl)
Create a cookie
name (String
) – the name of the cookie
value (String
) – the value stored in the cookie
ttl (Integer
) – time to live of the cookie in millis. -1 to erase the cookie.
function stableSort(array, iteratee)
Sort an array in place, keeping the initial order for identical values.
array (Array
)
iteratee (function
)
function traverse(tree, f)
Visit a tree of objects, where each children are in an attribute ‘children’. For each children, we call the callback function given in arguments.
tree (Object
) – an object describing a tree structure
f (function
) – a callback
function deepFreeze(obj)
Visit a tree of objects and freeze all
- obj (
Object
)module web.crash_manager
object instance of CrashManager
module mail.ChatThread
class Thread(parent, options)
parent
options
method insert_read_more($element)
Modifies $element to add the ‘read more/read less’ functionality
All element nodes with “data-o-mail-quote” attribute are concerned.
All text nodes after a #stopSpelling
element are concerned.
Those text nodes need to be wrapped in a span (toggle functionality).
All consecutive elements are joined in one ‘read more/read less’.
- $element
method remove_message_and_render([message_id][, messages][, options])
Removes a message and re-renders the thread
message_id (int
) – the id of the removed message
messages (array
) – the list of messages to display, without the removed one
options (object
) – options for the thread rendering
method scroll_to(options)
Scrolls the thread to a given message or offset if any, to bottom otherwise
- options (
ScrollToOptions
)class ScrollToOptions()
attribute id int
optional: the id of the message to scroll to
attribute offset int
optional: the number of pixels to scroll
module web.ServicesMixin
mixin ServicesMixin
function do_action(action, options) → Deferred
Informs the action manager to do an action. This supposes that the action manager can be found amongst the ancestors of the current widget. If that’s not the case this method will simply return an unresolved deferred.
action (any
)
options (any
)
module web_editor.base
namespace
function ready() → Deferred
If a widget needs to be instantiated on page loading, it needs to wait for appropriate resources to be loaded. This function returns a Deferred which is resolved when the dom is ready, the session is bound (translations loaded) and the XML is loaded. This should however not be necessary anymore as widgets should not be parentless and should then be instantiated (directly or not) by the page main component (webclient, website root, editor bar, …). The DOM will be ready then, the main component is in charge of waiting for the session and the XML can be lazy loaded thanks to the @see Widget.xmlDependencies key.
module web_tour.Tip
class Tip(parent[, info])
parent (Widget
)
info (Object
) – description of the tip, containing the following keys:
- content [String] the html content of the tip
- event_handlers [Object] description of optional event handlers to bind to the tip:
- event [String] the event name
- selector [String] the jQuery selector on which the event should be bound
- handler [function] the handler
- position [String] tip’s position (‘top’, ‘right’, ‘left’ or ‘bottom’), default ‘right’
- width [int] the width in px of the tip when opened, default 270
- space [int] space in px between anchor and tip, default 10
- overlay [Object] x and y values for the number of pixels the mouseout detection area
overlaps the opened tip, default {x: 50, y: 50}
module web.KanbanColumn
class KanbanColumn()
method addQuickCreate()
Adds the quick create record to the top of the column.
module website_sale.website_sale_category
namespace
module web.Domain
class Domain(domain[, evalContext])
domain (string
or Array
or boolean
or undefined) – The given domain can be:
* a string representation of the Python prefix-array
representation of the domain.
* a JS prefix-array representation of the domain.
* a boolean where the “true” domain match all records and the
“false” domain does not match any records.
* undefined, considered as the false boolean.
* a number, considered as true except 0 considered as false.
evalContext (Object
) – in case the given domain is a string, an
evaluation context might be needed
The Domain Class allows to work with a domain as a tree and provides tools to manipulate array and string representations of domains.
method compute(values) → boolean
Evaluates the domain with a set of values.
- values (
Object
) – a mapping {fieldName -> fieldValue} (note: all
the fields used in the domain should be given a
value otherwise the computation will break)boolean
method toArray() → Array
Return the JS prefix-array representation of this domain. Note that all domains that use the “false” domain cannot be represented as such.
Array
method arrayToString(domain) → string
Converts JS prefix-array representation of a domain to a string representation of the Python prefix-array representation of this domain.
- domain (
Array
or string
)string
method stringToArray(domain[, evalContext]) → Array
Converts a string representation of the Python prefix-array representation of a domain to a JS prefix-array representation of this domain.
domain (string
or Array
)
evalContext (Object
)
Array
method normalizeArray(domain) → Array
Makes implicit “&” operators explicit in the given JS prefix-array representation of domain (e.g [A, B] -> [“&”, A, B])
- domain (
Array
) – the JS prefix-array representation of the domain
to normalize (! will be normalized in-place)Array
class Domain(domain[, evalContext])
domain (string
or Array
or boolean
or undefined) – The given domain can be:
* a string representation of the Python prefix-array
representation of the domain.
* a JS prefix-array representation of the domain.
* a boolean where the “true” domain match all records and the
“false” domain does not match any records.
* undefined, considered as the false boolean.
* a number, considered as true except 0 considered as false.
evalContext (Object
) – in case the given domain is a string, an
evaluation context might be needed
The Domain Class allows to work with a domain as a tree and provides tools to manipulate array and string representations of domains.
method compute(values) → boolean
Evaluates the domain with a set of values.
- values (
Object
) – a mapping {fieldName -> fieldValue} (note: all
the fields used in the domain should be given a
value otherwise the computation will break)boolean
method toArray() → Array
Return the JS prefix-array representation of this domain. Note that all domains that use the “false” domain cannot be represented as such.
Array
method arrayToString(domain) → string
Converts JS prefix-array representation of a domain to a string representation of the Python prefix-array representation of this domain.
- domain (
Array
or string
)string
method stringToArray(domain[, evalContext]) → Array
Converts a string representation of the Python prefix-array representation of a domain to a JS prefix-array representation of this domain.
domain (string
or Array
)
evalContext (Object
)
Array
method normalizeArray(domain) → Array
Makes implicit “&” operators explicit in the given JS prefix-array representation of domain (e.g [A, B] -> [“&”, A, B])
- domain (
Array
) – the JS prefix-array representation of the domain
to normalize (! will be normalized in-place)Array
module web.framework
function redirect(url, wait)
Redirect to url by replacing window.location If wait is true, sleep 1s and wait for the server i.e. after a restart.
url
wait
function ReloadContext(parent, action)
Client action to refresh the session context (making sure HTTP requests will have the right one) then reload the whole interface.
parent
action
function blockAccessKeys()
Remove the “accesskey” attributes to avoid the use of the access keys while the blockUI is enable.
function Home(parent, action)
Client action to go back home.
parent
action
function HistoryBack(parent)
Client action to go back in breadcrumb history. If can’t go back in history stack, will go back to home.
- parent
namespace
function redirect(url, wait)
Redirect to url by replacing window.location If wait is true, sleep 1s and wait for the server i.e. after a restart.
url
wait
module web.GraphModel
class (parent)
- parent (
Widget
)function get() → Object
We defend against outside modifications by extending the chart data. It may be overkill.
Object
function load(params) → Deferred
Initial loading.
- params (
LoadParams
)class LoadParams()
attribute mode string
one of ‘pie’, ‘bar’, ‘line
attribute measure string
a valid field name
attribute groupBys string[]
a list of valid field names
attribute context Object
attribute domain string[]
function reload(handle, params) → Deferred
Reload data. It is similar to the load function. Note that we ignore the handle parameter, we always expect our data to be in this.chart object.
handle (any
) – ignored!
params (ReloadParams
)
class ReloadParams()
attribute domain string[]
attribute groupBy string[]
attribute mode string
one of ‘bar’, ‘pie’, ‘line’
attribute measure string
a valid field name
module website.ace
class WebsiteAceEditor()
Extends the default view editor so that the URL hash is updated with view ID
class WebsiteAceEditor()
Extends the default view editor so that the URL hash is updated with view ID
module web_editor.ace
function _getCheckReturn(isValid[, errorLine][, errorMessage]) → Object
Formats a content-check result (@see checkXML, checkLESS).
isValid (boolean
)
errorLine (integer
) – needed if isValid is false
errorMessage (string
) – needed if isValid is false
Object
function formatLESS(less) → string
Formats some LESS so that it has proper indentation and structure.
- less (
string
)string
class ViewEditor(parent, viewKey[, options])
parent (Widget
)
viewKey (string
or integer
) – xml_id or id of the view whose linked resources have to be loaded.
options (ViewEditorOptions
)
Allows to visualize resources (by default, XML views) and edit them.
method willStart()
Loads everything the ace library needs to work. It also loads the resources to visualize (@see _loadResources).
method start()
Initializes the library and initial view once the DOM is ready. It also initializes the resize feature of the ace editor.
class ViewEditorOptions()
attribute initialResID string|integer
- a specific view ID / LESS URL to load on start (otherwise the main
- view ID associated with the specified viewKey will be used)
attribute position string
attribute doNotLoadViews boolean
attribute doNotLoadLess boolean
attribute includeBundles boolean
attribute includeAllLess boolean
attribute defaultBundlesRestriction string[]
function formatXML(xml) → string
Formats some XML so that it has proper indentation and structure.
- xml (
string
)string
function checkXML(xml) → Object
Checks the syntax validity of some XML.
- xml (
string
)Object
namespace checkLESS
Checks the syntax validity of some LESS.
class ViewEditor(parent, viewKey[, options])
parent (Widget
)
viewKey (string
or integer
) – xml_id or id of the view whose linked resources have to be loaded.
options (ViewEditorOptions
)
Allows to visualize resources (by default, XML views) and edit them.
method willStart()
Loads everything the ace library needs to work. It also loads the resources to visualize (@see _loadResources).
method start()
Initializes the library and initial view once the DOM is ready. It also initializes the resize feature of the ace editor.
class ViewEditorOptions()
attribute initialResID string|integer
- a specific view ID / LESS URL to load on start (otherwise the main
- view ID associated with the specified viewKey will be used)
attribute position string
attribute doNotLoadViews boolean
attribute doNotLoadLess boolean
attribute includeBundles boolean
attribute includeAllLess boolean
attribute defaultBundlesRestriction string[]
module web.PivotView
class PivotView(viewInfo, params)
viewInfo
params (Object
)
module web.dom
function _notify([content, ]callbacks)
Private function to notify that something has been attached in the DOM
content (htmlString
or Element
or Array
or jQuery
) – the content that
has been attached in the DOM
callbacks
namespace
function append([$target, ][content, ]options)
Appends content in a jQuery object and optionnally triggers an event
$target (jQuery
) – the node where content will be appended
content (htmlString
or Element
or Array
or jQuery
) – DOM element,
array of elements, HTML string or jQuery object to append to $target
options (AppendOptions
)
class AppendOptions()
attribute in_DOM Boolean
true if $target is in the DOM
attribute callbacks Array
- array of objects describing the
- callbacks to perform (see _notify for a complete description)
function autoresize($textarea, options)
Autoresize a $textarea node, by recomputing its height when necessary
$textarea
options (AutoresizeOptions
)
class AutoresizeOptions()
attribute min_height number
by default, 50.
attribute parent Widget
- if set, autoresize will listen to some
- extra events to decide when to resize itself. This is useful for widgets that are not in the dom when the autoresize is declared.
function cssFind($from, selector) → jQuery
jQuery find function behavior is:
$('A').find('A B') <=> $('A A B')
The searches behavior to find options’ DOM needs to be:
$('A').find('A B') <=> $('A B')
This is what this function does.
$from (jQuery
) – the jQuery element(s) from which to search
selector (string
) – the CSS selector to match
jQuery
function detach([to_detach, ]options) → jQuery
Detaches widgets from the DOM and performs their on_detach_callback()
to_detach (Array
) – array of {widget: w, callback_args: args} such
that w.$el will be detached and w.on_detach_callback(args) will be
called
options (DetachOptions
)
jQuery
class DetachOptions()
attribute $to_detach jQuery
- if given, detached instead of
- widgets’ $el
function getSelectionRange(node) → Object
Returns the selection range of an input or textarea
- node (
Object
) – DOM item input or textearaObject
function getPosition(e) → Object
Returns the distance between a DOM element and the top-left corner of the window
- e (
Object
) – DOM element (input or texteara)Object
function prepend([$target, ][content, ]options)
Prepends content in a jQuery object and optionnally triggers an event
$target (jQuery
) – the node where content will be prepended
content (htmlString
or Element
or Array
or jQuery
) – DOM element,
array of elements, HTML string or jQuery object to prepend to $target
options (PrependOptions
)
class PrependOptions()
attribute in_DOM Boolean
true if $target is in the DOM
attribute callbacks Array
- array of objects describing the
- callbacks to perform (see _notify for a complete description)
function renderButton(options) → jQuery
Renders a button with standard flectra template. This does not use any xml template to avoid forcing the frontend part to lazy load a xml file for each widget which might want to create a simple button.
- options (
RenderButtonOptions
)jQuery
class RenderButtonOptions()
attribute attrs Object
Attributes to put on the button element
attribute attrs.type string
attribute attrs.class string
- Note: automatically completed with “btn btn-X” (@see options.size
- for the value of X)
attribute size string
@see options.attrs.class
attribute icon string
- The specific fa icon class (for example “fa-home”) or an URL for
- an image to use as icon.
attribute text string
the button’s text
function renderCheckbox([options]) → jQuery
Renders a checkbox with standard flectra template. This does not use any xml template to avoid forcing the frontend part to lazy load a xml file for each widget which might want to create a simple checkbox.
- options (
RenderCheckboxOptions
)jQuery
class RenderCheckboxOptions()
attribute prop Object
Allows to set the input properties (disabled and checked states).
attribute text string
- The checkbox’s associated text. If none is given then a simple
- checkbox without label structure is rendered.
function setSelectionRange(node, range)
Sets the selection range of a given input or textarea
node (Object
) – DOM element (input or textarea)
range (SetSelectionRangeRange
)
class SetSelectionRangeRange()
attribute start integer
attribute end integer
module base.settings
namespace
module web.Registry
class Registry([mapping])
- mapping (
Object
) – the initial data in the registryThe registry is really pretty much only a mapping from some keys to some values. The Registry class only add a few simple methods around that to make it nicer and slightly safer.
Note that registries have a fundamental problem: the value that you try to get in a registry might not have been added yet, so of course, you need to make sure that your dependencies are solid. For this reason, it is a good practice to avoid using the registry if you can simply import what you need with the ‘require’ statement.
However, on the flip side, sometimes you cannot just simply import something because we would have a dependency cycle. In that case, registries might help.
method add(key, value) → Registry
Add a key (and a value) to the registry.
method contains(key) → boolean
Check if the registry contains the value
- key (
string
)boolean
method extend([mapping])
Creates and returns a copy of the current mapping, with the provided mapping argument added in (replacing existing keys if needed)
Parent and child remain linked, a new key in the parent (which is not overwritten by the child) will appear in the child.
- mapping={} (
Object
) – a mapping of keys to object-pathsmethod get(key) → any
Returns the value associated to the given key.
- key (
string
)any
method getAny(keys) → any
Tries a number of keys, and returns the first object matching one of the keys.
- keys (
Array
<string
>) – a sequence of keys to fetch the object forany
class Registry([mapping])
- mapping (
Object
) – the initial data in the registryThe registry is really pretty much only a mapping from some keys to some values. The Registry class only add a few simple methods around that to make it nicer and slightly safer.
Note that registries have a fundamental problem: the value that you try to get in a registry might not have been added yet, so of course, you need to make sure that your dependencies are solid. For this reason, it is a good practice to avoid using the registry if you can simply import what you need with the ‘require’ statement.
However, on the flip side, sometimes you cannot just simply import something because we would have a dependency cycle. In that case, registries might help.
method add(key, value) → Registry
Add a key (and a value) to the registry.
method contains(key) → boolean
Check if the registry contains the value
- key (
string
)boolean
method extend([mapping])
Creates and returns a copy of the current mapping, with the provided mapping argument added in (replacing existing keys if needed)
Parent and child remain linked, a new key in the parent (which is not overwritten by the child) will appear in the child.
- mapping={} (
Object
) – a mapping of keys to object-pathsmethod get(key) → any
Returns the value associated to the given key.
- key (
string
)any
method getAny(keys) → any
Tries a number of keys, and returns the first object matching one of the keys.
- keys (
Array
<string
>) – a sequence of keys to fetch the object forany
module web.basic_fields
class FieldDomain()
The “Domain” field allows the user to construct a technical-prefix domain thanks to a tree-like interface and see the selected records in real time. In debug mode, an input is also there to be able to enter the prefix char domain directly (or to build advanced domains the tree-like interface does not allow to).
attribute specialData String
Fetches the number of records which are matched by the domain (if the domain is not server-valid, the value is false) and the model the field must work with.
method isSet()
A domain field is always set since the false value is considered to be equal to “[]” (match all records).
class AceEditor()
This widget is intended to be used on Text fields. It will provide Ace Editor for editing XML and Python.
class HandleWidget()
Displays a handle to modify the sequence.
class FieldProgressBar()
Node options:
- title: title of the bar, displayed on top of the bar options
- editable: boolean if value is editable
- current_value: get the current_value from the field that must be present in the view
- max_value: get the max_value from the field that must be present in the view
- edit_max_value: boolean if the max_value is editable
- title: title of the bar, displayed on top of the bar –> not translated, use parameter “title” instead
class FieldToggleBoolean()
This widget is intended to be used on boolean fields. It toggles a button switching between a green bullet / gray bullet.
method isSet()
A boolean field is always set since false is a valid value.
namespace
class FieldDomain()
The “Domain” field allows the user to construct a technical-prefix domain thanks to a tree-like interface and see the selected records in real time. In debug mode, an input is also there to be able to enter the prefix char domain directly (or to build advanced domains the tree-like interface does not allow to).
attribute specialData String
Fetches the number of records which are matched by the domain (if the domain is not server-valid, the value is false) and the model the field must work with.
method isSet()
A domain field is always set since the false value is considered to be equal to “[]” (match all records).
class FieldProgressBar()
Node options:
- title: title of the bar, displayed on top of the bar options
- editable: boolean if value is editable
- current_value: get the current_value from the field that must be present in the view
- max_value: get the max_value from the field that must be present in the view
- edit_max_value: boolean if the max_value is editable
- title: title of the bar, displayed on top of the bar –> not translated, use parameter “title” instead
class FieldToggleBoolean()
This widget is intended to be used on boolean fields. It toggles a button switching between a green bullet / gray bullet.
method isSet()
A boolean field is always set since false is a valid value.
class HandleWidget()
Displays a handle to modify the sequence.
class AceEditor()
This widget is intended to be used on Text fields. It will provide Ace Editor for editing XML and Python.
module web_editor.backend
class FieldTextHtmlSimple()
- TranslatableFieldMixin
FieldTextHtmlSimple Widget Intended to display HTML content. This widget uses the summernote editor improved by flectra.
method commitChanges()
Summernote doesn’t notify for changes done in code mode. We override commitChanges to manually switch back to normal mode before committing changes, so that the widget is aware of the changes done in code mode.
method reset(record, event)
Do not re-render this field if it was the origin of the onchange call.
record
event
namespace
class FieldTextHtmlSimple()
- TranslatableFieldMixin
FieldTextHtmlSimple Widget Intended to display HTML content. This widget uses the summernote editor improved by flectra.
method commitChanges()
Summernote doesn’t notify for changes done in code mode. We override commitChanges to manually switch back to normal mode before committing changes, so that the widget is aware of the changes done in code mode.
method reset(record, event)
Do not re-render this field if it was the origin of the onchange call.
record
event
module website.WebsiteRoot.instance
namespace
module report.utils
namespace
module web.field_utils
function formatX2Many(value) → string
Returns a string indicating the number of records in the relation.
- value (
Object
) – a valid element from a BasicModel, that represents a
list of valuesstring
function formatBinary([value][, field][, options]) → string
Convert binary to bin_size
value (string
) – base64 representation of the binary (might be already a bin_size!)
field (Object
) – a description of the field (note: this parameter is ignored)
options (Object
) – additional options (note: this parameter is ignored)
string
function formatInteger(value[, field][, options]) → string
Returns a string representing an integer. If the value is false, then we return an empty string.
value (integer
or false)
field (Object
) – a description of the field (note: this parameter is ignored)
options (FormatIntegerOptions
) – additional options
string
class FormatIntegerOptions()
additional options
attribute isPassword boolean
if true, returns ‘****’
function parseInteger(value) → integer
Parse a String containing integer with language formating
- value (
string
) – The string to be parsed with the setting of thousands and
decimal separatorinteger
function formatSelection(value[, field][, options])
Returns a string representing the value of the selection.
value (string
or false)
field (Object
) – a description of the field (note: this parameter is ignored)
options (FormatSelectionOptions
) – additional options
class FormatSelectionOptions()
additional options
attribute escape boolean
if true, escapes the formatted value
function parseMany2one(value) → Object
Creates an object with id and display_name.
- value (
Array
or number
or string
or Object
) – The given value can be :
- an array with id as first element and display_name as second element
- a number or a string representing the id (the display_name will be
returned as undefined)
- an object, simply returned untouchedObject
namespace
function parseFloat(value) → float
Parse a String containing float in language formating
- value (
string
) – The string to be parsed with the setting of thousands and
decimal separatorfloat
function formatDateTime(value[, field][, options]) → string
Returns a string representing a datetime. If the value is false, then we return an empty string. Note that this is dependant on the localization settings
value
field (Object
) – a description of the field (note: this parameter is ignored)
options (FormatDateTimeOptions
) – additional options
string
class FormatDateTimeOptions()
additional options
attribute timezone boolean
- use the user timezone when formating the
- date
function formatDate(value[, field][, options]) → string
Returns a string representing a date. If the value is false, then we return an empty string. Note that this is dependant on the localization settings
value (Moment
or false)
field (Object
) – a description of the field (note: this parameter is ignored)
options (FormatDateOptions
) – additional options
string
class FormatDateOptions()
additional options
attribute timezone boolean
- use the user timezone when formating the
- date
function parseNumber(value) → float|NaN
Parse a String containing number in language formating
- value (
string
) – The string to be parsed with the setting of thousands and
decimal separatorfloat
or NaN
function parseMonetary(value[, field][, options]) → float
Parse a String containing currency symbol and returns amount
value (string
) – The string to be parsed
We assume that a monetary is always a pair (symbol, amount) separated
by a non breaking space. A simple float can also be accepted as value
field (Object
) – a description of the field (returned by fields_get for example).
options (ParseMonetaryOptions
) – additional options.
float
class ParseMonetaryOptions()
additional options.
attribute currency Object
the description of the currency to use
attribute currency_id integer
the id of the ‘res.currency’ to use (ignored if options.currency)
attribute currency_field string
- the name of the field whose value is the currency id
- (ignore if options.currency or options.currency_id) Note: if not given it will default to the field currency_field value or to ‘currency_id’.
attribute data Object
- a mapping of field name to field value, required with
- options.currency_field
function parseDateTime(value[, field][, options]) → Moment|false
Create an Date object The method toJSON return the formated value to send value server side
value (string
)
field (Object
) – a description of the field (note: this parameter is ignored)
options (ParseDateTimeOptions
) – additional options
Moment
or falseclass ParseDateTimeOptions()
additional options
attribute isUTC boolean
the formatted date is utc
attribute timezone boolean
- format the date after apply the timezone
- offset
function formatChar(value[, field][, options]) → string
Returns a string representing a char. If the value is false, then we return an empty string.
value (string
or false)
field (Object
) – a description of the field (note: this parameter is ignored)
options (FormatCharOptions
) – additional options
string
class FormatCharOptions()
additional options
attribute escape boolean
if true, escapes the formatted value
attribute isPassword boolean
- if true, returns ‘****’
- instead of the formatted value
function formatMany2one(value[, field][, options]) → string
Returns a string representing an many2one. If the value is false, then we return an empty string. Note that it accepts two types of input parameters: an array, in that case we assume that the many2one value is of the form [id, nameget], and we return the nameget, or it can be an object, and in that case, we assume that it is a record from a BasicModel.
value (Array
or Object
or false)
field (Object
) – a description of the field (note: this parameter is ignored)
options (FormatMany2oneOptions
) – additional options
string
class FormatMany2oneOptions()
additional options
attribute escape boolean
if true, escapes the formatted value
function parseDate(value[, field][, options]) → Moment|false
Create an Date object The method toJSON return the formated value to send value server side
value (string
)
field (Object
) – a description of the field (note: this parameter is ignored)
options (ParseDateOptions
) – additional options
Moment
or falseclass ParseDateOptions()
additional options
attribute isUTC boolean
the formatted date is utc
attribute timezone boolean
- format the date after apply the timezone
- offset
function formatFloat(value[, field][, options]) → string
Returns a string representing a float. The result takes into account the user settings (to display the correct decimal separator).
value (float
or false) – the value that should be formatted
field (Object
) – a description of the field (returned by fields_get
for example). It may contain a description of the number of digits that
should be used.
options (FormatFloatOptions
) – additional options to override the values in the
python description of the field.
string
class FormatFloatOptions()
- additional options to override the values in the
- python description of the field.
attribute digits integer[]
- the number of digits that should be used,
- instead of the default digits precision in the field.
function formatMonetary(value[, field][, options]) → string
Returns a string representing a monetary value. The result takes into account the user settings (to display the correct decimal separator, currency, …).
value (float
or false) – the value that should be formatted
field (Object
) – a description of the field (returned by fields_get for example). It
may contain a description of the number of digits that should be used.
options (FormatMonetaryOptions
) – additional options to override the values in the python description of
the field.
string
class FormatMonetaryOptions()
- additional options to override the values in the python description of
- the field.
attribute currency Object
the description of the currency to use
attribute currency_id integer
the id of the ‘res.currency’ to use (ignored if options.currency)
attribute currency_field string
- the name of the field whose value is the currency id
- (ignore if options.currency or options.currency_id) Note: if not given it will default to the field currency_field value or to ‘currency_id’.
attribute data Object
- a mapping of field name to field value, required with
- options.currency_field
attribute digits integer[]
- the number of digits that should be used, instead of the default
- digits precision in the field. Note: if the currency defines a precision, the currency’s one is used.
function formatFloatTime(value) → string
Returns a string representing a time value, from a float. The idea is that we sometimes want to display something like 1:45 instead of 1.75, or 0:15 instead of 0.25.
- value (
float
)string
module web_diagram.DiagramController
class DiagramController(parent, model, renderer, params)
parent (Widget
)
model (DiagramModel
)
renderer (DiagramRenderer
)
params (Object
)
Diagram Controller
method renderButtons([$node])
Render the buttons according to the DiagramView.buttons template and add listeners on it. Set this.$buttons with the produced jQuery element
- $node (
jQuery
) – a jQuery node where the rendered buttons should
be inserted $node may be undefined, in which case they are inserted
into this.options.$buttonsclass DiagramController(parent, model, renderer, params)
parent (Widget
)
model (DiagramModel
)
renderer (DiagramRenderer
)
params (Object
)
Diagram Controller
method renderButtons([$node])
Render the buttons according to the DiagramView.buttons template and add listeners on it. Set this.$buttons with the produced jQuery element
- $node (
jQuery
) – a jQuery node where the rendered buttons should
be inserted $node may be undefined, in which case they are inserted
into this.options.$buttonsmodule account.ReconciliationModel
namespace
class StatementModel(parent, options)
parent (Widget
)
options (object
)
Model use to fetch, format and update ‘account.bank.statement’ and ‘account.bank.statement.line’ datas allowing reconciliation
The statement internal structure:
{
valuenow: integer
valuenow: valuemax
[bank_statement_id]: {
id: integer
display_name: string
}
reconcileModels: [object]
accounts: {id: code}
}
The internal structure of each line is:
{
balance: {
type: number - show/hide action button
amount: number - real amount
amount_str: string - formated amount
account_code: string
},
st_line: {
partner_id: integer
partner_name: string
}
mode: string ('inactive', 'match', 'create')
reconciliation_proposition: {
id: number|string
partial_reconcile: boolean
invalid: boolean - through the invalid line (without account, label...)
is_tax: boolean
account_code: string
date: string
date_maturity: string
label: string
amount: number - real amount
amount_str: string - formated amount
[already_paid]: boolean
[partner_id]: integer
[partner_name]: string
[account_code]: string
[journal_id]: {
id: integer
display_name: string
}
[ref]: string
[is_partially_reconciled]: boolean
[amount_currency_str]: string|false (amount in record currency)
}
mv_lines: object - idem than reconciliation_proposition
offset: integer
limitMoveLines: integer
filter: string
[createForm]: {
account_id: {
id: integer
display_name: string
}
tax_id: {
id: integer
display_name: string
}
analytic_account_id: {
id: integer
display_name: string
}
label: string
amount: number,
[journal_id]: {
id: integer
display_name: string
}
}
}
method addProposition(handle, mv_line_id) → Deferred
add a reconciliation proposition from the matched lines We also display a warning if the user tries to add 2 line with different account type
handle (string
)
mv_line_id (number
)
method autoReconciliation() → Deferred<Object>
send information ‘account.bank.statement.line’ model to reconciliate lines, call rpc to ‘reconciliation_widget_auto_reconcile’ Update the number of validated line
Deferred
<Object
>method changeFilter(handle, filter) → Deferred
change the filter for the target line and fetch the new matched lines
handle (string
)
filter (string
)
method changeMode(handle, mode) → Deferred
change the mode line (‘inactive’, ‘match’, ‘create’), and fetch the new matched lines or prepare to create a new line
match
- display the matched lines, the user can select the lines to apply there as proposition
create
- display fields and quick create button to create a new proposition for the reconciliation
handle (string
)
mode (inactive or match or create)
method changeName(name) → Deferred
call ‘write’ method on the ‘account.bank.statement’
- name (
string
)method changeOffset(handle, offset) → Deferred
change the offset for the matched lines, and fetch the new matched lines
handle (string
)
offset (number
)
method changePartner(handle, partner) → Deferred
change the partner on the line and fetch the new matched lines
handle (string
)
partner (ChangePartnerPartner
)
class ChangePartnerPartner()
attribute display_name string
attribute id number
function closeStatement() → Deferred<number>
close the statement
Deferred
<number
>function createProposition(handle) → Deferred
then open the first available line
- handle (
string
)function getContext() → Object
Return context information and journal_id
Object
function getStatementLines() → Object
Return the lines that needs to be displayed by the widget
Object
function hasMoreLines() → boolean
Return a boolean telling if load button needs to be displayed or not
boolean
function getLine(handle) → Object
get the line data for this handle
- handle (
Object
)Object
function load(context) → Deferred
load data from
- ‘account.bank.statement’ fetch the line id and bank_statement_id info
- ‘account.reconcile.model’ fetch all reconcile model (for quick add)
- ‘account.account’ fetch all account code
- ‘account.bank.statement.line’ fetch each line data
- context (
LoadContext
)class LoadContext()
attribute statement_ids number[]
function loadMore(qty) → Deferred
Load more bank statement line
- qty (
integer
) – quantity to loadfunction loadData(ids, excluded_ids) → Deferred
RPC method to load informations on lines
ids (Array
) – ids of bank statement line passed to rpc call
excluded_ids (Array
) – list of move_line ids that needs to be excluded from search
function quickCreateProposition(handle, reconcileModelId) → Deferred
Add lines into the propositions from the reconcile model Can add 2 lines, and each with its taxes. The second line become editable in the create mode.
handle (string
)
reconcileModelId (integer
)
function removeProposition(handle, id) → Deferred
Remove a proposition and switch to an active mode (‘create’ or ‘match’)
handle (string
)
id (number
) – (move line id)
function togglePartialReconcile(handle) → Deferred
Force the partial reconciliation to display the reconciliate button. This method should only be called when there is onely one proposition.
- handle (
string
)function updateProposition(handle, values) → Deferred
Change the value of the editable proposition line or create a new one.
If the editable line comes from a reconcile model with 2 lines and their ‘amount_type’ is “percent” and their total equals 100% (this doesn’t take into account the taxes who can be included or not) Then the total is recomputed to have 100%.
handle (string
)
values (any
)
function validate(handle) → Deferred<Object>
Format the value and send it to ‘account.bank.statement.line’ model Update the number of validated lines
- handle (
string
or Array
<string
>)Deferred
<Object
>class ManualModel()
Model use to fetch, format and update ‘account.move.line’ and ‘res.partner’ datas allowing manual reconciliation
method hasMoreLines() → boolean
Return a boolean telling if load button needs to be displayed or not
boolean
method load(context) → Deferred
load data from - ‘account.move.line’ fetch the lines to reconciliate - ‘account.account’ fetch all account code
- context (
LoadContext
)class LoadContext()
attribute mode string
‘customers’, ‘suppliers’ or ‘accounts’
attribute company_ids integer[]
attribute partner_ids integer[]
- used for ‘customers’ and
- ‘suppliers’ mode
function loadMore(qty) → Deferred
Load more partners/accounts
- qty (
integer
) – quantity to loadfunction loadData(lines) → Deferred
Method to load informations on lines
- lines (
Array
) – manualLines to loadfunction validate(handle) → Deferred<Array>
Mark the account or the partner as reconciled
- handle (
string
or Array
<string
>)Deferred
<Array
>class StatementModel(parent, options)
parent (Widget
)
options (object
)
Model use to fetch, format and update ‘account.bank.statement’ and ‘account.bank.statement.line’ datas allowing reconciliation
The statement internal structure:
{
valuenow: integer
valuenow: valuemax
[bank_statement_id]: {
id: integer
display_name: string
}
reconcileModels: [object]
accounts: {id: code}
}
The internal structure of each line is:
{
balance: {
type: number - show/hide action button
amount: number - real amount
amount_str: string - formated amount
account_code: string
},
st_line: {
partner_id: integer
partner_name: string
}
mode: string ('inactive', 'match', 'create')
reconciliation_proposition: {
id: number|string
partial_reconcile: boolean
invalid: boolean - through the invalid line (without account, label...)
is_tax: boolean
account_code: string
date: string
date_maturity: string
label: string
amount: number - real amount
amount_str: string - formated amount
[already_paid]: boolean
[partner_id]: integer
[partner_name]: string
[account_code]: string
[journal_id]: {
id: integer
display_name: string
}
[ref]: string
[is_partially_reconciled]: boolean
[amount_currency_str]: string|false (amount in record currency)
}
mv_lines: object - idem than reconciliation_proposition
offset: integer
limitMoveLines: integer
filter: string
[createForm]: {
account_id: {
id: integer
display_name: string
}
tax_id: {
id: integer
display_name: string
}
analytic_account_id: {
id: integer
display_name: string
}
label: string
amount: number,
[journal_id]: {
id: integer
display_name: string
}
}
}
method addProposition(handle, mv_line_id) → Deferred
add a reconciliation proposition from the matched lines We also display a warning if the user tries to add 2 line with different account type
handle (string
)
mv_line_id (number
)
method autoReconciliation() → Deferred<Object>
send information ‘account.bank.statement.line’ model to reconciliate lines, call rpc to ‘reconciliation_widget_auto_reconcile’ Update the number of validated line
Deferred
<Object
>method changeFilter(handle, filter) → Deferred
change the filter for the target line and fetch the new matched lines
handle (string
)
filter (string
)
method changeMode(handle, mode) → Deferred
change the mode line (‘inactive’, ‘match’, ‘create’), and fetch the new matched lines or prepare to create a new line
match
- display the matched lines, the user can select the lines to apply there as proposition
create
- display fields and quick create button to create a new proposition for the reconciliation
handle (string
)
mode (inactive or match or create)
method changeName(name) → Deferred
call ‘write’ method on the ‘account.bank.statement’
- name (
string
)method changeOffset(handle, offset) → Deferred
change the offset for the matched lines, and fetch the new matched lines
handle (string
)
offset (number
)
method changePartner(handle, partner) → Deferred
change the partner on the line and fetch the new matched lines
handle (string
)
partner (ChangePartnerPartner
)
class ChangePartnerPartner()
attribute display_name string
attribute id number
function closeStatement() → Deferred<number>
close the statement
Deferred
<number
>function createProposition(handle) → Deferred
then open the first available line
- handle (
string
)function getContext() → Object
Return context information and journal_id
Object
function getStatementLines() → Object
Return the lines that needs to be displayed by the widget
Object
function hasMoreLines() → boolean
Return a boolean telling if load button needs to be displayed or not
boolean
function getLine(handle) → Object
get the line data for this handle
- handle (
Object
)Object
function load(context) → Deferred
load data from
- ‘account.bank.statement’ fetch the line id and bank_statement_id info
- ‘account.reconcile.model’ fetch all reconcile model (for quick add)
- ‘account.account’ fetch all account code
- ‘account.bank.statement.line’ fetch each line data
- context (
LoadContext
)class LoadContext()
attribute statement_ids number[]
function loadMore(qty) → Deferred
Load more bank statement line
- qty (
integer
) – quantity to loadfunction loadData(ids, excluded_ids) → Deferred
RPC method to load informations on lines
ids (Array
) – ids of bank statement line passed to rpc call
excluded_ids (Array
) – list of move_line ids that needs to be excluded from search
function quickCreateProposition(handle, reconcileModelId) → Deferred
Add lines into the propositions from the reconcile model Can add 2 lines, and each with its taxes. The second line become editable in the create mode.
handle (string
)
reconcileModelId (integer
)
function removeProposition(handle, id) → Deferred
Remove a proposition and switch to an active mode (‘create’ or ‘match’)
handle (string
)
id (number
) – (move line id)
function togglePartialReconcile(handle) → Deferred
Force the partial reconciliation to display the reconciliate button. This method should only be called when there is onely one proposition.
- handle (
string
)function updateProposition(handle, values) → Deferred
Change the value of the editable proposition line or create a new one.
If the editable line comes from a reconcile model with 2 lines and their ‘amount_type’ is “percent” and their total equals 100% (this doesn’t take into account the taxes who can be included or not) Then the total is recomputed to have 100%.
handle (string
)
values (any
)
function validate(handle) → Deferred<Object>
Format the value and send it to ‘account.bank.statement.line’ model Update the number of validated lines
- handle (
string
or Array
<string
>)Deferred
<Object
>class ManualModel()
Model use to fetch, format and update ‘account.move.line’ and ‘res.partner’ datas allowing manual reconciliation
method hasMoreLines() → boolean
Return a boolean telling if load button needs to be displayed or not
boolean
method load(context) → Deferred
load data from - ‘account.move.line’ fetch the lines to reconciliate - ‘account.account’ fetch all account code
- context (
LoadContext
)class LoadContext()
attribute mode string
‘customers’, ‘suppliers’ or ‘accounts’
attribute company_ids integer[]
attribute partner_ids integer[]
- used for ‘customers’ and
- ‘suppliers’ mode
function loadMore(qty) → Deferred
Load more partners/accounts
- qty (
integer
) – quantity to loadfunction loadData(lines) → Deferred
Method to load informations on lines
- lines (
Array
) – manualLines to loadfunction validate(handle) → Deferred<Array>
Mark the account or the partner as reconciled
- handle (
string
or Array
<string
>)Deferred
<Array
>module mail.DocumentViewer
class DocumentViewer(parent, attachments, activeAttachmentID)
parent
attachments (Array
<Object
>) – list of attachments
activeAttachmentID (integer
)
method start()
Open a modal displaying the active attachment
module web.KanbanColumnProgressBar
class KanbanColumnProgressBar(parent, options, columnState)
parent
options
columnState
attribute ANIMATE boolean
Allows to disable animations for tests.
module mail.utils
namespace
module portal.portal
namespace
module web.BasicController
class BasicController(parent, model, renderer, params)
method canBeDiscarded([recordID]) → Deferred<boolean>
Determines if we can discard the current changes. If the model is not dirty, that is not a problem. However, if it is dirty, we have to ask the user for confirmation.
- recordID (
string
) – default to main recordIDDeferred
<boolean
>method canBeSaved([recordID]) → boolean
Ask the renderer if all associated field widget are in a valid state for saving (valid value and non-empty value for required fields). If this is not the case, this notifies the user with a warning containing the names of the invalid fields.
Note: changing the style of invalid fields is the renderer’s job.
- recordID (
string
) – default to main recordIDboolean
method discardChanges(recordID, options)
Waits for the mutex to be unlocked and then calls _.discardChanges. This ensures that the confirm dialog isn’t displayed directly if there is a pending ‘write’ rpc.
recordID
options
method getSelectedIds() → Array
Method that will be overriden by the views with the ability to have selected ids
Array
method saveRecord([recordID][, options]) → Deferred
Saves the record whose ID is given if necessary (@see _saveRecord).
recordID (string
) – default to main recordID
options (Object
)
class BasicControllerParams()
attribute archiveEnabled boolean
attribute confirmOnDelete boolean
attribute hasButtons boolean
module website_links.website_links
namespace exports
module web.KanbanView
class KanbanView()
module web.pyeval
function ensure_evaluated(args, kwargs)
If args or kwargs are unevaluated contexts or domains (compound or not), evaluated them in-place.
Potentially mutates both parameters.
args
kwargs
function tmxxx(year, month, day, hour, minute, second, microsecond)
Converts the stuff passed in into a valid date, applying overflows as needed
year
month
day
hour
minute
second
microsecond
function context_today() → datetime.date
Returns the current local date, which means the date on the client (which can be different compared to the date of the server).
datetime.date
function get_normalized_domain(domain_array) → Array
Returns a normalized copy of the given domain array. Normalization is is making the implicit “&” at the start of the domain explicit, e.g. [A, B, C] would become [“&”, “&”, A, B, C].
- domain_array (
Array
)Array
namespace
function ensure_evaluated(args, kwargs)
If args or kwargs are unevaluated contexts or domains (compound or not), evaluated them in-place.
Potentially mutates both parameters.
args
kwargs
module website.planner
class WebsitePlannerLauncher()
module web_editor.summernote
module web.session
object session instance of Session
module web.FormController
class FormController()
method autofocus()
Calls autofocus on the renderer
method createRecord([parentID]) → Deferred
This method switches the form view in edit mode, with a new record.
- parentID (
string
) – if given, the parentID will be used as parent
for the new record.method getSelectedIds() → number[]
Returns the current res_id, wrapped in a list. This is only used by the sidebar (and the debugmanager)
Array
<number
>method on_attach_callback()
Called each time the form view is attached into the DOM
method renderButtons($node)
Render buttons for the control panel. The form view can be rendered in a dialog, and in that case, if we have buttons defined in the footer, we have to use them instead of the standard buttons.
- $node (
jQueryElement
)method renderPager($node, options)
The form view has to prevent a click on the pager if the form is dirty
$node (jQueryElement
)
options (Object
)
method renderSidebar([$node])
Instantiate and render the sidebar if a sidebar is requested Sets this.sidebar
- $node (
jQuery
) – a jQuery node where the sidebar should be
insertedmethod saveRecord()
Show a warning message if the user modified a translated field. For each field, the notification provides a link to edit the field’s translations.
method update(params, options)
Overrides to force the viewType to ‘form’, so that we ensure that the correct fields are reloaded (this is only useful for one2many form views).
params
options
module web.ActionManager
class WidgetAction(action, widget)
action
widget
Specialization of Action for client actions that are Widgets
method restore()
Restores WidgetAction by calling do_show on its widget
method detach() → *
Detaches the action’s widget from the DOM
method destroy()
Destroys the widget
class ViewManagerAction()
Specialization of WidgetAction for window actions (i.e. ViewManagers)
method restore([view_index])
Restores a ViewManagerAction Switches to the requested view by calling select_view on the ViewManager
- view_index (
int
) – the index of the view to selectmethod set_on_reverse_breadcrumb([callback][, scrollTop])
Sets the on_reverse_breadcrumb_callback and the scrollTop to apply when coming back to that action
callback (Function
) – the callback
scrollTop (int
) – the number of pixels to scroll
method setScrollTop([scrollTop])
Sets the scroll position of the widgets’s active_view
- scrollTop (
integer
) – the number of pixels to scrollmethod getScrollTop() → integer
Returns the current scrolling offset for the current action. We have to ask nicely the question to the active view, because the answer depends on the view.
integer
class Action(action)
- action
Class representing the actions of the ActionManager Basic implementation for client actions that are functions
method restore() → Deferred
This method should restore this previously loaded action Calls on_reverse_breadcrumb_callback if defined
method detach()
There is nothing to detach in the case of a client function
method destroy()
Destroyer: there is nothing to destroy in the case of a client function
method set_on_reverse_breadcrumb([callback])
Sets the on_reverse_breadcrumb_callback to be called when coming back to that action
- callback (
Function
) – the callbackmethod setScrollTop()
Not implemented for client actions
method set_fragment([$fragment])
Stores the DOM fragment of the action
- $fragment (
jQuery
) – the DOM fragmentmethod getScrollTop() → int
Not implemented for client actions
int
class ActionManager(parent, options)
parent
options
method on_attach_callback()
Called each time the action manager is attached into the DOM
method on_detach_callback()
Called each time the action manager is detached from the DOM
method push_action(widget, action_descr, options)
Add a new action to the action manager
widget (Widget
) – typically, widgets added are openerp.web.ViewManager. The action manager uses the stack of actions to handle the breadcrumbs.
action_descr (Object
) – new action description
options (PushActionOptions
)
class PushActionOptions()
attribute on_reverse_breadcrumb
will be called when breadcrumb is clicked on
attribute clear_breadcrumbs
boolean, if true, action stack is destroyed
function do_action(action[, options]) → jQuery.Deferred
Execute an OpenERP action
action (Number
or String
or String
or Object
) – Can be either an action id, an action XML id, a client action tag or an action descriptor.
options (DoActionOptions
)
jQuery.Deferred
class DoActionOptions()
attribute clear_breadcrumbs Boolean
Clear the breadcrumbs history list
attribute replace_breadcrumb Boolean
Replace the current breadcrumb with the action
attribute on_reverse_breadcrumb Function
Callback to be executed whenever an anterior breadcrumb item is clicked on.
attribute hide_breadcrumb Function
Do not display this widget’s title in the breadcrumb
attribute on_close Function
Callback to be executed when the dialog is closed (only relevant for target=new actions)
attribute action_menu_id Function
Manually set the menu id on the fly.
attribute additional_context Object
Additional context to be merged with the action’s context.
module web.CalendarQuickCreate
class QuickCreate(parent, buttons, options, dataTemplate, dataCalendar)
parent (Widget
)
buttons (Object
)
options (Object
)
dataTemplate (Object
)
dataCalendar (Object
)
Quick creation view.
Triggers a single event “added” with a single parameter “name”, which is the name entered by the user
class QuickCreate(parent, buttons, options, dataTemplate, dataCalendar)
parent (Widget
)
buttons (Object
)
options (Object
)
dataTemplate (Object
)
dataCalendar (Object
)
Quick creation view.
Triggers a single event “added” with a single parameter “name”, which is the name entered by the user
module mail.chat_mixin
namespace ChatMixin
module web.FormRenderer
class FormRenderer(parent, state, params)
parent
state
params
method autofocus()
Focuses the field having attribute ‘default_focus’ set, if any, or the first focusable field otherwise.
method canBeSaved(recordID) → string[]
Extend the method so that labels also receive the ‘o_field_invalid’ class if necessary.
- recordID (
string
)Array
<string
>method displayTranslationAlert(alertFields)
Show a warning message if the user modified a translated field. For each field, the notification provides a link to edit the field’s translations.
- alertFields (
Array
<Object
>) – field listmethod confirmChange(state, id, fields)
Updates the chatter area with the new state if its fields has changed
state
id
fields
method disableButtons()
Disable statusbar buttons and stat buttons so that they can’t be clicked anymore
method enableButtons()
Enable statusbar buttons and stat buttons so they can be clicked again
method getLocalState() → Object
returns the active tab pages for each notebook
Object
method setLocalState(state)
restore active tab pages for each notebook
- state (
Object
) – the result from a getLocalState callmethod on_attach_callback()
Call on_attach_callback
for each subview
method on_detach_callback()
Call on_detach_callback
for each subview
method getBoard() → Object
Returns a representation of the current dashboard
Object
module portal.chatter
class PortalChatter(parent, options)
parent
options
Widget PortalChatter
- Fetch message fron controller
- Display chatter: pager, total message, composer (according to access right)
- Provider API to filter displayed messages
method messageFetch(domain) → Deferred
Fetch the messages and the message count from the server for the current page and current domain.
- domain (
Array
)method preprocessMessages(messages) → Array
Update the messages format
- messages (
Array
<Object
>)Array
method round_to_half(value)
Round the given value with a precision of 0.5.
Examples: - 1.2 –> 1.0 - 1.7 –> 1.5 - 1.9 –> 2.0
- value (
Number
)namespace
class PortalChatter(parent, options)
parent
options
Widget PortalChatter
- Fetch message fron controller
- Display chatter: pager, total message, composer (according to access right)
- Provider API to filter displayed messages
method messageFetch(domain) → Deferred
Fetch the messages and the message count from the server for the current page and current domain.
- domain (
Array
)method preprocessMessages(messages) → Array
Update the messages format
- messages (
Array
<Object
>)Array
method round_to_half(value)
Round the given value with a precision of 0.5.
Examples: - 1.2 –> 1.0 - 1.7 –> 1.5 - 1.9 –> 2.0
- value (
Number
)module im_livechat.im_livechat
namespace
module website_sale.editor
namespace
module web.local_storage
object localStorage instance of RamStorage
module web.translation
function _t(source) → String
Eager translation function, performs translation immediately at call
site. Beware using this outside of method bodies (before the
translation database is loaded), you probably want _lt()
instead.
- source (
String
) – string to translateString
function _lt(s) → Object
Lazy translation function, only performs the translation when actually printed (e.g. inserted into a template)
Useful when defining translatable strings in code evaluated before the translation database is loaded, as class attributes or at the top-level of an OpenERP Web module
- s (
String
) – string to translateObject
namespace
function _t(source) → String
Eager translation function, performs translation immediately at call
site. Beware using this outside of method bodies (before the
translation database is loaded), you probably want _lt()
instead.
- source (
String
) – string to translateString
function _lt(s) → Object
Lazy translation function, only performs the translation when actually printed (e.g. inserted into a template)
Useful when defining translatable strings in code evaluated before the translation database is loaded, as class attributes or at the top-level of an OpenERP Web module
- s (
String
) – string to translateObject
module web_kanban_gauge.widget
class GaugeWidget()
options
- max_value: maximum value of the gauge [default: 100]
- max_field: get the max_value from the field that must be present in the view; takes over max_value
- gauge_value_field: if set, the value displayed below the gauge is taken from this field instead of the base field used for the gauge. This allows to display a number different from the gauge.
- label: lable of the gauge, displayed below the gauge value
- label_field: get the label from the field that must be present in the view; takes over label
- title: title of the gauge, displayed on top of the gauge
- style: custom style
class GaugeWidget()
options
- max_value: maximum value of the gauge [default: 100]
- max_field: get the max_value from the field that must be present in the view; takes over max_value
- gauge_value_field: if set, the value displayed below the gauge is taken from this field instead of the base field used for the gauge. This allows to display a number different from the gauge.
- label: lable of the gauge, displayed below the gauge value
- label_field: get the label from the field that must be present in the view; takes over label
- title: title of the gauge, displayed on top of the gauge
- style: custom style
module website.contentMenu
namespace
module point_of_sale.BaseWidget
class PosBaseWidget(parent, options)
parent
options
module web.ajax
namespace ajax
namespace loadXML
Loads an XML file according to the given URL and adds its associated qweb templates to the given qweb engine. The function can also be used to get the deferred which indicates when all the calls to the function are finished.
Note: “all the calls” = the calls that happened before the current no-args one + the calls that will happen after but when the previous ones are not finished yet.
function loadLibs(libs) → Deferred
Loads the given js and css libraries. Note that the ajax loadJS and loadCSS methods don’t do anything if the given file is already loaded.
- libs (
LoadLibsLibs
)class LoadLibsLibs()
attribute cssLibs Array<string>
- A list of css files, to be loaded in
- parallel
function get_file(options) → boolean
Cooperative file download implementation, for ajaxy APIs.
Requires that the server side implements an httprequest correctly
setting the fileToken
cookie to the value provided as the token
parameter. The cookie must be set on the /
path and must not be
httpOnly
.
It would probably also be a good idea for the response to use a
Content-Disposition: attachment
header, especially if the MIME is a
“known” type (e.g. text/plain, or for some browsers application/json
- options (
GetFileOptions
)boolean
class GetFileOptions()
attribute url String
used to dynamically create a form
attribute data Object
data to add to the form submission. If can be used without a form, in which case a form is created from scratch. Otherwise, added to form data
attribute form HTMLFormElement
the form to submit in order to fetch the file
attribute success Function
callback in case of download success
attribute error Function
callback in case of request error, provided with the error body
attribute complete Function
called after both success
and error
callbacks have executed
function get_file(options) → boolean
Cooperative file download implementation, for ajaxy APIs.
Requires that the server side implements an httprequest correctly
setting the fileToken
cookie to the value provided as the token
parameter. The cookie must be set on the /
path and must not be
httpOnly
.
It would probably also be a good idea for the response to use a
Content-Disposition: attachment
header, especially if the MIME is a
“known” type (e.g. text/plain, or for some browsers application/json
- options (
GetFileOptions
)boolean
class GetFileOptions()
attribute url String
used to dynamically create a form
attribute data Object
data to add to the form submission. If can be used without a form, in which case a form is created from scratch. Otherwise, added to form data
attribute form HTMLFormElement
the form to submit in order to fetch the file
attribute success Function
callback in case of download success
attribute error Function
callback in case of request error, provided with the error body
attribute complete Function
called after both success
and error
callbacks have executed
function loadLibs(libs) → Deferred
Loads the given js and css libraries. Note that the ajax loadJS and loadCSS methods don’t do anything if the given file is already loaded.
- libs (
LoadLibsLibs
)class LoadLibsLibs()
attribute cssLibs Array<string>
- A list of css files, to be loaded in
- parallel
namespace loadXML
Loads an XML file according to the given URL and adds its associated qweb templates to the given qweb engine. The function can also be used to get the deferred which indicates when all the calls to the function are finished.
Note: “all the calls” = the calls that happened before the current no-args one + the calls that will happen after but when the previous ones are not finished yet.
module web.ModelFieldSelector
class ModelFieldSelector(parent, model, chain[, options])
parent
model (string
) – the model name (e.g. “res.partner”)
chain (Array
<string
>) – list of the initial field chain parts
options (ModelFieldSelectorOptions
) – some key-value options
The ModelFieldSelector widget can be used to display/select a particular field chain from a given model.
method getSelectedField() → Object
Returns the field information selected by the field chain.
Object
method isValid() → boolean
Indicates if the field chain is valid. If the field chain has not been processed yet (the widget is not ready), this method will return undefined.
boolean
method setChain(chain) → Deferred
Saves a new field chain (array) and re-render.
- chain (
Array
<string
>) – the new field chainclass ModelFieldSelectorOptions()
some key-value options
attribute readonly boolean
true if should be readonly
attribute filters Object
some key-value options to filter the fetched fields
attribute filters.searchable boolean
true if only the searchable fields have to be used
attribute fields Object[]
- the list of fields info to use when no relation has
- been followed (null indicates the widget has to request the fields itself)
attribute followRelations boolean
true if can follow relation when building the chain
attribute debugMode boolean
true if the widget is in debug mode, false otherwise
namespace modelFieldsCache
Field Selector Cache - TODO Should be improved to use external cache ? - Stores fields per model used in field selector
function sortFields(fields, model) → Object[]
Allows to transform a mapping field name -> field info in an array of the field infos, sorted by field user name (“string” value). The field infos in the final array contain an additional key “name” with the field name.
fields (Object
) – the mapping field name -> field info
model (string
)
Array
<Object
>class ModelFieldSelector(parent, model, chain[, options])
parent
model (string
) – the model name (e.g. “res.partner”)
chain (Array
<string
>) – list of the initial field chain parts
options (ModelFieldSelectorOptions
) – some key-value options
The ModelFieldSelector widget can be used to display/select a particular field chain from a given model.
method getSelectedField() → Object
Returns the field information selected by the field chain.
Object
method isValid() → boolean
Indicates if the field chain is valid. If the field chain has not been processed yet (the widget is not ready), this method will return undefined.
boolean
method setChain(chain) → Deferred
Saves a new field chain (array) and re-render.
- chain (
Array
<string
>) – the new field chainclass ModelFieldSelectorOptions()
some key-value options
attribute readonly boolean
true if should be readonly
attribute filters Object
some key-value options to filter the fetched fields
attribute filters.searchable boolean
true if only the searchable fields have to be used
attribute fields Object[]
- the list of fields info to use when no relation has
- been followed (null indicates the widget has to request the fields itself)
attribute followRelations boolean
true if can follow relation when building the chain
attribute debugMode boolean
true if the widget is in debug mode, false otherwise
module web.FormView
class FormView(viewInfo)
- viewInfo
module web.SwitchCompanyMenu
class SwitchCompanyMenu()
module web_editor.widget
class Dialog(parent, options)
parent
options
Extend Dialog class to handle save/cancel of edition components.
class LinkDialog(parent, options, editable, linkInfo)
parent
options
editable
linkInfo
The Link Dialog allows to customize link content and style.
method bind_data()
Allows the URL input to propose existing website pages.
class MediaDialog(parent, options, $editable, media)
parent
options
$editable
media
MediaDialog widget. Lets users change a media, including uploading a new image, font awsome or video and can change a media into an other media
options: select_images: allow the selection of more of one image
class fontIconsDialog(parent, media)
parent
media
FontIconsDialog widget. Lets users change a font awesome, support all font awesome loaded in the css files.
method save()
Removes existing FontAwesome classes on the bound element, and sets all the new ones if necessary.
method getFont(classNames)
return the data font object (with base, parser and icons) or null
- classNames
method load_data()
Looks up the various FontAwesome classes on the bound element and sets the corresponding template/form elements to the right state. If multiple classes of the same category are present on an element (e.g. fa-lg and fa-3x) the last one occurring will be selected, which may not match the visual look of the element.
method get_fa_classes()
Serializes the dialog to an array of FontAwesome classes. Includes the base fa
.
class alt(parent, options, $editable, media)
parent
options
$editable
media
alt widget. Lets users change a alt & title on a media
namespace
class Dialog(parent, options)
parent
options
Extend Dialog class to handle save/cancel of edition components.
class alt(parent, options, $editable, media)
parent
options
$editable
media
alt widget. Lets users change a alt & title on a media
class MediaDialog(parent, options, $editable, media)
parent
options
$editable
media
MediaDialog widget. Lets users change a media, including uploading a new image, font awsome or video and can change a media into an other media
options: select_images: allow the selection of more of one image
class LinkDialog(parent, options, editable, linkInfo)
parent
options
editable
linkInfo
The Link Dialog allows to customize link content and style.
method bind_data()
Allows the URL input to propose existing website pages.
attribute fontIcons Array
list of font icons to load by editor. The icons are displayed in the media editor and identified like font and image (can be colored, spinned, resized with fa classes). To add font, push a new object {base, parser}
- base: class who appear on all fonts (eg: fa fa-refresh)
- parser: regular expression used to select all font in css style sheets
class fontIconsDialog(parent, media)
parent
media
FontIconsDialog widget. Lets users change a font awesome, support all font awesome loaded in the css files.
method save()
Removes existing FontAwesome classes on the bound element, and sets all the new ones if necessary.
method getFont(classNames)
return the data font object (with base, parser and icons) or null
- classNames
method load_data()
Looks up the various FontAwesome classes on the bound element and sets the corresponding template/form elements to the right state. If multiple classes of the same category are present on an element (e.g. fa-lg and fa-3x) the last one occurring will be selected, which may not match the visual look of the element.
method get_fa_classes()
Serializes the dialog to an array of FontAwesome classes. Includes the base fa
.
class ImageDialog(parent, media, options)
parent
media
options
ImageDialog widget. Let users change an image, including uploading a new image in flectra or selecting the image style (if supported by the caller).
class VideoDialog(parent, media)
parent
media
VideoDialog widget. Let users change a video, support all summernote video, and embed iframe.
class VideoDialog(parent, media)
parent
media
VideoDialog widget. Let users change a video, support all summernote video, and embed iframe.
attribute fontIcons Array
list of font icons to load by editor. The icons are displayed in the media editor and identified like font and image (can be colored, spinned, resized with fa classes). To add font, push a new object {base, parser}
- base: class who appear on all fonts (eg: fa fa-refresh)
- parser: regular expression used to select all font in css style sheets
class ImageDialog(parent, media, options)
parent
media
options
ImageDialog widget. Let users change an image, including uploading a new image in flectra or selecting the image style (if supported by the caller).
module mail.composer
namespace
module web.ViewManager
class ViewManager(parent, [dataset, ][views, ][flags, ]options)
parent
dataset (Object
)
views (Array
) – List of [view_id, view_type[, fields_view]]
flags (Object
) – various boolean describing UI state
options
method on_attach_callback()
Called each time the view manager is attached into the DOM
method on_detach_callback()
Called each time the view manager is detached from the DOM
method load_views() → Deferred
Loads all missing field_views of views in this.views and the search view.
method get_default_view() → Object
Returns the default view with the following fallbacks:
- use the default_view defined in the flags, if any
- use the first view in the view_order
Object
method render_switch_buttons()
Renders the switch buttons for multi- and mono-record views and adds listeners on them, but does not append them to the DOM Sets switch_buttons.$mono and switch_buttons.$multi to send to the ControlPanel
method render_view_control_elements()
Renders the control elements (buttons, sidebar, pager) of the current view. Fills this.active_view.control_elements dictionnary with the rendered elements and the adequate view switcher, to send to the ControlPanel. Warning: it should be called before calling do_show on the view as the sidebar is extended to listen on the load_record event triggered as soon as do_show is done (the sidebar should thus be instantiated before).
method setup_search_view()
Sets up the current viewmanager’s search view. Sets $searchview and $searchview_buttons in searchview_elements to send to the ControlPanel
method do_execute_action(action_data, env, on_closed)
Fetches and executes the action identified by action_data
.
action_data (DoExecuteActionActionData
) – the action descriptor data
env (DoExecuteActionEnv
)
on_closed (Function
) – callback to execute when dialog is closed or when the action does not generate any result (no new action)
class DoExecuteActionEnv()
attribute model string
the model of the record(s) triggering the action
attribute resIDs integer[]
the current ids in the environment where the action is triggered
attribute currentID integer
the id of the record triggering the action
attribute context Object
a context to pass to the action
class DoExecuteActionActionData()
the action descriptor data
attribute name String
the action name, used to uniquely identify the action to find and execute it
attribute special String
special action handlers, closes the dialog if set
attribute type String
the action type, if present, one of 'object'
, 'action'
or 'workflow'
attribute context Object
additional action context, to add to the current context
attribute effect string
- if given, a visual effect (a
- rainbowman by default) will be displayed when the action is complete, with the string (evaluated) given as options.
module web.QWeb
function QWeb(debug, default_dict[, enableTranslation])
debug (boolean
)
default_dict (Object
)
enableTranslation=true (boolean
) – if true (this is the default),
the rendering will translate all strings that are not marked with
t-translation=off. This is useful for the kanban view, which uses a
template which is already translated by the server
module web_editor.rte
namespace
module web.Context
class Context()
method set_eval_context(evalContext) → Context
Set the evaluation context to be used when we actually eval.
- evalContext (
Object
)Widgets
Exported in
web.Widget
, the base class for all visual components. It corresponds to an MVC view, and provides a number of service to simplify handling of a section of a page:
- Handles parent/child relationships between widgets
- Provides extensive lifecycle management with safety features (e.g. automatically destroying children widgets during the destruction of a parent)
- Automatic rendering with qweb
- Backbone-compatible shortcuts
DOM Root
A Widget()
is responsible for a section of the page
materialized by the DOM root of the widget.
A widget’s DOM root is available via two attributes:
Widget.el
raw DOM element set as root to the widget
Widget.$el
jQuery wrapper around el
There are two main ways to define and generate this DOM root:
Widget.template
Should be set to the name of a QWeb template. If set, the template will be rendered after the widget has been initialized but before it has been started. The root element generated by the template will be set as the DOM root of the widget.
Widget.tagName
Used if the widget has no template defined. Defaults to div
,
will be used as the tag name to create the DOM element to set as
the widget’s DOM root. It is possible to further customize this
generated DOM root with the following attributes:
Widget.Widget.id
Used to generate an id
attribute on the generated DOM
root.
Widget.className
Used to generate a class
attribute on the generated DOM root.
Widget.attributes
Mapping (object literal) of attribute names to attribute values. Each of these k:v pairs will be set as a DOM attribute on the generated DOM root.
None of these is used in case a template is specified on the widget.
The DOM root can also be defined programmatically by overridding
Widget.renderElement()
Renders the widget’s DOM root and sets it. The default
implementation will render a set template or generate an element
as described above, and will call
setElement()
on the result.
Any override to renderElement()
which
does not call its _super
must call
setElement()
with whatever it
generated or the widget’s behavior is undefined.
Note
The default renderElement()
can
be called repeatedly, it will replace the previous DOM root
(using replaceWith
). However, this requires that the
widget correctly sets and unsets its events (and children
widgets). Generally, renderElement()
should
not be called repeatedly unless the widget advertizes this feature.
Using a widget
A widget’s lifecycle has 3 main phases:
creation and initialization of the widget instance
Widget.init(parent)
initialization method of widgets, synchronous, can be overridden to take more parameters from the widget’s creator/parent
Arguments- parent (
Widget()
) – the new widget’s parent, used to handle automatic destruction and event propagation. Can benull
for the widget to have no parent.
- parent (
DOM injection and startup, this is done by calling one of:
Widget.appendTo(element)
Renders the widget and inserts it as the last child of the target, uses .appendTo()
Widget.prependTo(element)
Renders the widget and inserts it as the first child of the target, uses .prependTo()
Widget.insertAfter(element)
Renders the widget and inserts it as the preceding sibling of the target, uses .insertAfter()
Widget.insertBefore(element)
Renders the widget and inserts it as the following sibling of the target, uses .insertBefore()
All of these methods accept whatever the corresponding jQuery method accepts (CSS selectors, DOM nodes or jQuery objects). They all return a deferred and are charged with three tasks:
- rendering the widget’s root element via
renderElement()
- inserting the widget’s root element in the DOM using whichever jQuery method they match
starting the widget, and returning the result of starting it
Widget.start()
asynchronous startup of the widget once it’s been injected in the DOM, generally used to perform asynchronous RPC calls to fetch whatever remote data is necessary for the widget to do its work.
Must return a deferred to indicate when its work is done.
A widget is not guaranteed to work correctly until its
start()
method has finished executing. The widget’s parent/creator must wait for a widget to be fully started before interacting with itReturnsdeferred object
- rendering the widget’s root element via
widget destruction and cleanup
Widget.destroy()
destroys the widget’s children, unbinds its events and removes its root from the DOM. Automatically called when the widget’s parent is destroyed, must be called explicitly if the widget has no parents or if it is removed but its parent remains.
A widget being destroyed is automatically unlinked from its parent.
Related to widget destruction is an important utility method:
Widget.alive(deferred[, reject=false])
A significant issue with RPC and destruction is that an RPC call may take a long time to execute and return while a widget is being destroyed or after it has been destroyed, trying to execute its operations on a widget in a broken/invalid state.
This is a frequent source of errors or strange behaviors.
alive()
can be used to wrap an RPC call,
ensuring that whatever operations should be executed when the call ends
are only executed if the widget is still alive:
this.alive(this.model.query().all()).then(function (records) {
// would break if executed after the widget is destroyed, wrapping
// rpc in alive() prevents execution
_.each(records, function (record) {
self.$el.append(self.format(record));
});
});
Widget.isDestroyed()
true
if the widget is being or has been destroyed, false
otherwiseAccessing DOM content
- Because a widget is only responsible for the content below its DOM root, there
- is a shortcut for selecting sub-sections of a widget’s DOM:
Widget.$(selector)
Applies the CSS selector specified as parameter to the widget’s DOM root:
this.$(selector);
is functionally identical to:
this.$el.find(selector);
- selector (
String
) – CSS selector
Note
this helper method is similar to Backbone.View.$
Resetting the DOM root
Widget.setElement(element)
Re-sets the widget’s DOM root to the provided element, also handles re-setting the various aliases of the DOM root as well as unsetting and re-setting delegated events.
- element (
Element
) – a DOM element or jQuery object to set as the widget’s DOM root
Note
should be mostly compatible with Backbone’s setElement
DOM events handling
A widget will generally need to respond to user action within its section of the page. This entails binding events to DOM elements.
To this end, Widget()
provides a shortcut:
Widget.events
Events are a mapping of an event selector (an event name and an optional
CSS selector separated by a space) to a callback. The callback can
be the name of a widget’s method or a function object. In either case, the
this
will be set to the widget:
events: {
'click p.oe_some_class a': 'some_method',
'change input': function (e) {
e.stopPropagation();
}
},
The selector is used for jQuery’s event delegation, the callback will only be triggered for descendants of the DOM root matching the selector1. If the selector is left out (only an event name is specified), the event will be set directly on the widget’s DOM root.
Widget.delegateEvents()
This method is in charge of binding events
to the
DOM. It is automatically called after setting the widget’s DOM root.
It can be overridden to set up more complex events than the
events
map allows, but the parent should always be
called (or events
won’t be handled correctly).
Widget.undelegateEvents()
This method is in charge of unbinding events
from
the DOM root when the widget is destroyed or the DOM root is reset, in
order to avoid leaving “phantom” events.
It should be overridden to un-set any event set in an override of
delegateEvents()
.
Note
this behavior should be compatible with Backbone’s delegateEvents, apart from not accepting any argument.
Subclassing Widget
Widget()
is subclassed in the standard manner (via the
extend()
method), and provides a number of
abstract properties and concrete methods (which you may or may not want to
override). Creating a subclass looks like this:
var MyWidget = Widget.extend({
// QWeb template to use when rendering the object
template: "MyQWebTemplate",
events: {
// events binding example
'click .my-button': 'handle_click',
},
init: function(parent) {
this._super(parent);
// insert code to execute before rendering, for object
// initialization
},
start: function() {
var sup = this._super();
// post-rendering initialization code, at this point
// allows multiplexing deferred objects
return $.when(
// propagate asynchronous signal from parent class
sup,
// return own's asynchronous signal
this.rpc(/* … */))
}
});
The new class can then be used in the following manner:
// Create the instance
var my_widget = new MyWidget(this);
// Render and insert into DOM
my_widget.appendTo(".some-div");
After these two lines have executed (and any promise returned by
appendTo()
has been resolved if needed), the widget is
ready to be used.
Note
the insertion methods will start the widget themselves, and will
return the result of start()
.
If for some reason you do not want to call these methods, you will
have to first call render()
on the
widget, then insert it into your DOM and start it.
If the widget is not needed anymore (because it’s transient), simply terminate it:
my_widget.destroy();
will unbind all DOM events, remove the widget’s content from the DOM and destroy all widget data.
Development Guidelines
Identifiers (
id
attribute) should be avoided. In generic applications and modules,id
limits the re-usability of components and tends to make code more brittle. Most of the time, they can be replaced with nothing, classes or keeping a reference to a DOM node or jQuery element.If an
id
is absolutely necessary (because a third-party library requires one), the id should be partially generated using_.uniqueId()
e.g.:this.id = _.uniqueId('my-widget-')
- Avoid predictable/common CSS class names. Class names such as “content” or “navigation” might match the desired meaning/semantics, but it is likely an other developer will have the same need, creating a naming conflict and unintended behavior. Generic class names should be prefixed with e.g. the name of the component they belong to (creating “informal” namespaces, much as in C or Objective-C).
- Global selectors should be avoided. Because a component may be used several
times in a single page (an example in Flectra is dashboards), queries should be
restricted to a given component’s scope. Unfiltered selections such as
$(selector)
ordocument.querySelectorAll(selector)
will generally lead to unintended or incorrect behavior. Flectra Web’sWidget()
has an attribute providing its DOM root ($el
), and a shortcut to select nodes directly ($()
). - More generally, never assume your components own or controls anything beyond
its own personal
$el
- html templating/rendering should use QWeb unless absolutely trivial.
- All interactive components (components displaying information to the screen
or intercepting DOM events) must inherit from
Widget()
and correctly implement and use its API and life cycle.
RPC
To display and interact with data, calls to the Flectra server are necessary. This is performed using RPC <Remote Procedure Call>.
Flectra Web provides two primary APIs to handle this: a low-level JSON-RPC based API communicating with the Python section of Flectra Web (and of your module, if you have a Python part) and a high-level API above that allowing your code to talk directly to high-level Flectra models.
All networking APIs are asynchronous. As a result, all of them will return Deferred objects (whether they resolve those with values or not). Understanding how those work before before moving on is probably necessary.
High-level API: calling into Flectra models
Access to Flectra object methods (made available through XML-RPC from the server)
is done via Model()
. It maps onto the Flectra server objects via two primary
methods, call()
(exported in web.Model
) and query()
(exported in web.DataModel
, only available in the backend client).
call()
is a direct mapping to the corresponding method of
the Flectra server object. Its usage is similar to that of the Flectra Model API,
with three differences:
- The interface is asynchronous, so instead of returning results directly RPC method calls will return Deferred instances, which will themselves resolve to the result of the matching RPC call.
- Because ECMAScript 3/Javascript 1.5 doesnt feature any equivalent to
__getattr__
ormethod_missing
, there needs to be an explicit method to dispatch RPC methods. No notion of pooler, the model proxy is instantiated where needed, not fetched from an other (somewhat global) object:
var Users = new Model('res.users'); Users.call('change_password', ['oldpassword', 'newpassword'], {context: some_context}).then(function (result) { // do something with change_password result });
query()
is a shortcut for a builder-style
interface to searches (search
+ read
in Flectra RPC terms). It
returns a Query()
object which is immutable but
allows building new Query()
instances from the
first one, adding new properties or modifiying the parent object’s:
Users.query(['name', 'login', 'user_email', 'signature'])
.filter([['active', '=', true], ['company_id', '=', main_company]])
.limit(15)
.all().then(function (users) {
// do work with users records
});
The query is only actually performed when calling one of the query
serialization methods, all()
and
first()
. These methods will perform a new
RPC call every time they are called.
For that reason, it’s actually possible to keep “intermediate” queries around and use them differently/add new specifications on them.
class Model(name)
Model.Model.name
name of the model this object is bound to
Model.Model.call(method[, args][, kwargs])
Calls the method
method of the current model, with the
provided positional and keyword arguments.
- method (
String
) – method to call over rpc on thename
- args (
Array<>
) – positional arguments to pass to the method, optional - kwargs (
Object<>
) – keyword arguments to pass to the method, optional
Model.Model.query(fields)
- fields (
Array<String>
) – list of fields to fetch during the search
Query()
object
representing the search to performclass flectra.web.Query(fields)
The first set of methods is the “fetching” methods. They perform RPC queries using the internal data of the object they’re called on.
flectra.web.Query.flectra.web.Query.all()
Fetches the result of the current Query()
object’s
search.
flectra.web.Query.flectra.web.Query.first()
Fetches the first result of the current
Query()
, or null
if the current
Query()
does have any result.
flectra.web.Query.flectra.web.Query.count()
Fetches the number of records the current
Query()
would retrieve.
flectra.web.Query.flectra.web.Query.group_by(grouping...)
Fetches the groups for the query, using the first specified grouping parameter
- grouping (
Array<String>
) – Lists the levels of grouping asked of the server. Grouping can actually be an array or varargs.
The second set of methods is the “mutator” methods, they create a
new Query()
object with the relevant
(internal) attribute either augmented or replaced.
flectra.web.Query.flectra.web.Query.context(ctx)
Adds the provided ctx
to the query, on top of any existing
context
flectra.web.Query.flectra.web.Query.filter(domain)
Adds the provided domain to the query, this domain is
AND
-ed to the existing query domain.
flectra.web.Query.opeenrp.web.Query.offset(offset)
Sets the provided offset on the query. The new offset replaces the old one.
flectra.web.Query.flectra.web.Query.limit(limit)
Sets the provided limit on the query. The new limit replaces the old one.
flectra.web.Query.flectra.web.Query.order_by(fields…)
Overrides the model’s natural order with the provided field
specifications. Behaves much like Django’s QuerySet.order_by
:
- Takes 1..n field names, in order of most to least importance (the first field is the first sorting key). Fields are provided as strings.
- A field specifies an ascending order, unless it is prefixed
with the minus sign “
-
” in which case the field is used in the descending order
Divergences from Django’s sorting include a lack of random sort
(?
field) and the inability to “drill down” into relations
for sorting.
Aggregation (grouping)
Flectra has powerful grouping capacities, but they are kind-of strange
in that they’re recursive, and level n+1 relies on data provided
directly by the grouping at level n. As a result, while
flectra.models.Model.read_group()
works it’s not a very intuitive
API.
Flectra Web eschews direct calls to read_group()
in favor of calling a method of Query()
, much
in the way it is done in SQLAlchemy
2:
some_query.group_by(['field1', 'field2']).then(function (groups) {
// do things with the fetched groups
});
This method is asynchronous when provided with 1..n fields (to group
on) as argument, but it can also be called without any field (empty
fields collection or nothing at all). In this case, instead of
returning a Deferred object it will return null
.
When grouping criterion come from a third-party and may or may not list fields (e.g. could be an empty list), this provides two ways to test the presence of actual subgroups (versus the need to perform a regular query for records):
A check on
group_by
’s result and two completely separate code paths:var groups; if (groups = some_query.group_by(gby)) { groups.then(function (gs) { // groups }); } // no groups
Or a more coherent code path using
when()
’s ability to coerce values into deferreds:$.when(some_query.group_by(gby)).then(function (groups) { if (!groups) { // No grouping } else { // grouping, even if there are no groups (groups // itself could be an empty array) } });
The result of a (successful) group_by()
is
an array of QueryGroup()
:
class flectra.web.QueryGroup()
flectra.web.QueryGroup.flectra.web.QueryGroup.get(key)
returns the group’s attribute key
. Known attributes are:
grouped_on
- which grouping field resulted from this group
value
grouped_on
’s value for this grouplength
- the number of records in the group
aggregates
- a {field: value} mapping of aggregations for the group
flectra.web.QueryGroup.flectra.web.QueryGroup.query([fields...])
equivalent to Model.query()
but pre-filtered to
only include the records within this group. Returns a
Query()
which can be further manipulated as
usual.
flectra.web.QueryGroup.flectra.web.QueryGroup.subgroups()
returns a deferred to an array of QueryGroup()
below this one
Low-level API: RPC calls to Python side
While the previous section is great for calling core OpenERP code (models code), it does not work if you want to call the Python side of Flectra Web.
For this, a lower-level API exists on on
Session()
objects (the class is exported in web.Session
, but
an instance isusually available through web.session
): the rpc
method.
This method simply takes an absolute path (the absolute URL of the JSON route to call) and a mapping of attributes to values (passed as keyword arguments to the Python method). This function fetches the return value of the Python methods, converted to JSON.
For instance, to call the resequence
of the
DataSet()
controller:
session.rpc('/web/dataset/resequence', {
model: some_model,
ids: array_of_ids,
offset: 42
}).then(function (result) {
// resequence didn't error out
}, function () {
// an error occured during during call
});
Web Client
Javascript module system overview
A new module system (inspired from requirejs) has now been deployed. It has many advantages over the Flectra version 8 system.
- loading order: dependencies are guaranteed to be loaded first, even if files are not loaded in the correct order in the bundle files.
- easier to split a file into smaller logical units.
- no global variables: easier to reason.
- it is possible to examine every dependencies and dependants. This makes refactoring much simpler, and less risky.
It has also some disadvantages:
- files are required to use the module system if they want to interact with flectra, since the various objects are only available in the module system, and not in global variables
- circular dependencies are not supported. It makes sense, but it means that one needs to be careful.
This is obviously a very large change and will require everyone to adopt new habits. For example, the variable flectra does not exist anymore. The new way of doing things is to import explicitely the module you need, and declaring explicitely the objects you export. Here is a simple example:
flectra.define('addon_name.service', function (require) {
var utils = require('web.utils');
var Model = require('web.Model');
// do things with utils and Model
var something_useful = 15;
return {
something_useful: something_useful,
};
});
This snippet shows a module named addon_name.service
. It is defined
with the flectra.define
function. flectra.define
takes a name and a
function for arguments:
- The name is the concatenation of the name of the addon it is defined in and a name describing its purpose.
- The function is the place where the javascript module is actually
defined. It takes a function
require
as first argument, and returns something (or not, depending if it needs to export something). Therequire
function is used to get a handle on the dependencies. In this case, it gives a handle on two javascript modules from theweb
addon, namelyweb.utils
andweb.Model
.
The idea is that you define what you need to import (by using the
require
function) and declare what you export (by returning
something). The web client will then make sure that your code is loaded
properly.
Modules are contained in a file, but a file can define several modules (however, it is better to keep them in separate files).
Each module can return a deferred. In that case, the module is marked as loaded only when the deferred is resolved, and its value is equal to the resolved value. The module can be rejected (unloaded). This will be logged in the console as info.
Missing dependencies
: These modules do not appear in the page. It is possible that the JavaScript file is not in the page or that the module name is wrongFailed modules
: A javascript error is detectedRejected modules
: The module returns a rejected deferred. It (and its dependent modules) is not loaded.Rejected linked modules
: Modules who depend on a rejected moduleNon loaded modules
: Modules who depend on a missing or a failed module
Web client structure
The web client files have been refactored into smaller and simpler files. Here is a description of the current file structure:
the
framework/
folder contains all basic low level modules. The modules here are supposed to be generic. Some of them are:web.ajax
implements rpc callsweb.core
is a generic modules. It exports various useful objects and functions, such asqweb
,_t
or the main bus.web.Widget
contains the widget classweb.Model
is an abstraction overweb.ajax
to make calls to the server model methodsweb.session
is the formerflectra.session
web.utils
for useful code snippetsweb.time
for every time-related generic functions
- the
views/
folder contains all view definitions widgets/
is for standalone widgets
The js/
folder also contains some important files:
action_manager.js
is the ActionManager classboot.js
is the file actually implementing the module systemmenu.js
is the definition of the top menuweb_client.js
is for the root widget WebClientview_manager.js
contains the ViewManager
The two other files are tour.js
for the tours and compatibility.js
.
The latter file is a compatibility layer bridging the old system to the
new module system. This is where every module names are exported to the
global variable flectra
. In theory, our addons should work without
ever using the variable flectra
, and the compatibility module can be
disabled safely.
Javascript conventions
Here are some basic conventions for the javascript code:
- declare all your dependencies at the top of the module. Also, they should be sorted alphabetically by module name. This makes it easier to understand your module.
- declare all exports at the end.
- add the
use strict
statement at the beginning of every module - always name your module properly:
addon_name.description
. - use capital letters for classes (for example,
ActionManager
is defined in the moduleweb.ActionManager
), and lowercase for everything else (for example,ajax
is defined inweb.ajax
). - declare one module per file
Testing in Flectra Web Client
Javascript Unit Testing
Flectra Web includes means to unit-test both the core code of Flectra Web and your own javascript modules. On the javascript side, unit-testing is based on QUnit with a number of helpers and extensions for better integration with Flectra.
To see what the runner looks like, find (or start) an Flectra server
with the web client enabled, and navigate to /web/tests
This will show the runner selector, which lists all modules with javascript
unit tests, and allows starting any of them (or all javascript tests in all
modules at once).
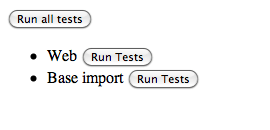
Clicking any runner button will launch the corresponding tests in the bundled QUnit runner:
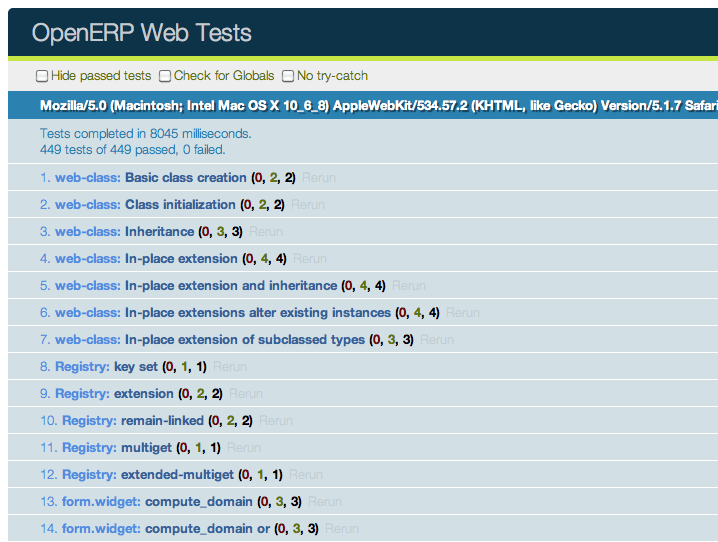
Writing a test case
The first step is to list the test file(s). This is done through the
test
key of the Flectra manifest, by adding javascript files to it:
{
'name': "Demonstration of web/javascript tests",
'category': 'Hidden',
'depends': ['web'],
'test': ['static/tests/demo.js'],
}
and to create the corresponding test file(s)
Note
Test files which do not exist will be ignored, if all test files of a module are ignored (can not be found), the test runner will consider that the module has no javascript tests.
After that, refreshing the runner selector will display the new module and allow running all of its (0 so far) tests:
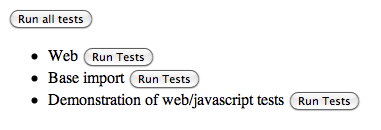
The next step is to create a test case:
flectra.testing.section('basic section', function (test) {
test('my first test', function () {
ok(false, "this test has run");
});
});
All testing helpers and structures live in the flectra.testing
module. Flectra tests live in a section()
,
which is itself part of a module. The first argument to a section is
the name of the section, the second one is the section body.
test
, provided by the
section()
to the callback, is used to
register a given test case which will be run whenever the test runner
actually does its job. Flectra Web test case use standard QUnit
assertions within them.
Launching the test runner at this point will run the test and display the corresponding assertion message, with red colors indicating the test failed:
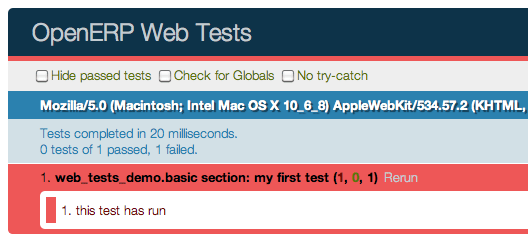
Fixing the test (by replacing false
to true
in the assertion)
will make it pass:
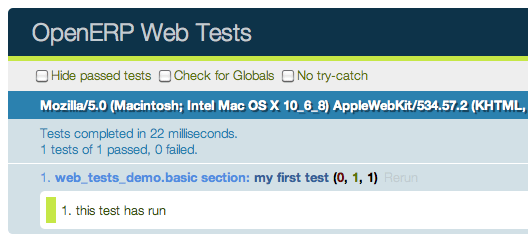
Assertions
As noted above, Flectra Web’s tests use qunit assertions. They are available globally (so they can just be called without references to anything). The following list is available:
ok(state[, message])
checks that state
is truthy (in the javascript sense)
strictEqual(actual, expected[, message])
checks that the actual (produced by a method being tested) and
expected values are identical (roughly equivalent to ok(actual
=== expected, message)
)
notStrictEqual(actual, expected[, message])
checks that the actual and expected values are not identical
(roughly equivalent to ok(actual !== expected, message)
)
deepEqual(actual, expected[, message])
deep comparison between actual and expected: recurse into containers (objects and arrays) to ensure that they have the same keys/number of elements, and the values match.
notDeepEqual(actual, expected[, message])
inverse operation to deepEqual()
throws(block[, expected][, message])
checks that, when called, the block
throws an
error. Optionally validates that error against expected
.
- block (
Function
) – - expected (
Error | RegExp
) – if a regexp, checks that the thrown error’s message matches the regular expression. If an error type, checks that the thrown error is of that type.
equal(actual, expected[, message])
checks that actual
and expected
are loosely equal, using
the ==
operator and its coercion rules.
notEqual(actual, expected[, message])
inverse operation to equal()
Getting an Flectra instance
The Flectra instance is the base through which most Flectra Web modules behaviors (functions, objects, …) are accessed. As a result, the test framework automatically builds one, and loads the module being tested and all of its dependencies inside it. This new instance is provided as the first positional parameter to your test cases. Let’s observe by adding javascript code (not test code) to the test module:
{
'name': "Demonstration of web/javascript tests",
'category': 'Hidden',
'depends': ['web'],
'js': ['static/src/js/demo.js'],
'test': ['static/tests/demo.js'],
}
// src/js/demo.js
flectra.web_tests_demo = function (instance) {
instance.web_tests_demo = {
value_true: true,
SomeType: instance.web.Class.extend({
init: function (value) {
this.value = value;
}
})
};
};
and then adding a new test case, which simply checks that the
instance
contains all the expected stuff we created in the
module:
// test/demo.js
test('module content', function (instance) {
ok(instance.web_tests_demo.value_true, "should have a true value");
var type_instance = new instance.web_tests_demo.SomeType(42);
strictEqual(type_instance.value, 42, "should have provided value");
});
DOM Scratchpad
As in the wider client, arbitrarily accessing document content is
strongly discouraged during tests. But DOM access is still needed to
e.g. fully initialize widgets
before
testing them.
Thus, a test case gets a DOM scratchpad as its second positional parameter, in a jQuery instance. That scratchpad is fully cleaned up before each test, and as long as it doesn’t do anything outside the scratchpad your code can do whatever it wants:
// test/demo.js
test('DOM content', function (instance, $scratchpad) {
$scratchpad.html('<div><span class="foo bar">ok</span></div>');
ok($scratchpad.find('span').hasClass('foo'),
"should have provided class");
});
test('clean scratchpad', function (instance, $scratchpad) {
ok(!$scratchpad.children().length, "should have no content");
ok(!$scratchpad.text(), "should have no text");
});
Note
The top-level element of the scratchpad is not cleaned up, test
cases can add text or DOM children but shoud not alter
$scratchpad
itself.
Loading templates
To avoid the corresponding processing costs, by default templates are
not loaded into QWeb. If you need to render e.g. widgets making use of
QWeb templates, you can request their loading through the
templates
option to the test case
function
.
This will automatically load all relevant templates in the instance’s qweb before running the test case:
{
'name': "Demonstration of web/javascript tests",
'category': 'Hidden',
'depends': ['web'],
'js': ['static/src/js/demo.js'],
'test': ['static/tests/demo.js'],
'qweb': ['static/src/xml/demo.xml'],
}
<!-- src/xml/demo.xml -->
<templates id="template" xml:space="preserve">
<t t-name="DemoTemplate">
<t t-foreach="5" t-as="value">
<p><t t-esc="value"/></p>
</t>
</t>
</templates>
// test/demo.js
test('templates', {templates: true}, function (instance) {
var s = instance.web.qweb.render('DemoTemplate');
var texts = $(s).find('p').map(function () {
return $(this).text();
}).get();
deepEqual(texts, ['0', '1', '2', '3', '4']);
});
Asynchronous cases
The test case examples so far are all synchronous, they execute from the first to the last line and once the last line has executed the test is done. But the web client is full of asynchronous code, and thus test cases need to be async-aware.
This is done by returning a deferred
from the
case callback:
// test/demo.js
test('asynchronous', {
asserts: 1
}, function () {
var d = $.Deferred();
setTimeout(function () {
ok(true);
d.resolve();
}, 100);
return d;
});
This example also uses the options parameter
to specify the number of assertions the case should expect, if less or
more assertions are specified the case will count as failed.
Asynchronous test cases must specify the number of assertions they will run. This allows more easily catching situations where e.g. the test architecture was not warned about asynchronous operations.
Note
Asynchronous test cases also have a 2 seconds timeout: if the test does not finish within 2 seconds, it will be considered failed. This pretty much always means the test will not resolve. This timeout only applies to the test itself, not to the setup and teardown processes.
Note
If the returned deferred is rejected, the test will be failed
unless fail_on_rejection
is set to
false
.
RPC
An important subset of asynchronous test cases is test cases which need to perform (and chain, to an extent) RPC calls.
Note
Because they are a subset of asynchronous cases, RPC cases must
also provide a valid assertions count
.
To enable mock RPC, set the rpc option
to
mock
. This will add a third parameter to the test case callback:
mock(rpc_spec, handler)
Can be used in two different ways depending on the shape of the first parameter:
If it matches the pattern
model:method
(if it contains a colon, essentially) the call will set up the mocking of an RPC call straight to the Flectra server (through XMLRPC) as performed via e.g.flectra.web.Model.call()
.In that case,
handler
should be a function taking two argumentsargs
andkwargs
, matching the corresponding arguments on the server side and should simply return the value as if it were returned by the Python XMLRPC handler:test('XML-RPC', {rpc: 'mock', asserts: 3}, function (instance, $s, mock) { // set up mocking mock('people.famous:name_search', function (args, kwargs) { strictEqual(kwargs.name, 'bob'); return [ [1, "Microsoft Bob"], [2, "Bob the Builder"], [3, "Silent Bob"] ]; }); // actual test code return new instance.web.Model('people.famous') .call('name_search', {name: 'bob'}).then(function (result) { strictEqual(result.length, 3, "shoud return 3 people"); strictEqual(result[0][1], "Microsoft Bob", "the most famous bob should be Microsoft Bob"); }); });
Otherwise, if it matches an absolute path (e.g.
/a/b/c
) it will mock a JSON-RPC call to a web client controller, such as/web/webclient/translations
. In that case, the handler takes a singleparams
argument holding all of the parameters provided over JSON-RPC.As previously, the handler should simply return the result value as if returned by the original JSON-RPC handler:
test('JSON-RPC', {rpc: 'mock', asserts: 3, templates: true}, function (instance, $s, mock) { var fetched_dbs = false, fetched_langs = false; mock('/web/database/get_list', function () { fetched_dbs = true; return ['foo', 'bar', 'baz']; }); mock('/web/session/get_lang_list', function () { fetched_langs = true; return [['vo_IS', 'Hopelandic / Vonlenska']]; }); // widget needs that or it blows up instance.webclient = {toggle_bars: flectra.testing.noop}; var dbm = new instance.web.DatabaseManager({}); return dbm.appendTo($s).then(function () { ok(fetched_dbs, "should have fetched databases"); ok(fetched_langs, "should have fetched languages"); deepEqual(dbm.db_list, ['foo', 'bar', 'baz']); }); });
Note
Mock handlers can contain assertions, these assertions should be part of the assertions count (and if multiple calls are made to a handler containing assertions, it multiplies the effective number of assertions).
Testing API
flectra.testing.section(name, [options, ]body)
A test section, serves as shared namespace for related tests (for
constants or values to only set up once). The body
function
should contain the tests themselves.
Note that the order in which tests are run is essentially undefined, do not rely on it.
- name (
String
) – - options (
TestOptions
) – - body (Function<
case()
, void>) –
flectra.testing.case(name, [options, ]callback)
Registers a test case callback in the test runner, the callback will only be run once the runner is started (or maybe not at all, if the test is filtered out).
- name (
String
) – - options (
TestOptions
) – - callback (
Function<instance, $, Function<String, Function, void>>
) –
class TestOptions()
the various options which can be passed to
section()
or
case()
. Except for
setup
and
teardown
, an option on
case()
will overwrite the corresponding
option on section()
so
e.g. rpc
can be set for a
section()
and then differently set for
some case()
of that
section()
TestOptions.TestOptions.asserts
An integer, the number of assertions which should run during a normal execution of the test. Mandatory for asynchronous tests.
TestOptions.TestOptions.setup
Test case setup, run right before each test case. A section’s
setup()
is run before the case’s own, if
both are specified.
TestOptions.TestOptions.teardown
Test case teardown, a case’s teardown()
is run before the corresponding section if both are present.
TestOptions.TestOptions.fail_on_rejection
If the test is asynchronous and its resulting promise is
rejected, fail the test. Defaults to true
, set to
false
to not fail the test in case of rejection:
// test/demo.js
test('unfail rejection', {
asserts: 1,
fail_on_rejection: false
}, function () {
var d = $.Deferred();
setTimeout(function () {
ok(true);
d.reject();
}, 100);
return d;
});
TestOptions.TestOptions.rpc
RPC method to use during tests, one of "mock"
or
"rpc"
. Any other value will disable RPC for the test (if
they were enabled by the suite for instance).
TestOptions.TestOptions.templates
Whether the current module (and its dependencies)’s templates
should be loaded into QWeb before starting the test. A
boolean, false
by default.
The test runner can also use two global configuration values set
directly on the window
object:
oe_all_dependencies
is anArray
of all modules with a web component, ordered by dependency (for a moduleA
with dependenciesA'
, any module ofA'
must come beforeA
in the array)
Running through Python
The web client includes the means to run these tests on the command-line (or in a CI system), but while actually running it is pretty simple the setup of the pre-requisite parts has some complexities.
Install PhantomJS. It is a headless browser which allows automating running and testing web pages. QUnitSuite uses it to actually run the qunit test suite.
The PhantomJS website provides pre-built binaries for some platforms, and your OS’s package management probably provides it as well.
If you’re building PhantomJS from source, I recommend preparing for some knitting time as it’s not exactly fast (it needs to compile both Qt and Webkit, both being pretty big projects).
Note
Because PhantomJS is webkit-based, it will not be able to test if Firefox, Opera or Internet Explorer can correctly run the test suite (and it is only an approximation for Safari and Chrome). It is therefore recommended to also run the test suites in actual browsers once in a while.
Install a new database with all relevant modules (all modules with a web component at least), then restart the server
Note
For some tests, a source database needs to be duplicated. This operation requires that there be no connection to the database being duplicated, but Flectra doesn’t currently break existing/outstanding connections, so restarting the server is the simplest way to ensure everything is in the right state.
Launch
oe run-tests -d $DATABASE -mweb
with the correct addons-path specified (and replacing$DATABASE
by the source database you created above)Note
If you leave out
-mweb
, the runner will attempt to run all the tests in all the modules, which may or may not work.
If everything went correctly, you should now see a list of tests with
(hopefully) ok
next to their names, closing with a report of the
number of tests run and the time it took:
test_empty_find (flectra.addons.web.tests.test_dataset.TestDataSetController) ... ok
test_ids_shortcut (flectra.addons.web.tests.test_dataset.TestDataSetController) ... ok
test_regular_find (flectra.addons.web.tests.test_dataset.TestDataSetController) ... ok
web.testing.stack: direct, value, success ... ok
web.testing.stack: direct, deferred, success ... ok
web.testing.stack: direct, value, error ... ok
web.testing.stack: direct, deferred, failure ... ok
web.testing.stack: successful setup ... ok
web.testing.stack: successful teardown ... ok
web.testing.stack: successful setup and teardown ... ok
[snip ~150 lines]
test_convert_complex_context (flectra.addons.web.tests.test_view.DomainsAndContextsTest) ... ok
test_convert_complex_domain (flectra.addons.web.tests.test_view.DomainsAndContextsTest) ... ok
test_convert_literal_context (flectra.addons.web.tests.test_view.DomainsAndContextsTest) ... ok
test_convert_literal_domain (flectra.addons.web.tests.test_view.DomainsAndContextsTest) ... ok
test_retrieve_nonliteral_context (flectra.addons.web.tests.test_view.DomainsAndContextsTest) ... ok
test_retrieve_nonliteral_domain (flectra.addons.web.tests.test_view.DomainsAndContextsTest) ... ok
----------------------------------------------------------------------
Ran 181 tests in 15.706s
OK
Congratulation, you have just performed a successful “offline” run of the OpenERP Web test suite.
Note
Note that this runs all the Python tests for the web
module,
but all the web tests for all of Flectra. This can be surprising.
sqlalchemy.orm.query.Query.group_by()
is not
terminal, it returns a query which can still be altered.